mirror of
https://github.com/vector-im/element-web.git
synced 2024-11-23 00:28:48 +08:00
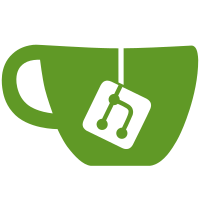
Co-authored-by: github-merge-queue <118344674+github-merge-queue@users.noreply.github.com> Co-authored-by: github-merge-queue <github-merge-queue@users.noreply.github.com> Co-authored-by: renovate[bot] <29139614+renovate[bot]@users.noreply.github.com> Co-authored-by: Florian Duros <florian.duros@ormaz.fr> Co-authored-by: Kim Brose <kim.brose@nordeck.net> Co-authored-by: Florian Duros <florianduros@element.io> Co-authored-by: R Midhun Suresh <hi@midhun.dev> Co-authored-by: dbkr <986903+dbkr@users.noreply.github.com> Co-authored-by: ElementRobot <releases@riot.im> Co-authored-by: dbkr <dbkr@users.noreply.github.com> Co-authored-by: David Baker <dbkr@users.noreply.github.com> Co-authored-by: Michael Telatynski <7t3chguy@gmail.com> Co-authored-by: Richard van der Hoff <1389908+richvdh@users.noreply.github.com> Co-authored-by: David Langley <davidl@element.io> Co-authored-by: Michael Weimann <michaelw@matrix.org> Co-authored-by: Timshel <Timshel@users.noreply.github.com> Co-authored-by: Sahil Silare <32628578+sahil9001@users.noreply.github.com> Co-authored-by: Will Hunt <will@half-shot.uk> Co-authored-by: Hubert Chathi <hubert@uhoreg.ca> Co-authored-by: Andrew Ferrazzutti <andrewf@element.io> Co-authored-by: Robin <robin@robin.town> Co-authored-by: Tulir Asokan <tulir@maunium.net>
138 lines
5.4 KiB
TypeScript
138 lines
5.4 KiB
TypeScript
/*
|
|
Copyright 2024 New Vector Ltd.
|
|
Copyright 2023 The Matrix.org Foundation C.I.C.
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only OR GPL-3.0-only
|
|
Please see LICENSE files in the repository root for full details.
|
|
*/
|
|
|
|
import { type Preset, type Visibility } from "matrix-js-sdk/src/matrix";
|
|
|
|
import { test, expect } from "../../element-web-test";
|
|
import { doTwoWaySasVerification, awaitVerifier } from "./utils";
|
|
import { Client } from "../../pages/client";
|
|
|
|
test.describe("User verification", () => {
|
|
// note that there are other tests that check user verification works in `crypto.spec.ts`.
|
|
|
|
test.use({
|
|
displayName: "Alice",
|
|
botCreateOpts: { displayName: "Bob", autoAcceptInvites: true, userIdPrefix: "bob_" },
|
|
room: async ({ page, app, bot: bob, user: aliceCredentials }, use) => {
|
|
await app.client.bootstrapCrossSigning(aliceCredentials);
|
|
|
|
// the other user creates a DM
|
|
const dmRoomId = await createDMRoom(bob, aliceCredentials.userId);
|
|
|
|
// accept the DM
|
|
await app.viewRoomByName("Bob");
|
|
await page.getByRole("button", { name: "Start chatting" }).click();
|
|
await use({ roomId: dmRoomId });
|
|
},
|
|
});
|
|
|
|
test("can receive a verification request when there is no existing DM", async ({
|
|
page,
|
|
bot: bob,
|
|
user: aliceCredentials,
|
|
toasts,
|
|
room: { roomId: dmRoomId },
|
|
}) => {
|
|
// once Alice has joined, Bob starts the verification
|
|
const bobVerificationRequest = await bob.evaluateHandle(
|
|
async (client, { dmRoomId, aliceCredentials }) => {
|
|
const room = client.getRoom(dmRoomId);
|
|
while (room.getMember(aliceCredentials.userId)?.membership !== "join") {
|
|
await new Promise((resolve) => {
|
|
room.once(window.matrixcs.RoomStateEvent.Members, resolve);
|
|
});
|
|
}
|
|
|
|
return client.getCrypto().requestVerificationDM(aliceCredentials.userId, dmRoomId);
|
|
},
|
|
{ dmRoomId, aliceCredentials },
|
|
);
|
|
|
|
// there should also be a toast
|
|
const toast = await toasts.getToast("Verification requested");
|
|
// it should contain the details of the requesting user
|
|
await expect(toast.getByText(`Bob (${bob.credentials.userId})`)).toBeVisible();
|
|
// Accept
|
|
await toast.getByRole("button", { name: "Verify User" }).click();
|
|
|
|
// request verification by emoji
|
|
await page.locator("#mx_RightPanel").getByRole("button", { name: "Verify by emoji" }).click();
|
|
|
|
/* on the bot side, wait for the verifier to exist ... */
|
|
const botVerifier = await awaitVerifier(bobVerificationRequest);
|
|
// ... confirm ...
