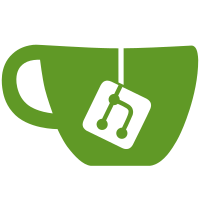
Add the following files: python3-flightgear/README-l10n.txt python3-flightgear/fg-convert-translation-files python3-flightgear/fg-new-translations python3-flightgear/fg-update-translation-files python3-flightgear/flightgear/__init__.py python3-flightgear/flightgear/meta/__init__.py python3-flightgear/flightgear/meta/exceptions.py python3-flightgear/flightgear/meta/i18n.py python3-flightgear/flightgear/meta/logging.py python3-flightgear/flightgear/meta/misc.py They should work on Python 3.4 and later (tested with 3.5.3). The folder structure is chosen so that other FG support modules can insert themselves here, and possibly be used together. I put all of these inside 'flightgear.meta', because I don't expect them to be needed at FG runtime (neither now nor in the future), probably not even by the CMake build system. To declare that a string has plural forms, simply set the attribute 'with-plural' to 'true' on the corresponding element of the default translation (and as in Qt, use %n as a placeholder for the number that determines which singular or plural form to use).
82 lines
2.7 KiB
Python
82 lines
2.7 KiB
Python
# -*- coding: utf-8 -*-
|
|
|
|
# misc.py --- Miscellaneous classes and/or functions
|
|
# Copyright (C) 2015-2017 Florent Rougon
|
|
#
|
|
# This program is free software; you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation; either version 2 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License along
|
|
# with this program; if not, write to the Free Software Foundation, Inc.,
|
|
# 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
|
|
|
|
import enum
|
|
|
|
# Based on an example from the 'enum' documentation
|
|
class OrderedEnum(enum.Enum):
|
|
"""Base class for enumerations whose members can be ordered.
|
|
|
|
Contrary to enum.IntEnum, this class maintains normal enum.Enum
|
|
invariants, such as members not being comparable to members of other
|
|
enumerations (nor of any other class, actually).
|
|
|
|
"""
|
|
def __ge__(self, other):
|
|
if self.__class__ is other.__class__:
|
|
return self.value >= other.value
|
|
return NotImplemented
|
|
|
|
def __gt__(self, other):
|
|
if self.__class__ is other.__class__:
|
|
return self.value > other.value
|
|
return NotImplemented
|
|
|
|
def __le__(self, other):
|
|
if self.__class__ is other.__class__:
|
|
return self.value <= other.value
|
|
return NotImplemented
|
|
|
|
def __lt__(self, other):
|
|
if self.__class__ is other.__class__:
|
|
return self.value < other.value
|
|
return NotImplemented
|
|
|
|
def __eq__(self, other):
|
|
if self.__class__ is other.__class__:
|
|
return self.value == other.value
|
|
return NotImplemented
|
|
|
|
def __ne__(self, other):
|
|
if self.__class__ is other.__class__:
|
|
return self.value != other.value
|
|
return NotImplemented
|
|
|
|
|
|
# Taken from <http://effbot.org/zone/element-lib.htm#prettyprint> and modified
|
|
# by Florent Rougon
|
|
def indentXmlTree(elem, level=0, basicOffset=2, lastChild=False):
|
|
def indentation(level):
|
|
return "\n" + level*basicOffset*" "
|
|
|
|
if len(elem):
|
|
if not elem.text or not elem.text.strip():
|
|
elem.text = indentation(level+1)
|
|
|
|
for e in elem[:-1]:
|
|
indentXmlTree(e, level+1, basicOffset, False)
|
|
if len(elem):
|
|
indentXmlTree(elem[-1], level+1, basicOffset, True)
|
|
|
|
if level and (not elem.tail or not elem.tail.strip()):
|
|
if lastChild:
|
|
elem.tail = indentation(level-1)
|
|
else:
|
|
elem.tail = indentation(level)
|