mirror of
https://github.com/vector-im/element-call.git
synced 2024-11-27 00:48:06 +08:00
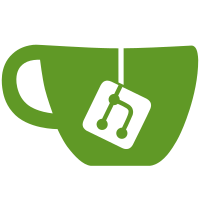
by fixing the cause rather than the symptom: this upgrades the code to use the new, recommended JSX transform mode of React 17+, which no longer requires you to import React manually just to write JSX.
63 lines
1.5 KiB
TypeScript
63 lines
1.5 KiB
TypeScript
import { Room, RoomOptions } from "livekit-client";
|
|
import { useLiveKitRoom, useToken } from "@livekit/components-react";
|
|
import { useMemo } from "react";
|
|
|
|
import { defaultLiveKitOptions } from "./options";
|
|
|
|
export type UserChoices = {
|
|
audio?: DeviceChoices;
|
|
video?: DeviceChoices;
|
|
};
|
|
|
|
export type DeviceChoices = {
|
|
selectedId: string;
|
|
enabled: boolean;
|
|
};
|
|
|
|
export type LiveKitConfig = {
|
|
sfuUrl: string;
|
|
jwtUrl: string;
|
|
roomName: string;
|
|
userDisplayName: string;
|
|
userIdentity: string;
|
|
};
|
|
|
|
export function useLiveKit(
|
|
userChoices: UserChoices,
|
|
config: LiveKitConfig
|
|
): Room | undefined {
|
|
const tokenOptions = useMemo(
|
|
() => ({
|
|
userInfo: {
|
|
name: config.userDisplayName,
|
|
identity: config.userIdentity,
|
|
},
|
|
}),
|
|
[config.userDisplayName, config.userIdentity]
|
|
);
|
|
const token = useToken(config.jwtUrl, config.roomName, tokenOptions);
|
|
|
|
const roomOptions = useMemo((): RoomOptions => {
|
|
const options = defaultLiveKitOptions;
|
|
options.videoCaptureDefaults = {
|
|
...options.videoCaptureDefaults,
|
|
deviceId: userChoices.video?.selectedId,
|
|
};
|
|
options.audioCaptureDefaults = {
|
|
...options.audioCaptureDefaults,
|
|
deviceId: userChoices.audio?.selectedId,
|
|
};
|
|
return options;
|
|
}, [userChoices.video, userChoices.audio]);
|
|
|
|
const { room } = useLiveKitRoom({
|
|
token,
|
|
serverUrl: config.sfuUrl,
|
|
audio: userChoices.audio?.enabled ?? false,
|
|
video: userChoices.video?.enabled ?? false,
|
|
options: roomOptions,
|
|
});
|
|
|
|
return room;
|
|
}
|