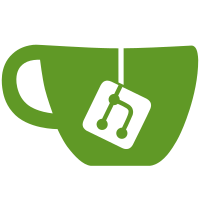
* Fix for Hourly Cron We should be using whereTime instead of whereDate https://laravel.com/docs/8.x/queries#additional-where-clauses `The whereDate method may be used to compare a column's value against a date` * Fix for RemoveExpiredBids I used where to do check 'cause people may set 48 hours to remove a bid, thus neither whereDate nor whereTime will give a correct results. * Fix for DeletePireps I used where to do check 'cause people may set 48 hours to delete cancelled or rejected pireps, thus neither whereDate nor whereTime will give a correct results.
53 lines
1.5 KiB
PHP
53 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace App\Cron\Hourly;
|
|
|
|
use App\Contracts\Listener;
|
|
use App\Events\CronHourly;
|
|
use App\Models\Enums\PirepState;
|
|
use App\Models\Pirep;
|
|
use App\Services\PirepService;
|
|
use Carbon\Carbon;
|
|
use Illuminate\Support\Facades\Log;
|
|
|
|
/**
|
|
* Remove cancelled/deleted PIREPs. Look for PIREPs that were created before the setting time
|
|
* (e.g, 12 hours ago) and are marked with the
|
|
*/
|
|
class DeletePireps extends Listener
|
|
{
|
|
/**
|
|
* Remove expired bids
|
|
*
|
|
* @param CronHourly $event
|
|
*
|
|
* @throws \Exception
|
|
*/
|
|
public function handle(CronHourly $event): void
|
|
{
|
|
$this->deletePireps(setting('pireps.delete_rejected_hours'), PirepState::REJECTED);
|
|
$this->deletePireps(setting('pireps.delete_cancelled_hours'), PirepState::CANCELLED);
|
|
}
|
|
|
|
/**
|
|
* Look for and delete PIREPs which match the criteria
|
|
*
|
|
* @param int $expire_time_hours The time in hours to look for PIREPs
|
|
* @param int $state The PirepState enum value
|
|
*/
|
|
protected function deletePireps(int $expire_time_hours, int $state)
|
|
{
|
|
$dt = Carbon::now()->subHours($expire_time_hours);
|
|
$pireps = Pirep::where('created_at', '<', $dt)->where(['state' => $state])->get();
|
|
|
|
/** @var PirepService $pirepSvc */
|
|
$pirepSvc = app(PirepService::class);
|
|
|
|
/** @var Pirep $pirep */
|
|
foreach ($pireps as $pirep) {
|
|
Log::info('Cron: Deleting PIREP id='.$pirep->id.', state='.PirepState::label($state));
|
|
$pirepSvc->delete($pirep);
|
|
}
|
|
}
|
|
}
|