move web socket to unique place
and support Joe’s satellite node
This commit is contained in:
parent
950264ac13
commit
d507389953
@ -7,6 +7,7 @@ map web page for plotting "things" on.
|
||||
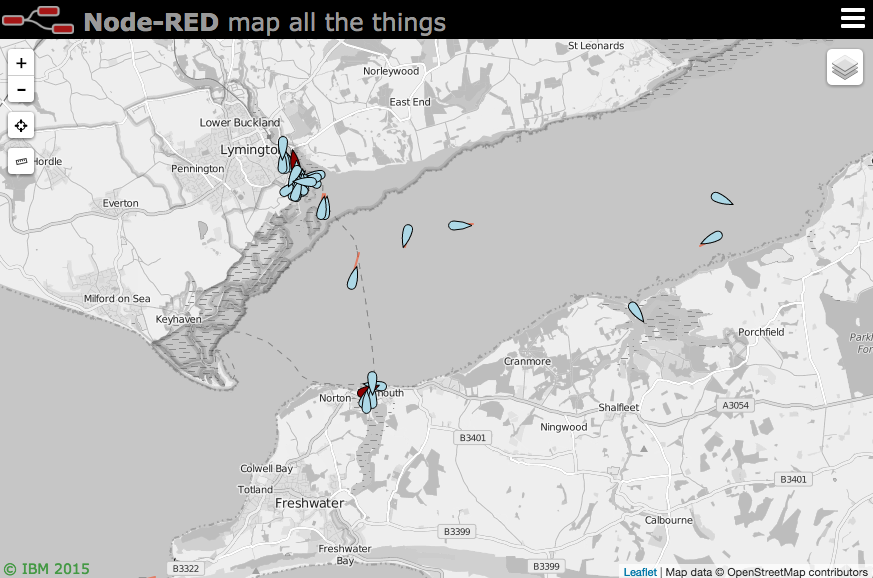
|
||||
|
||||
### Changes
|
||||
- v1.0.28 - Move websocket to specific path, and support satellite node
|
||||
- v1.0.26 - Add info on how to use with local WMS server
|
||||
- v1.0.24 - Add `.weblink` property to allow links out to other information.
|
||||
- v1.0.23 - Add msg.payload.command.heatmap to allow setting of heatmap config.
|
||||
|
@ -1,6 +1,6 @@
|
||||
{
|
||||
"name" : "node-red-contrib-web-worldmap",
|
||||
"version" : "1.0.26",
|
||||
"version" : "1.0.28",
|
||||
"description" : "A Node-RED node to provide a web page of a world map for plotting things on.",
|
||||
"dependencies" : {
|
||||
"express": "^4.15.0",
|
||||
|
@ -1,6 +1,6 @@
|
||||
<!DOCTYPE html>
|
||||
<!--
|
||||
Copyright 2015, 2016 IBM Corp.
|
||||
Copyright 2015, 2017 IBM Corp.
|
||||
|
||||
Licensed under the Apache License, Version 2.0 (the "License");
|
||||
you may not use this file except in compliance with the License.
|
||||
|
@ -1,5 +1,5 @@
|
||||
/**
|
||||
* Copyright 2015, 2016 IBM Corp.
|
||||
* Copyright 2015, 2017 IBM Corp.
|
||||
*
|
||||
* Licensed under the Apache License, Version 2.0 (the "License");
|
||||
* you may not use this file except in compliance with the License.