|
|
botVerifier.evaluate((verifier) => verifier.verify());
|
|
// ... and then check the emoji match
|
|
await doTwoWaySasVerification(page, botVerifier);
|
|
|
|
await page.getByRole("button", { name: "They match" }).click();
|
|
await expect(page.getByText("You've successfully verified Bob!")).toBeVisible();
|
|
await page.getByRole("button", { name: "Got it" }).click();
|
|
});
|
|
|
|
test("can abort emoji verification when emoji mismatch", async ({
|
|
page,
|
|
bot: bob,
|
|
user: aliceCredentials,
|
|
toasts,
|
|
room: { roomId: dmRoomId },
|
|
}) => {
|
|
// once Alice has joined, Bob starts the verification
|
|
const bobVerificationRequest = await bob.evaluateHandle(
|
|
async (client, { dmRoomId, aliceCredentials }) => {
|
|
const room = client.getRoom(dmRoomId);
|
|
while (room.getMember(aliceCredentials.userId)?.membership !== "join") {
|
|
await new Promise((resolve) => {
|
|
room.once(window.matrixcs.RoomStateEvent.Members, resolve);
|
|
});
|
|
}
|
|
|
|
return client.getCrypto().requestVerificationDM(aliceCredentials.userId, dmRoomId);
|
|
},
|
|
{ dmRoomId, aliceCredentials },
|
|
);
|
|
|
|
// Accept verification via toast
|
|
const toast = await toasts.getToast("Verification requested");
|
|
await toast.getByRole("button", { name: "Verify User" }).click();
|
|
|
|
// request verification by emoji
|
|
await page.locator("#mx_RightPanel").getByRole("button", { name: "Verify by emoji" }).click();
|
|
|
|
/* on the bot side, wait for the verifier to exist ... */
|
|
const botVerifier = await awaitVerifier(bobVerificationRequest);
|
|
// ... confirm ...
|
|
botVerifier.evaluate((verifier) => verifier.verify()).catch(() => {});
|
|
// ... and abort the verification
|
|
await page.getByRole("button", { name: "They don't match" }).click();
|
|
|
|
const dialog = page.locator(".mx_Dialog");
|
|
await expect(dialog.getByText("Your messages are not secure")).toBeVisible();
|
|
await dialog.getByRole("button", { name: "OK" }).click();
|
|
await expect(dialog).not.toBeVisible();
|
|
});
|
|
});
|
|
|
|
async function createDMRoom(client: Client, userId: string): Promise<string> {
|
|
return client.createRoom({
|
|
preset: "trusted_private_chat" as Preset,
|
|
visibility: "private" as Visibility,
|
|
invite: [userId],
|
|
is_direct: true,
|
|
initial_state: [
|
|
{
|
|
type: "m.room.encryption",
|
|
state_key: "",
|
|
content: {
|
|
algorithm: "m.megolm.v1.aes-sha2",
|
|
},
|
|
},
|
|
],
|
|
});
|
|
}
|