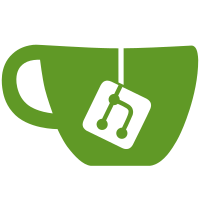
* Update module generation #714 * Fix method signatures * Fix paths within stubs, use single provider.stub file * Add separate index controller * Update module generation #714 * Fix method signatures * Fix paths within stubs, use single provider.stub file * Update module generation Disable/lower the cache time as found as a workaround in https://github.com/nWidart/laravel-modules/issues/995 * Update editorconfig for line endings * Formatting * Formatting
102 lines
2.8 KiB
PHP
102 lines
2.8 KiB
PHP
<?php
|
|
|
|
namespace Modules\Installer\Providers;
|
|
|
|
use App\Contracts\Modules\ServiceProvider;
|
|
use Illuminate\Support\Facades\Route;
|
|
|
|
class InstallerServiceProvider extends ServiceProvider
|
|
{
|
|
/**
|
|
* Boot the application events.
|
|
*/
|
|
public function boot(): void
|
|
{
|
|
$this->registerRoutes();
|
|
$this->registerTranslations();
|
|
$this->registerConfig();
|
|
$this->registerViews();
|
|
}
|
|
|
|
/**
|
|
* Register the routes
|
|
*/
|
|
protected function registerRoutes()
|
|
{
|
|
Route::group([
|
|
'as' => 'installer.',
|
|
'prefix' => 'install',
|
|
'middleware' => ['web'],
|
|
'namespace' => 'Modules\Installer\Http\Controllers',
|
|
], function () {
|
|
Route::get('/', 'InstallerController@index')->name('index');
|
|
Route::post('/dbtest', 'InstallerController@dbtest')->name('dbtest');
|
|
|
|
Route::get('/step1', 'InstallerController@step1')->name('step1');
|
|
Route::post('/step1', 'InstallerController@step1')->name('step1post');
|
|
|
|
Route::get('/step2', 'InstallerController@step2')->name('step2');
|
|
Route::post('/envsetup', 'InstallerController@envsetup')->name('envsetup');
|
|
Route::get('/dbsetup', 'InstallerController@dbsetup')->name('dbsetup');
|
|
|
|
Route::get('/step3', 'InstallerController@step3')->name('step3');
|
|
Route::post('/usersetup', 'InstallerController@usersetup')->name('usersetup');
|
|
|
|
Route::get('/complete', 'InstallerController@complete')->name('complete');
|
|
});
|
|
}
|
|
|
|
/**
|
|
* Register config.
|
|
*/
|
|
protected function registerConfig()
|
|
{
|
|
$this->mergeConfigFrom(__DIR__.'/../Config/config.php', 'installer');
|
|
}
|
|
|
|
/**
|
|
* Register views.
|
|
*/
|
|
public function registerViews()
|
|
{
|
|
$viewPath = resource_path('views/modules/installer');
|
|
$sourcePath = __DIR__.'/../Resources/views';
|
|
|
|
$this->publishes([
|
|
$sourcePath => $viewPath,
|
|
], 'views');
|
|
|
|
$paths = array_map(
|
|
function ($path) {
|
|
return $path.'/modules/installer';
|
|
},
|
|
\Config::get('view.paths')
|
|
);
|
|
|
|
$paths[] = $sourcePath;
|
|
$this->loadViewsFrom($paths, 'installer');
|
|
}
|
|
|
|
/**
|
|
* Register translations.
|
|
*/
|
|
public function registerTranslations()
|
|
{
|
|
$langPath = resource_path('lang/modules/installer');
|
|
|
|
if (is_dir($langPath)) {
|
|
$this->loadTranslationsFrom($langPath, 'installer');
|
|
} else {
|
|
$this->loadTranslationsFrom(__DIR__.'/../Resources/lang', 'installer');
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Get the services provided by the provider.
|
|
*/
|
|
public function provides(): array
|
|
{
|
|
return [];
|
|
}
|
|
}
|