mirror of
https://github.com/vector-im/element-call.git
synced 2024-11-27 00:48:06 +08:00
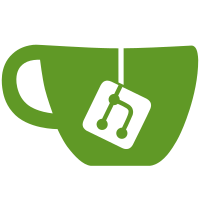
* Initial support for Hand Raise feature Signed-off-by: Milton Moura <miltonmoura@gmail.com> * Refactored to use reaction and redaction events Signed-off-by: Milton Moura <miltonmoura@gmail.com> * Replacing button svg with raised hand emoji Signed-off-by: Milton Moura <miltonmoura@gmail.com> * SpotlightTile should not duplicate the raised hand Signed-off-by: Milton Moura <miltonmoura@gmail.com> * Update src/room/useRaisedHands.tsx Element Call recently changed to AGPL-3.0 * Use relations to load existing reactions when joining the call Signed-off-by: Milton Moura <miltonmoura@gmail.com> * Links to sha commit of matrix-js-sdk that exposes the call membership event id and refactors some async code Signed-off-by: Milton Moura <miltonmoura@gmail.com> * Removing RaiseHand.svg * Check for reaction & redaction capabilities in widget mode Signed-off-by: Milton Moura <miltonmoura@gmail.com> * Fix failing GridTile test Signed-off-by: Milton Moura <miltonmoura@gmail.com> * Center align hand raise. * Add support for displaying the duration of a raised hand. * Add a sound for when a hand is raised. * Refactor raised hand indicator and add tests. * lint * Refactor into own files. * Redact the right thing. * Tidy up useEffect * Lint tests * Remove extra layer * Add better sound. (woosh) * Add a small mode for spotlight * Fix timestamp calculation on relaod. * Fix call border resizing video * lint * Fix and update tests * Allow timer to be configurable. * Add preferences tab for choosing to enable timer. * Drop border from raised hand icon * Handle cases when a new member event happens. * Prevent infinite loop * Major refactor to support various state problems. * Tidy up and finish test rewrites * Add some explanation comments. * Even more comments. * Use proper duration formatter * Remove rerender * Fix redactions not working because they pick up events in transit. * More tidying * Use deferred value * linting * Add tests for cases where we got a reaction from someone else. * Be even less brittle. * Transpose border to GridTile. * lint --------- Signed-off-by: Milton Moura <miltonmoura@gmail.com> Co-authored-by: fkwp <fkwp@users.noreply.github.com> Co-authored-by: Half-Shot <will@half-shot.uk> Co-authored-by: Will Hunt <github@half-shot.uk>
269 lines
8.3 KiB
TypeScript
269 lines
8.3 KiB
TypeScript
/*
|
|
Copyright 2024 New Vector Ltd.
|
|
|
|
SPDX-License-Identifier: AGPL-3.0-only
|
|
Please see LICENSE in the repository root for full details.
|
|
*/
|
|
|
|
import { act, render } from "@testing-library/react";
|
|
import { FC, ReactNode } from "react";
|
|
import { describe, expect, test } from "vitest";
|
|
import {
|
|
MatrixRTCSession,
|
|
MatrixRTCSessionEvent,
|
|
} from "matrix-js-sdk/src/matrixrtc/MatrixRTCSession";
|
|
import {
|
|
EventTimeline,
|
|
EventTimelineSet,
|
|
EventType,
|
|
MatrixClient,
|
|
MatrixEvent,
|
|
Room,
|
|
RoomEvent,
|
|
} from "matrix-js-sdk/src/matrix";
|
|
import EventEmitter from "events";
|
|
import { randomUUID } from "crypto";
|
|
|
|
import { ReactionsProvider, useReactions } from "./useReactions";
|
|
|
|
/**
|
|
* Test explanation.
|
|
* This test suite checks that the useReactions hook appropriately reacts
|
|
* to new reactions, redactions and membership changesin the room. There is
|
|
* a large amount of test structure used to construct a mock environment.
|
|
*/
|
|
|
|
const memberUserIdAlice = "@alice:example.org";
|
|
const memberEventAlice = "$membership-alice:example.org";
|
|
const memberUserIdBob = "@bob:example.org";
|
|
const memberEventBob = "$membership-bob:example.org";
|
|
|
|
const membership: Record<string, string> = {
|
|
[memberEventAlice]: memberUserIdAlice,
|
|
[memberEventBob]: memberUserIdBob,
|
|
"$membership-charlie:example.org": "@charlie:example.org",
|
|
};
|
|
|
|
const TestComponent: FC = () => {
|
|
const { raisedHands, myReactionId } = useReactions();
|
|
return (
|
|
<div>
|
|
<ul>
|
|
{Object.entries(raisedHands).map(([userId, date]) => (
|
|
<li key={userId}>
|
|
<span>{userId}</span>
|
|
<time>{date.getTime()}</time>
|
|
</li>
|
|
))}
|
|
</ul>
|
|
<p>{myReactionId ? "Local reaction" : "No local reaction"}</p>
|
|
</div>
|
|
);
|
|
};
|
|
|
|
const TestComponentWrapper = ({
|
|
rtcSession,
|
|
}: {
|
|
rtcSession: MockRTCSession;
|
|
}): ReactNode => {
|
|
return (
|
|
<ReactionsProvider rtcSession={rtcSession as unknown as MatrixRTCSession}>
|
|
<TestComponent />
|
|
</ReactionsProvider>
|
|
);
|
|
};
|
|
|
|
export class MockRTCSession extends EventEmitter {
|
|
public memberships = Object.entries(membership).map(([eventId, sender]) => ({
|
|
sender,
|
|
eventId,
|
|
createdTs: (): Date => new Date(),
|
|
}));
|
|
|
|
public constructor(public readonly room: MockRoom) {
|
|
super();
|
|
}
|
|
|
|
public testRemoveMember(userId: string): void {
|
|
this.memberships = this.memberships.filter((u) => u.sender !== userId);
|
|
this.emit(MatrixRTCSessionEvent.MembershipsChanged);
|
|
}
|
|
|
|
