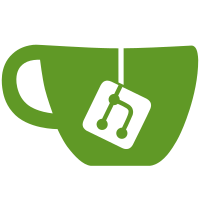
PositionAttitudeTransform, removed the equivialnt callbacks once found in these transform classes. Changed the NodeCallback class so its derived from osg::Object instead of osg::Referenced to allow it to be saved out in the .osg format. Added support for Update and Cull callbacks into the .osg file format. Added support for AnimationPathCallback into the .osg file format.
89 lines
2.3 KiB
Plaintext
89 lines
2.3 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2002 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSG_NODECALLBACK
|
|
#define OSG_NODECALLBACK 1
|
|
|
|
#include <osg/Object>
|
|
#include <osg/ref_ptr>
|
|
|
|
namespace osg {
|
|
|
|
class Node;
|
|
class NodeVisitor;
|
|
|
|
class SG_EXPORT NodeCallback : public virtual Object {
|
|
|
|
public :
|
|
|
|
|
|
NodeCallback(){}
|
|
|
|
NodeCallback(const NodeCallback&,const CopyOp&):
|
|
_nestedCallback(_nestedCallback) {}
|
|
|
|
|
|
META_Object(osg,NodeCallback)
|
|
|
|
|
|
/** Callback method call by the NodeVisitor when visiting a node.*/
|
|
virtual void operator()(Node* node, NodeVisitor* nv)
|
|
{
|
|
// note, callback is repsonsible for scenegraph traversal so
|
|
// should always include call the traverse(node,nv) to ensure
|
|
// that the rest of cullbacks and the scene graph are traversed.
|
|
traverse(node,nv);
|
|
}
|
|
|
|
/** Call any nested callbacks and then traverse the scene graph. */
|
|
void traverse(Node* node,NodeVisitor* nv);
|
|
|
|
void setNestedCallback(NodeCallback* nc) { _nestedCallback = nc; }
|
|
NodeCallback* getNestedCallback() { return _nestedCallback.get(); }
|
|
|
|
inline void addNestedCallback(NodeCallback* nc)
|
|
{
|
|
if (nc)
|
|
{
|
|
if (_nestedCallback.valid())
|
|
{
|
|
nc->addNestedCallback(_nestedCallback.get());
|
|
_nestedCallback = nc;
|
|
}
|
|
else
|
|
{
|
|
_nestedCallback = nc;
|
|
}
|
|
}
|
|
}
|
|
|
|
inline void removeNestedCallback(NodeCallback* nc)
|
|
{
|
|
if (nc)
|
|
{
|
|
if (_nestedCallback==nc)
|
|
{
|
|
_nestedCallback = _nestedCallback->getNestedCallback();
|
|
}
|
|
else if (_nestedCallback.valid())
|
|
{
|
|
_nestedCallback->removeNestedCallback(nc);
|
|
}
|
|
}
|
|
}
|
|
|
|
public:
|
|
|
|
ref_ptr<NodeCallback> _nestedCallback;
|
|
|
|
protected:
|
|
|
|
virtual ~NodeCallback() {}
|
|
};
|
|
|
|
} // namespace
|
|
|
|
#endif
|
|
|