mirror of
https://github.com/vector-im/element-web.git
synced 2024-11-16 13:14:58 +08:00
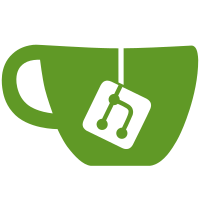
* New room header - add chat button during call - close lobby button in lobby - join button if session exists - allow to toggle call <-> timeline during call with call button Compound style for join button in call notify toast. Signed-off-by: Timo K <toger5@hotmail.de> * dont show start call, join button in video rooms. Signed-off-by: Timo K <toger5@hotmail.de> * Make active call check based on participant count Not based on available call object Signed-off-by: Timo K <toger5@hotmail.de> * fix room header tests Signed-off-by: Timo K <toger5@hotmail.de> * fix room header tests Signed-off-by: Timo K <toger5@hotmail.de> * remove chat button test for displaying. Chat button display logic is now part of the RoomHeader. Signed-off-by: Timo K <toger5@hotmail.de> * remove duplicate notification Tread icon Signed-off-by: Timo K <toger5@hotmail.de> * remove obsolete jest snapshot Signed-off-by: Timo K <toger5@hotmail.de> * Update src/components/views/rooms/RoomHeader.tsx Co-authored-by: Robin <robin@robin.town> * update isECWidget logic Signed-off-by: Timo K <toger5@hotmail.de> * remove dead code Signed-off-by: Timo K <toger5@hotmail.de> * refactor call options Add menu to choose if there are multiple options Signed-off-by: Timo K <toger5@hotmail.de> * join ec when clicking join button (dont start jitsi) Use icon buttons don't show call icon when join button is visible Signed-off-by: Timo K <toger5@hotmail.de> * refactor isViewingCall Signed-off-by: Timo K <toger5@hotmail.de> * fix room header tests Signed-off-by: Timo K <toger5@hotmail.de> * fix header snapshot Signed-off-by: Timo K <toger5@hotmail.de> * sonar proposals Signed-off-by: Timo K <toger5@hotmail.de> * fix event shiftKey may be undefined Signed-off-by: Timo K <toger5@hotmail.de> * more lobby time before timeout only await sticky promise on becoming sticky. Signed-off-by: Timo K <toger5@hotmail.de> * don't allow starting new calls if there is an ongoing other call. Signed-off-by: Timo K <toger5@hotmail.de> * review Signed-off-by: Timo K <toger5@hotmail.de> * fix translation typo Signed-off-by: Timo K <toger5@hotmail.de> --------- Signed-off-by: Timo K <toger5@hotmail.de> Co-authored-by: Robin <robin@robin.town>
136 lines
5.4 KiB
TypeScript
136 lines
5.4 KiB
TypeScript
/*
|
|
Copyright 2023 The Matrix.org Foundation C.I.C.
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
import React from "react";
|
|
import { MockedObject } from "jest-mock";
|
|
import { Room } from "matrix-js-sdk/src/matrix";
|
|
import { fireEvent, render, screen } from "@testing-library/react";
|
|
import { TooltipProvider } from "@vector-im/compound-web";
|
|
|
|
import { VideoRoomChatButton } from "../../../../../src/components/views/rooms/RoomHeader/VideoRoomChatButton";
|
|
import { SDKContext, SdkContextClass } from "../../../../../src/contexts/SDKContext";
|
|
import RightPanelStore from "../../../../../src/stores/right-panel/RightPanelStore";
|
|
import { getMockClientWithEventEmitter, mockClientMethodsUser } from "../../../../test-utils";
|
|
import { RoomNotificationState } from "../../../../../src/stores/notifications/RoomNotificationState";
|
|
import { NotificationLevel } from "../../../../../src/stores/notifications/NotificationLevel";
|
|
import { NotificationStateEvents } from "../../../../../src/stores/notifications/NotificationState";
|
|
import { RightPanelPhases } from "../../../../../src/stores/right-panel/RightPanelStorePhases";
|
|
|
|
describe("<VideoRoomChatButton />", () => {
|
|
const roomId = "!room:server.org";
