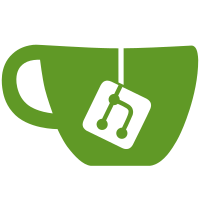
-v, --version print node's version --debug[=port] enable remote debugging via given TCP port --debug-brk[=port] as above, but break in node.js and wait for remote debugger to connect --cflags print pre-processor and compiler flags --v8-options print v8 command line options Documentation can be found at http://nodejs.org/api.html or with 'man node', as it's, um, used by node. Use instead. Also moved tree.node requires in tree.js
66 lines
2.1 KiB
JavaScript
66 lines
2.1 KiB
JavaScript
if (typeof(window) === 'undefined') { var tree = require(require('path').join(__dirname, '..', '..', 'less', 'tree')); }
|
|
|
|
tree.Ruleset = function Ruleset(selectors, rules) {
|
|
this.selectors = selectors;
|
|
this.rules = rules;
|
|
};
|
|
tree.Ruleset.prototype = {
|
|
variables: function () {
|
|
return this.rules.filter(function (r) {
|
|
if (r instanceof tree.Rule && r.variable === true) { return r }
|
|
});
|
|
},
|
|
toCSS: function (context, env) {
|
|
var css = [],
|
|
rules = [],
|
|
rulesets = [],
|
|
paths = [],
|
|
selector;
|
|
|
|
if (! this.root) {
|
|
if (context.length === 0) {
|
|
paths = this.selectors.map(function (s) { return [s] });
|
|
} else {
|
|
for (var s = 0; s < this.selectors.length; s++) {
|
|
for (var c = 0; c < context.length; c++) {
|
|
paths.push(context[c].concat([this.selectors[s]]));
|
|
}
|
|
}
|
|
}
|
|
}
|
|
env.frames.unshift(this);
|
|
|
|
for (var i = 0; i < this.rules.length; i++) {
|
|
if (this.rules[i] instanceof tree.Ruleset) {
|
|
rulesets.push(this.rules[i].toCSS(paths, env));
|
|
} else {
|
|
if (this.rules[i].toCSS) {
|
|
rules.push(this.rules[i].toCSS(env));
|
|
} else if (this.rules[i].value) {
|
|
rules.push(this.rules[i].value.toString());
|
|
}
|
|
}
|
|
}
|
|
|
|
rulesets = rulesets.join('');
|
|
|
|
if (this.root) {
|
|
css.push(rules.join('\n'));
|
|
} else {
|
|
if (rules.length > 0) {
|
|
selector = paths.map(function (p) {
|
|
return new(tree.Selector)(p).toCSS().trim();
|
|
}).join(paths.length > 3 ? ',\n' : ', ');
|
|
css.push(selector, " {\n " + rules.join('\n ') + "\n}\n");
|
|
}
|
|
}
|
|
css.push(rulesets);
|
|
env.frames.shift();
|
|
|
|
for (var p = 0; p < paths.length; p++) { paths[p].pop() }
|
|
|
|
return css.join('');
|
|
}
|
|
};
|
|
|