public testAddMember(sender: string): void {
|
|
this.memberships.push({
|
|
sender,
|
|
eventId: `!fake-${randomUUID()}:event`,
|
|
createdTs: (): Date => new Date(),
|
|
});
|
|
this.emit(MatrixRTCSessionEvent.MembershipsChanged);
|
|
}
|
|
}
|
|
|
|
function createReaction(
|
|
parentMemberEvent: string,
|
|
overridenSender?: string,
|
|
): MatrixEvent {
|
|
return new MatrixEvent({
|
|
sender: overridenSender ?? membership[parentMemberEvent],
|
|
type: EventType.Reaction,
|
|
origin_server_ts: new Date().getTime(),
|
|
content: {
|
|
"m.relates_to": {
|
|
key: "🖐️",
|
|
event_id: parentMemberEvent,
|
|
},
|
|
},
|
|
event_id: randomUUID(),
|
|
});
|
|
}
|
|
|
|
function createRedaction(sender: string, reactionEventId: string): MatrixEvent {
|
|
return new MatrixEvent({
|
|
sender,
|
|
type: EventType.RoomRedaction,
|
|
origin_server_ts: new Date().getTime(),
|
|
redacts: reactionEventId,
|
|
content: {},
|
|
event_id: randomUUID(),
|
|
});
|
|
}
|
|
|
|
export class MockRoom extends EventEmitter {
|
|
public constructor(private readonly existingRelations: MatrixEvent[] = []) {
|
|
super();
|
|
}
|
|
|
|
public get client(): MatrixClient {
|
|
return {
|
|
getUserId: (): string => memberUserIdAlice,
|
|
} as unknown as MatrixClient;
|
|
}
|
|
|
|
public get relations(): Room["relations"] {
|
|
return {
|
|
getChildEventsForEvent: (membershipEventId: string) => ({
|
|
getRelations: (): MatrixEvent[] => {
|
|
return this.existingRelations.filter(
|
|
(r) =>
|
|
r.getContent()["m.relates_to"]?.event_id === membershipEventId,
|
|
);
|
|
},
|
|
}),
|
|
} as unknown as Room["relations"];
|
|
}
|
|
|
|
public testSendReaction(
|
|
parentMemberEvent: string,
|
|
overridenSender?: string,
|
|
): string {
|
|
const evt = createReaction(parentMemberEvent, overridenSender);
|
|
this.emit(RoomEvent.Timeline, evt, this, undefined, false, {
|
|
timeline: new EventTimeline(new EventTimelineSet(undefined)),
|
|
});
|
|
return evt.getId()!;
|
|
}
|
|
}
|
|
|
|
describe("useReactions", () => {
|
|
test("starts with an empty list", () => {
|
|
const rtcSession = new MockRTCSession(new MockRoom());
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(0);
|
|
});
|
|
test("handles own raised hand", async () => {
|
|
const room = new MockRoom();
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByText } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
await act(() => room.testSendReaction(memberEventAlice));
|
|
expect(queryByText("Local reaction")).toBeTruthy();
|
|
});
|
|
test("handles incoming raised hand", async () => {
|
|
const room = new MockRoom();
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
await act(() => room.testSendReaction(memberEventAlice));
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(1);
|
|
await act(() => room.testSendReaction(memberEventBob));
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(2);
|
|
});
|
|
test("handles incoming unraised hand", async () => {
|
|
const room = new MockRoom();
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
const reactionEventId = await act(() =>
|
|
room.testSendReaction(memberEventAlice),
|
|
);
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(1);
|
|
await act(() =>
|
|
room.emit(
|
|
RoomEvent.Redaction,
|
|
createRedaction(memberUserIdAlice, reactionEventId),
|
|
room,
|
|
undefined,
|
|
),
|
|
);
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(0);
|
|
});
|
|
test("handles loading prior raised hand events", () => {
|
|
const room = new MockRoom([createReaction(memberEventAlice)]);
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(1);
|
|
});
|
|
// If the membership event changes for a user, we want to remove
|
|
// the raised hand event.
|
|
test("will remove reaction when a member leaves the call", () => {
|
|
const room = new MockRoom([createReaction(memberEventAlice)]);
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(1);
|
|
act(() => rtcSession.testRemoveMember(memberUserIdAlice));
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(0);
|
|
});
|
|
test("will remove reaction when a member joins via a new event", () => {
|
|
const room = new MockRoom([createReaction(memberEventAlice)]);
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(1);
|
|
// Simulate leaving and rejoining
|
|
act(() => {
|
|
rtcSession.testRemoveMember(memberUserIdAlice);
|
|
rtcSession.testAddMember(memberUserIdAlice);
|
|
});
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(0);
|
|
});
|
|
test("ignores invalid sender for historic event", () => {
|
|
const room = new MockRoom([
|
|
createReaction(memberEventAlice, memberUserIdBob),
|
|
]);
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(0);
|
|
});
|
|
test("ignores invalid sender for new event", async () => {
|
|
const room = new MockRoom([]);
|
|
const rtcSession = new MockRTCSession(room);
|
|
const { queryByRole } = render(
|
|
<TestComponentWrapper rtcSession={rtcSession} />,
|
|
);
|
|
await act(() => room.testSendReaction(memberEventAlice, memberUserIdBob));
|
|
expect(queryByRole("list")?.children).to.have.lengthOf(0);
|
|
});
|
|
});
|