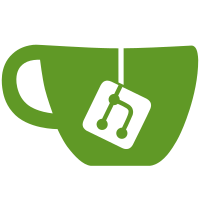
the depth of all drawables, so that it is always safe for RenderBin sort routines can use these values directly. Add an example of a RenderBin::SortCallback to sgv.cpp.
146 lines
4.4 KiB
Plaintext
146 lines
4.4 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2002 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGUTIL_RENDERBIN
|
|
#define OSGUTIL_RENDERBIN 1
|
|
|
|
#include <osgUtil/RenderGraph>
|
|
|
|
#include <map>
|
|
#include <vector>
|
|
#include <string>
|
|
|
|
// forward declare Statistics to remove link dependancy.
|
|
namespace osg { class Statistics; }
|
|
|
|
namespace osgUtil {
|
|
|
|
class RenderStage;
|
|
|
|
/**
|
|
* RenderBin base class.
|
|
*/
|
|
class OSGUTIL_EXPORT RenderBin : public osg::Object
|
|
{
|
|
public:
|
|
|
|
typedef std::vector<RenderLeaf*> RenderLeafList;
|
|
typedef std::vector<RenderGraph*> RenderGraphList;
|
|
typedef std::map< int, osg::ref_ptr<RenderBin> > RenderBinList;
|
|
|
|
// static methods.
|
|
static RenderBin* createRenderBin(const std::string& binName);
|
|
static RenderBin* getRenderBinPrototype(const std::string& binName);
|
|
static void addRenderBinPrototype(const std::string& binName,RenderBin* proto);
|
|
static void removeRenderBinPrototype(RenderBin* proto);
|
|
|
|
enum SortMode
|
|
{
|
|
SORT_BY_STATE,
|
|
SORT_FRONT_TO_BACK,
|
|
SORT_BACK_TO_FRONT
|
|
};
|
|
|
|
|
|
RenderBin(SortMode mode=SORT_BY_STATE);
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
RenderBin(const RenderBin& rhs,const osg::CopyOp& copyop=osg::CopyOp::SHALLOW_COPY);
|
|
|
|
virtual osg::Object* cloneType() const { return osgNew RenderBin(); }
|
|
virtual osg::Object* clone(const osg::CopyOp& copyop) const { return osgNew RenderBin(*this,copyop); } // note only implements a clone of type.
|
|
virtual bool isSameKindAs(const osg::Object* obj) const { return dynamic_cast<const RenderBin*>(obj)!=0L; }
|
|
virtual const char* libraryName() const { return "osgUtil"; }
|
|
virtual const char* className() const { return "RenderBin"; }
|
|
|
|
virtual void reset();
|
|
|
|
RenderBin* find_or_insert(int binNum,const std::string& binName);
|
|
|
|
void addRenderGraph(RenderGraph* rg)
|
|
{
|
|
_renderGraphList.push_back(rg);
|
|
}
|
|
|
|
void sort();
|
|
|
|
void setSortMode(SortMode mode);
|
|
SortMode getSortMode() const { return _sortMode; }
|
|
|
|
virtual void sort_local();
|
|
virtual void sort_local_by_state();
|
|
virtual void sort_local_front_to_back();
|
|
virtual void sort_local_back_to_front();
|
|
|
|
struct SortCallback : public osg::Referenced
|
|
{
|
|
virtual void sort(RenderBin*) = 0;
|
|
};
|
|
|
|
void setSortLocalCallback(SortCallback* sortCallback) { _sortLocalCallback = sortCallback; }
|
|
SortCallback* getSortLocalCallback() { return _sortLocalCallback.get(); }
|
|
const SortCallback* getSortLocalCallback() const { return _sortLocalCallback.get(); }
|
|
|
|
|
|
virtual void draw(osg::State& state,RenderLeaf*& previous);
|
|
|
|
virtual void draw_local(osg::State& state,RenderLeaf*& previous);
|
|
|
|
/** extract stats for current draw list. */
|
|
bool getStats(osg::Statistics* primStats);
|
|
void getPrims(osg::Statistics* primStats);
|
|
bool getPrims(osg::Statistics* primStats, int nbin);
|
|
|
|
|
|
public:
|
|
|
|
|
|
void copyLeavesFromRenderGraphListToRenderLeafList();
|
|
|
|
int _binNum;
|
|
RenderBin* _parent;
|
|
RenderStage* _stage;
|
|
RenderBinList _bins;
|
|
RenderGraphList _renderGraphList;
|
|
RenderLeafList _renderLeafList;
|
|
|
|
|
|
SortMode _sortMode;
|
|
osg::ref_ptr<SortCallback> _sortLocalCallback;
|
|
|
|
|
|
typedef std::map< std::string, osg::ref_ptr<RenderBin> > RenderBinPrototypeList;
|
|
static RenderBinPrototypeList s_renderBinPrototypeList;
|
|
|
|
protected:
|
|
|
|
virtual ~RenderBin();
|
|
|
|
};
|
|
|
|
/** Proxy class for automatic registration of renderbins with the RenderBin prototypelist.*/
|
|
class RegisterRenderBinProxy
|
|
{
|
|
public:
|
|
RegisterRenderBinProxy(const std::string& binName,RenderBin* proto)
|
|
{
|
|
_rb = proto;
|
|
RenderBin::addRenderBinPrototype(binName,_rb.get());
|
|
}
|
|
|
|
~RegisterRenderBinProxy()
|
|
{
|
|
RenderBin::removeRenderBinPrototype(_rb.get());
|
|
}
|
|
|
|
protected:
|
|
osg::ref_ptr<RenderBin> _rb;
|
|
};
|
|
|
|
|
|
}
|
|
|
|
#endif
|
|
|