|
||||
@ -16,13 +16,17 @@
|
||||
|
||||
module.exports = function(RED) {
|
||||
"use strict";
|
||||
var path = require("path");
|
||||
var express = require("express");
|
||||
var io = require('socket.io');
|
||||
var socket;
|
||||
|
||||
var WorldMap = function(n) {
|
||||
RED.nodes.createNode(this,n);
|
||||
if (!socket) { socket = io.listen(RED.server); }
|
||||
if (!socket) {
|
||||
var fullPath = path.join(RED.settings.httpNodeRoot, 'worldmap', 'socket.io');
|
||||
socket = io.listen(RED.server, {path:fullPath});
|
||||
}
|
||||
this.lat = n.lat || "";
|
||||
this.lon = n.lon || "";
|
||||
this.zoom = n.zoom || "";
|
||||
@ -72,6 +76,7 @@ module.exports = function(RED) {
|
||||
}
|
||||
RED.nodes.registerType("worldmap",WorldMap);
|
||||
|
||||
|
||||
var WorldMapIn = function(n) {
|
||||
RED.nodes.createNode(this,n);
|
||||
if (!socket) { socket = io.listen(RED.server); }
|
||||
|
@ -124,7 +124,7 @@ var inIframe = false;
|
||||
var showUserMenu = true;
|
||||
|
||||
// Create the socket
|
||||
var ws = io();
|
||||
var ws = io({path:location.pathname + 'socket.io'});
|
||||
|
||||
ws.on('connect', function() {
|
||||
console.log("CONNECTED");
|
||||
@ -771,9 +771,18 @@ function setMarker(data) {
|
||||
}
|
||||
//console.log("handling",data.name);
|
||||
if (typeof data.coordinates == "object") { ll = new L.LatLng(data.coordinates[1],data.coordinates[0]); }
|
||||
else if (data.hasOwnProperty("position") && data.position.hasOwnProperty("lat") && data.position.hasOwnProperty("lon")) {
|
||||
data.lat = data.position.lat*1;
|
||||
data.lon = data.position.lon*1;
|
||||
data.alt = data.position.alt;
|
||||
if (parseFloat(data.position.alt) == data.position.alt) { data.alt = data.position.alt + " m"; }
|
||||
delete data.position;
|
||||
ll = new L.LatLng((data.lat*1), (data.lon*1));
|
||||
}
|
||||
else if (data.hasOwnProperty("lat") && data.hasOwnProperty("lon")) { ll = new L.LatLng((data.lat*1), (data.lon*1)); }
|
||||
else if (data.hasOwnProperty("latitude") && data.hasOwnProperty("longitude")) { ll = new L.LatLng((data.latitude*1), (data.longitude*1)); }
|
||||
else { console.log("No location:",data); return; }
|
||||
|
||||
// Adding new L.LatLng object (lli) when optional intensity value is defined. Only for use in heatmap layer
|
||||
if (typeof data.coordinates == "object") { lli = new L.LatLng(data.coordinates[2],data.coordinates[1],data.coordinates[0]); }
|
||||
else if (data.hasOwnProperty("lat") && data.hasOwnProperty("lon") && data.hasOwnProperty("intensity")) { lli = new L.LatLng((data.lat*1), (data.lon*1), (data.intensity*1)); }
|
||||
@ -985,8 +994,13 @@ function doCommand(cmd) {
|
||||
if (basemaps.hasOwnProperty(cmd.map.name)) { existsalready = true; }
|
||||
if (cmd.map.hasOwnProperty("wms")) { // special case for wms
|
||||
console.log("New WMS:",cmd.map.name);
|
||||
if (cmd.map.wms === "grey") {
|
||||
basemaps[cmd.map.name] = L.tileLayer.graywms(cmd.map.url, cmd.map.opt);
|
||||
}
|
||||
else {
|
||||
basemaps[cmd.map.name] = L.tileLayer.wms(cmd.map.url, cmd.map.opt);
|
||||
}
|
||||
}
|
||||
else {
|
||||
console.log("New Map:",cmd.map.name);
|
||||
basemaps[cmd.map.name] = L.tileLayer(cmd.map.url, cmd.map.opt);
|
||||
@ -1000,8 +1014,13 @@ function doCommand(cmd) {
|
||||
console.log("New overlay:",cmd.map.overlay);
|
||||
if (overlays.hasOwnProperty(cmd.map.overlay)) { existsalready = true; }
|
||||
if (cmd.map.hasOwnProperty("wms")) { // special case for wms
|
||||
if (cmd.map.wms === "grey") {
|
||||
overlays[cmd.map.name] = L.tileLayer.graywms(cmd.map.url, cmd.map.opt);
|
||||
}
|
||||
else {
|
||||
overlays[cmd.map.name] = L.tileLayer.wms(cmd.map.url, cmd.map.opt);
|
||||
}
|
||||
}
|
||||
else {
|
||||
overlays[cmd.map.overlay] = L.tileLayer(cmd.map.url, cmd.map.opt);
|
||||
}
|
||||
|
@ -1,5 +1,5 @@
|
||||
CACHE MANIFEST
|
||||
# date: Apr 19th 2017 - v1.0.26
|
||||
# date: May 2nd 2017 - v1.0.28
|
||||
|
||||
CACHE:
|
||||
index.html
|
||||
|
Loading…
Reference in New Issue
Block a user