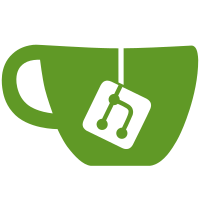
- Log system revamp: - Logs to stdout, disabled while testing - Use header `X-Request-Id`, or create a new `uuid` when no present, to identyfy log entries - Be able to set log level from env variable `LOG_LEVEL`, useful while testing: `LOG_LEVEL=info npm test`; even more human-readable: `LOG_LEVEL=info npm t | ./node_modules/.bin/pino-pretty` - Be able to reduce the footprint in the final log file depending on the environment - Use one logger for every service: Queries, Batch Queries (Jobs), and Data Ingestion (CopyTo/CopyFrom) - Stop using headers such as: `X-SQL-API-Log`, `X-SQL-API-Profiler`, and `X-SQL-API-Errors` as a way to log info. - Be able to tag requests with labels as an easier way to provide business metrics - Metro: Add log-collector utility (`metro`), it will be moved to its own repository. Attaching it here fro development purposes. Try it with the following command `LOG_LEVEL=info npm t | node metro` - Metro: Creates `metrics-collector.js` a stream to update Prometheus' counters and histograms and exposes them via Express' app (`:9145/metrics`). Use the ones defined in `grok_exporter` Announcements: - Profiler is always set. No need to check its existence anymore - Unify profiler usage for every endpoint Bug fixes: - Avoid hung requests while fetching user identifier
119 lines
2.7 KiB
JavaScript
119 lines
2.7 KiB
JavaScript
'use strict';
|
|
|
|
var util = require('util');
|
|
var uuid = require('node-uuid');
|
|
var JobStateMachine = require('./job-state-machine');
|
|
var jobStatus = require('../job-status');
|
|
var mandatoryProperties = [
|
|
'job_id',
|
|
'status',
|
|
'query',
|
|
'created_at',
|
|
'updated_at',
|
|
'host',
|
|
'user'
|
|
];
|
|
|
|
function JobBase (data) {
|
|
JobStateMachine.call(this);
|
|
|
|
var now = new Date().toISOString();
|
|
|
|
this.data = data;
|
|
|
|
if (!this.data.job_id) {
|
|
this.data.job_id = uuid.v4();
|
|
}
|
|
|
|
if (!this.data.created_at) {
|
|
this.data.created_at = now;
|
|
}
|
|
|
|
if (!this.data.updated_at) {
|
|
this.data.updated_at = now;
|
|
}
|
|
}
|
|
util.inherits(JobBase, JobStateMachine);
|
|
|
|
module.exports = JobBase;
|
|
|
|
// should be implemented by childs
|
|
JobBase.prototype.getNextQuery = function () {
|
|
throw new Error('Unimplemented method');
|
|
};
|
|
|
|
JobBase.prototype.hasNextQuery = function () {
|
|
return !!this.getNextQuery();
|
|
};
|
|
|
|
JobBase.prototype.isPending = function () {
|
|
return this.data.status === jobStatus.PENDING;
|
|
};
|
|
|
|
JobBase.prototype.isRunning = function () {
|
|
return this.data.status === jobStatus.RUNNING;
|
|
};
|
|
|
|
JobBase.prototype.isDone = function () {
|
|
return this.data.status === jobStatus.DONE;
|
|
};
|
|
|
|
JobBase.prototype.isCancelled = function () {
|
|
return this.data.status === jobStatus.CANCELLED;
|
|
};
|
|
|
|
JobBase.prototype.isFailed = function () {
|
|
return this.data.status === jobStatus.FAILED;
|
|
};
|
|
|
|
JobBase.prototype.isUnknown = function () {
|
|
return this.data.status === jobStatus.UNKNOWN;
|
|
};
|
|
|
|
JobBase.prototype.setQuery = function (query) {
|
|
var now = new Date().toISOString();
|
|
|
|
if (!this.isPending()) {
|
|
throw new Error('Job is not pending, it cannot be updated');
|
|
}
|
|
|
|
this.data.updated_at = now;
|
|
this.data.query = query;
|
|
};
|
|
|
|
JobBase.prototype.setStatus = function (finalStatus, errorMesssage) {
|
|
var now = new Date().toISOString();
|
|
var initialStatus = this.data.status;
|
|
var isValid = this.isValidTransition(initialStatus, finalStatus);
|
|
|
|
if (!isValid) {
|
|
throw new Error('Cannot set status from ' + initialStatus + ' to ' + finalStatus);
|
|
}
|
|
|
|
this.data.updated_at = now;
|
|
this.data.status = finalStatus;
|
|
if (finalStatus === jobStatus.FAILED && errorMesssage) {
|
|
this.data.failed_reason = errorMesssage;
|
|
}
|
|
};
|
|
|
|
JobBase.prototype.validate = function () {
|
|
for (var i = 0; i < mandatoryProperties.length; i++) {
|
|
if (!this.data[mandatoryProperties[i]]) {
|
|
throw new Error('property "' + mandatoryProperties[i] + '" is mandatory');
|
|
}
|
|
}
|
|
};
|
|
|
|
JobBase.prototype.serialize = function () {
|
|
var data = JSON.parse(JSON.stringify(this.data));
|
|
|
|
delete data.host;
|
|
delete data.dbuser;
|
|
delete data.port;
|
|
delete data.dbname;
|
|
delete data.pass;
|
|
|
|
return data;
|
|
};
|