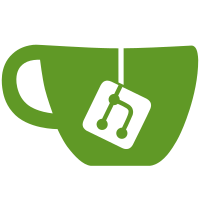
* Split the importer module out from the installer module * Cleanup of unused imports * Move updater into separate module #453 * Remove unused imports/formatting * Disable the install and importer modules at the end of the setup * Unused imports; update IJ style * test explicit stage for php+mysql * add more to matrix * Add different MariaDB versions * undo
71 lines
2.1 KiB
PHP
71 lines
2.1 KiB
PHP
<?php
|
|
|
|
namespace Modules\Importer\Services\Importers;
|
|
|
|
use App\Models\Flight;
|
|
use Modules\Importer\Services\BaseImporter;
|
|
|
|
class FlightImporter extends BaseImporter
|
|
{
|
|
protected $table = 'schedules';
|
|
|
|
public function run($start = 0)
|
|
{
|
|
$this->comment('--- FLIGHT SCHEDULE IMPORT ---');
|
|
|
|
$fields = [
|
|
'id',
|
|
'code',
|
|
'flightnum',
|
|
'depicao',
|
|
'arricao',
|
|
'route',
|
|
'distance',
|
|
'flightlevel',
|
|
'deptime',
|
|
'arrtime',
|
|
'flightttime',
|
|
'notes',
|
|
'enabled',
|
|
];
|
|
|
|
$count = 0;
|
|
foreach ($this->db->readRows($this->table, $start, $fields) as $row) {
|
|
$airline_id = $this->idMapper->getMapping('airlines', $row->code);
|
|
|
|
$flight_num = trim($row->flightnum);
|
|
|
|
$attrs = [
|
|
'dpt_airport_id' => $row->depicao,
|
|
'arr_airport_id' => $row->arricao,
|
|
'route' => $row->route ?: '',
|
|
'distance' => round($row->distance ?: 0, 2),
|
|
'level' => $row->flightlevel ?: 0,
|
|
'dpt_time' => $row->deptime ?: '',
|
|
'arr_time' => $row->arrtime ?: '',
|
|
'flight_time' => $this->convertDuration($row->flighttime) ?: '',
|
|
'notes' => $row->notes ?: '',
|
|
'active' => $row->enabled ?: true,
|
|
];
|
|
|
|
try {
|
|
$w = ['airline_id' => $airline_id, 'flight_number' => $flight_num];
|
|
// $flight = Flight::updateOrCreate($w, $attrs);
|
|
$flight = Flight::create(array_merge($w, $attrs));
|
|
} catch (\Exception $e) {
|
|
//$this->error($e);
|
|
}
|
|
|
|
$this->idMapper->addMapping('flights', $row->id, $flight->id);
|
|
|
|
// TODO: deserialize route_details into ACARS table
|
|
|
|
if ($flight->wasRecentlyCreated) {
|
|
$count++;
|
|
}
|
|
}
|
|
|
|
$this->info('Imported '.$count.' flights');
|
|
}
|
|
}
|