|
|
let sdkContext!: SdkContextClass;
|
|
let rightPanelStore!: MockedObject<RightPanelStore>;
|
|
|
|
/**
|
|
* Create a room using mocked client
|
|
* And mock isElementVideoRoom
|
|
*/
|
|
const makeRoom = (isVideoRoom = true): Room => {
|
|
const room = new Room(roomId, sdkContext.client!, sdkContext.client!.getSafeUserId());
|
|
jest.spyOn(room, "isElementVideoRoom").mockReturnValue(isVideoRoom);
|
|
// stub
|
|
jest.spyOn(room, "getPendingEvents").mockReturnValue([]);
|
|
return room;
|
|
};
|
|
|
|
const mockRoomNotificationState = (room: Room, level: NotificationLevel): RoomNotificationState => {
|
|
const roomNotificationState = new RoomNotificationState(room, false);
|
|
|
|
// @ts-ignore ugly mocking
|
|
roomNotificationState._level = level;
|
|
jest.spyOn(sdkContext.roomNotificationStateStore, "getRoomState").mockReturnValue(roomNotificationState);
|
|
return roomNotificationState;
|
|
};
|
|
|
|
const getComponent = (room: Room) =>
|
|
render(<VideoRoomChatButton room={room} />, {
|
|
wrapper: ({ children }) => (
|
|
<SDKContext.Provider value={sdkContext}>
|
|
<TooltipProvider>{children}</TooltipProvider>
|
|
</SDKContext.Provider>
|
|
),
|
|
});
|
|
|
|
beforeEach(() => {
|
|
const client = getMockClientWithEventEmitter({
|
|
...mockClientMethodsUser(),
|
|
});
|
|
rightPanelStore = {
|
|
showOrHidePanel: jest.fn(),
|
|
} as unknown as MockedObject<RightPanelStore>;
|
|
sdkContext = new SdkContextClass();
|
|
sdkContext.client = client;
|
|
jest.spyOn(sdkContext, "rightPanelStore", "get").mockReturnValue(rightPanelStore);
|
|
});
|
|
|
|
afterEach(() => {
|
|
jest.restoreAllMocks();
|
|
});
|
|
|
|
it("toggles timeline in right panel on click", () => {
|
|
const room = makeRoom();
|
|
getComponent(room);
|
|
|
|
fireEvent.click(screen.getByLabelText("Chat"));
|
|
|
|
expect(sdkContext.rightPanelStore.showOrHidePanel).toHaveBeenCalledWith(RightPanelPhases.Timeline);
|
|
});
|
|
|
|
it("renders button with an unread marker when room is unread", () => {
|
|
const room = makeRoom();
|
|
mockRoomNotificationState(room, NotificationLevel.Activity);
|
|
getComponent(room);
|
|
|
|
// snapshot includes `data-indicator` attribute
|
|
expect(screen.getByLabelText("Chat")).toMatchSnapshot();
|
|
expect(screen.getByLabelText("Chat").hasAttribute("data-indicator")).toBeTruthy();
|
|
});
|
|
|
|
it("adds unread marker when room notification state changes to unread", () => {
|
|
const room = makeRoom();
|
|
// start in read state
|
|
const notificationState = mockRoomNotificationState(room, NotificationLevel.None);
|
|
getComponent(room);
|
|
|
|
// no unread marker
|
|
expect(screen.getByLabelText("Chat").hasAttribute("data-indicator")).toBeFalsy();
|
|
|
|
// @ts-ignore ugly mocking
|
|
notificationState._level = NotificationLevel.Highlight;
|
|
notificationState.emit(NotificationStateEvents.Update);
|
|
|
|
// unread marker
|
|
expect(screen.getByLabelText("Chat").hasAttribute("data-indicator")).toBeTruthy();
|
|
});
|
|
|
|
it("clears unread marker when room notification state changes to read", () => {
|
|
const room = makeRoom();
|
|
// start in unread state
|
|
const notificationState = mockRoomNotificationState(room, NotificationLevel.Highlight);
|
|
getComponent(room);
|
|
|
|
// unread marker
|
|
expect(screen.getByLabelText("Chat").hasAttribute("data-indicator")).toBeTruthy();
|
|
|
|
// @ts-ignore ugly mocking
|
|
notificationState._level = NotificationLevel.None;
|
|
notificationState.emit(NotificationStateEvents.Update);
|
|
|
|
// unread marker cleared
|
|
expect(screen.getByLabelText("Chat").hasAttribute("data-indicator")).toBeFalsy();
|
|
});
|
|
});
|