mirror of
https://github.com/vector-im/element-web.git
synced 2024-11-30 06:21:09 +08:00
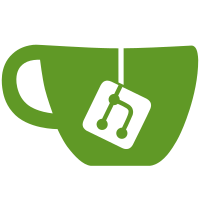
* Upgrade to latest compound-web package * Use a custom render function for jest tests This way we don't need to manually wrap our components with <TooltipProvider> * Pin wrap-ansi to fix broken yarn install * Add playwright helper to find tooltip from element and use it in the failing test * Exclude floating-ui divs/spans from axe testing This is rendered outside .MatrixChat by compound and contains all the tooltips. * Wrap outermost components with TooltipProvider * Remove onChange and use onSelect for toggle * Fix jest tests and update snapshots * Use vector-im/matrix-wysiwig --------- Co-authored-by: Michael Telatynski <7t3chguy@gmail.com>
57 lines
2.0 KiB
TypeScript
57 lines
2.0 KiB
TypeScript
/*
|
|
Copyright 2024 New Vector Ltd.
|
|
Copyright 2022 The Matrix.org Foundation C.I.C.
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only OR GPL-3.0-only
|
|
Please see LICENSE files in the repository root for full details.
|
|
*/
|
|
|
|
import React from "react";
|
|
import { render, screen } from "jest-matrix-react";
|
|
|
|
import LabsUserSettingsTab from "../../../../../../src/components/views/settings/tabs/user/LabsUserSettingsTab";
|
|
import SettingsStore from "../../../../../../src/settings/SettingsStore";
|
|
import SdkConfig from "../../../../../../src/SdkConfig";
|
|
|
|
describe("<LabsUserSettingsTab />", () => {
|
|
const defaultProps = {
|
|
closeSettingsFn: jest.fn(),
|
|
};
|
|
const getComponent = () => <LabsUserSettingsTab {...defaultProps} />;
|
|
|
|
const settingsValueSpy = jest.spyOn(SettingsStore, "getValue");
|
|
|
|
beforeEach(() => {
|
|
jest.clearAllMocks();
|
|
settingsValueSpy.mockReturnValue(false);
|
|
SdkConfig.reset();
|
|
SdkConfig.add({ brand: "BrandedClient" });
|
|
localStorage.clear();
|
|
});
|
|
|
|
it("renders settings marked as beta as beta cards", () => {
|
|
render(getComponent());
|
|
expect(screen.getByText("Upcoming features").parentElement!).toMatchSnapshot();
|
|
});
|
|
|
|
it("does not render non-beta labs settings when disabled in config", () => {
|
|
const sdkConfigSpy = jest.spyOn(SdkConfig, "get");
|
|
render(getComponent());
|
|
expect(sdkConfigSpy).toHaveBeenCalledWith("show_labs_settings");
|
|
|
|
// only section is beta section
|
|
expect(screen.queryByText("Early previews")).not.toBeInTheDocument();
|
|
});
|
|
|
|
it("renders non-beta labs settings when enabled in config", () => {
|
|
// enable labs
|
|
SdkConfig.add({ show_labs_settings: true });
|
|
const { container } = render(getComponent());
|
|
|
|
// non-beta labs section
|
|
expect(screen.getByText("Early previews")).toBeInTheDocument();
|
|
const labsSections = container.getElementsByClassName("mx_SettingsSubsection");
|
|
expect(labsSections).toHaveLength(10);
|
|
});
|
|
});
|