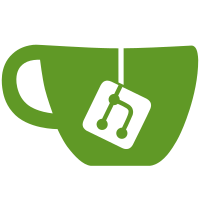
* Add alternative to using the artisan schedule runner * StyleCI fixes * Add additional cron time periods * Style fixes * Typo * Update the web cron to use the new system * Write out JSON for which tasks were run * Rename cron.php to just cron
73 lines
2.4 KiB
PHP
Executable File
73 lines
2.4 KiB
PHP
Executable File
<?php
|
|
|
|
namespace App\Console;
|
|
|
|
use App\Console\Cron\FifteenMinute;
|
|
use App\Console\Cron\FiveMinute;
|
|
use App\Console\Cron\Hourly;
|
|
use App\Console\Cron\JobQueue;
|
|
use App\Console\Cron\Monthly;
|
|
use App\Console\Cron\Nightly;
|
|
use App\Console\Cron\ThirtyMinute;
|
|
use App\Console\Cron\Weekly;
|
|
use App\Services\CronService;
|
|
use Illuminate\Console\Scheduling\Schedule;
|
|
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
|
|
|
|
class Kernel extends ConsoleKernel
|
|
{
|
|
/**
|
|
* Define the application's command schedule. How this works... according to the command
|
|
* time, an event gets send out with the appropriate time (e.g, hourly sends an hourly event)
|
|
*
|
|
* Then the CronServiceProvider has the list of cronjobs which then run according to the events
|
|
* and then calls those at the proper times.
|
|
*
|
|
* @param \Illuminate\Console\Scheduling\Schedule $schedule
|
|
*
|
|
* @return void
|
|
*/
|
|
protected function schedule(Schedule $schedule): void
|
|
{
|
|
// If not using the queue worker then run those via cron
|
|
if (!config('queue.worker', false)) {
|
|
$schedule->command(JobQueue::class)
|
|
->everyMinute()
|
|
->withoutOverlapping();
|
|
}
|
|
|
|
/*
|
|
* NOTE: IF MORE TASKS ARE ADDED, THEY ALSO MUST BE ADDED TO THE CRON.PHP
|
|
*/
|
|
$schedule->command(FiveMinute::class)->everyFiveMinutes();
|
|
$schedule->command(FifteenMinute::class)->everyFifteenMinutes();
|
|
$schedule->command(ThirtyMinute::class)->everyThirtyMinutes();
|
|
$schedule->command(Nightly::class)->dailyAt('01:00');
|
|
$schedule->command(Hourly::class)->hourly();
|
|
$schedule->command(Weekly::class)->weeklyOn(0);
|
|
$schedule->command(Monthly::class)->monthlyOn(1);
|
|
|
|
// When spatie-backups runs
|
|
/*if (config('backup.backup.enabled', false) === true) {
|
|
$schedule->command('backup:clean')->daily()->at('01:00');
|
|
$schedule->command('backup:run')->daily()->at('02:00');
|
|
}*/
|
|
|
|
// Update the last time the cron was run
|
|
/** @var CronService $cronSvc */
|
|
$cronSvc = app(CronService::class);
|
|
$cronSvc->updateLastRunTime();
|
|
}
|
|
|
|
/**
|
|
* Register the Closure based commands for the application.
|
|
*
|
|
* @return void
|
|
*/
|
|
protected function commands(): void
|
|
{
|
|
$this->load(__DIR__.'/Commands');
|
|
$this->load(__DIR__.'/Cron');
|
|
}
|
|
}
|