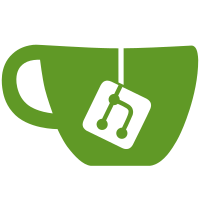
1. Pass an error to an active query if the client is ended while a query is in progress. 2. actually emit 'end' event on the client when the stream ends 3. do not emit an error from native bindings if lasterror is null
69 lines
1.6 KiB
JavaScript
69 lines
1.6 KiB
JavaScript
var helper = require(__dirname + "/../test-helper");
|
|
var Client = require(__dirname + "/../../lib/native");
|
|
|
|
test('query with non-text as first parameter throws error', function() {
|
|
var client = new Client(helper.config);
|
|
client.connect();
|
|
assert.emits(client, 'connect', function() {
|
|
client.end();
|
|
assert.emits(client, 'end', function() {
|
|
assert.throws(function() {
|
|
client.query({text:{fail: true}});
|
|
});
|
|
});
|
|
});
|
|
});
|
|
|
|
test('parameterized query with non-text as first parameter throws error', function() {
|
|
var client = new Client(helper.config);
|
|
client.connect();
|
|
assert.emits(client, 'connect', function() {
|
|
client.end();
|
|
assert.emits(client, 'end', function() {
|
|
assert.throws(function() {
|
|
client.query({
|
|
text: {fail: true},
|
|
values: [1, 2]
|
|
})
|
|
});
|
|
});
|
|
});
|
|
});
|
|
|
|
var connect = function(callback) {
|
|
var client = new Client(helper.config);
|
|
client.connect();
|
|
assert.emits(client, 'connect', function() {
|
|
callback(client);
|
|
})
|
|
}
|
|
|
|
test('parameterized query with non-array for second value', function() {
|
|
test('inline', function() {
|
|
connect(function(client) {
|
|
client.end();
|
|
assert.emits(client, 'end', function() {
|
|
assert.throws(function() {
|
|
client.query("SELECT *", "LKSDJF")
|
|
});
|
|
});
|
|
});
|
|
});
|
|
|
|
test('config', function() {
|
|
connect(function(client) {
|
|
client.end();
|
|
assert.emits(client, 'end', function() {
|
|
assert.throws(function() {
|
|
client.query({
|
|
text: "SELECT *",
|
|
values: "ALSDKFJ"
|
|
});
|
|
});
|
|
});
|
|
});
|
|
});
|
|
});
|
|
|
|
|