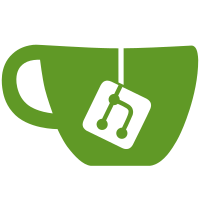
* Split the importer module out from the installer module * Cleanup of unused imports * Move updater into separate module #453 * Remove unused imports/formatting * Disable the install and importer modules at the end of the setup * Unused imports; update IJ style * test explicit stage for php+mysql * add more to matrix * Add different MariaDB versions * undo
66 lines
1.5 KiB
PHP
66 lines
1.5 KiB
PHP
<?php
|
|
|
|
namespace Modules\Importer\Services\Importers;
|
|
|
|
use App\Models\Aircraft;
|
|
use App\Models\Airline;
|
|
use App\Models\Subfleet;
|
|
use Modules\Importer\Services\BaseImporter;
|
|
|
|
class AircraftImporter extends BaseImporter
|
|
{
|
|
public $table = 'aircraft';
|
|
|
|
/**
|
|
* CONSTANTS
|
|
*/
|
|
public const SUBFLEET_NAME = 'Imported Aircraft';
|
|
|
|
public function run($start = 0)
|
|
{
|
|
$this->comment('--- AIRCRAFT IMPORT ---');
|
|
|
|
$subfleet = $this->getSubfleet();
|
|
|
|
$this->info('Subfleet ID is '.$subfleet->id);
|
|
|
|
$count = 0;
|
|
foreach ($this->db->readRows($this->table, $start) as $row) {
|
|
$where = [
|
|
'name' => $row->fullname,
|
|
'registration' => $row->registration,
|
|
];
|
|
|
|
$aircraft = Aircraft::firstOrCreate($where, [
|
|
'icao' => $row->icao,
|
|
'subfleet_id' => $subfleet->id,
|
|
'active' => $row->enabled,
|
|
]);
|
|
|
|
$this->idMapper->addMapping('aircraft', $row->id, $aircraft->id);
|
|
|
|
if ($aircraft->wasRecentlyCreated) {
|
|
$count++;
|
|
}
|
|
}
|
|
|
|
$this->info('Imported '.$count.' aircraft');
|
|
}
|
|
|
|
/**
|
|
* Return the subfleet
|
|
*
|
|
* @return mixed
|
|
*/
|
|
protected function getSubfleet()
|
|
{
|
|
$airline = Airline::first();
|
|
$subfleet = Subfleet::firstOrCreate([
|
|
'airline_id' => $airline->id,
|
|
'name' => self::SUBFLEET_NAME,
|
|
], ['type' => 'PHPVMS']);
|
|
|
|
return $subfleet;
|
|
}
|
|
}
|