mirror of
https://github.com/davisking/dlib.git
synced 2024-11-01 10:14:53 +08:00
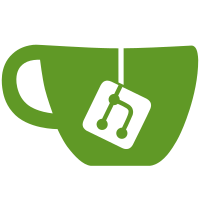
--HG-- extra : convert_revision : svn%3Afdd8eb12-d10e-0410-9acb-85c331704f74/trunk%402875
62 lines
1.6 KiB
C++
62 lines
1.6 KiB
C++
// The contents of this file are in the public domain. See LICENSE_FOR_EXAMPLE_PROGRAMS.txt
|
|
|
|
|
|
/*
|
|
This is an example illustrating the use of the timer object.
|
|
|
|
The timer object is an object that calls some user specified member function
|
|
in its own thread at regular intervals.
|
|
*/
|
|
|
|
|
|
#include "dlib/timer.h"
|
|
#include "dlib/misc_api.h" // for dlib::sleep
|
|
#include <iostream>
|
|
|
|
using namespace dlib;
|
|
using namespace std;
|
|
|
|
// ----------------------------------------------------------------------------------------
|
|
|
|
class timer_example
|
|
{
|
|
public:
|
|
timer_example(
|
|
) :
|
|
// Here we construct our timer object. It needs two things. The second argument is
|
|
// the member function it is going to call at regular intervals and the first
|
|
// argument is the object instance it will call that member function on.
|
|
t(*this,&timer_example::action_function)
|
|
{
|
|
// set the timer object to trigger every second
|
|
t.set_delay_time(1000);
|
|
|
|
// start the timer. It will start calling the action function
|
|
// 1 second from this call to start.
|
|
t.start();
|
|
}
|
|
|
|
private:
|
|
|
|
timer<timer_example>::kernel_2a t;
|
|
|
|
void action_function()
|
|
{
|
|
// print out a message so we can see that this function is being triggered
|
|
cout << "action_function() called" << endl;
|
|
}
|
|
};
|
|
|
|
// ----------------------------------------------------------------------------------------
|
|
|
|
int main()
|
|
{
|
|
timer_example e;
|
|
|
|
// sleep for 10 seconds before letting the program end
|
|
dlib::sleep(10000);
|
|
}
|
|
|
|
// ----------------------------------------------------------------------------------------
|
|
|