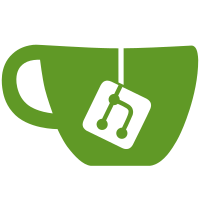
include change the CameraManipulators so they work with double for time instead of float. Also added support for DataType to osg::StateAttribute and StateSet so that they can be set to either STATIC or DYNAMIC, this allows the optimizer to know whether that an attribute can be optimized or not.
90 lines
2.2 KiB
Plaintext
90 lines
2.2 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGUTIL_GUIEVENTADAPTER
|
|
#define OSGUTIL_GUIEVENTADAPTER 1
|
|
|
|
#include <osg/Referenced>
|
|
#include <osgUtil/Export>
|
|
|
|
namespace osgUtil{
|
|
|
|
|
|
/** Pure virtual base class for adapting platform specific events into
|
|
* generic keyboard and mouse events.
|
|
*
|
|
* Used as GUI toolkit independent input into the osgUtil::CameraManipualor's.
|
|
* For an example of how GUIEventAdapter is specialised for a particular GUI
|
|
* Toolkit see osgGLUT::GLUTEventAdapter.
|
|
*/
|
|
class GUIEventAdapter : public osg::Referenced
|
|
{
|
|
public:
|
|
|
|
GUIEventAdapter() {}
|
|
|
|
|
|
enum MouseButtonMask {
|
|
LEFT_MOUSE_BUTTON=1,
|
|
MIDDLE_MOUSE_BUTTON=2,
|
|
RIGHT_MOUSE_BUTTON=4
|
|
};
|
|
|
|
enum EventType {
|
|
PUSH,
|
|
RELEASE,
|
|
DRAG,
|
|
MOVE,
|
|
KEYBOARD,
|
|
FRAME,
|
|
RESIZE,
|
|
NONE
|
|
};
|
|
|
|
/** Get the EventType of the GUI event.*/
|
|
virtual EventType getEventType() const = 0;
|
|
|
|
/** key pressed, return -1 if inappropriate for this event. */
|
|
virtual int getKey() const = 0;
|
|
|
|
/** button pressed/released, return -1 if inappropriate for this event.*/
|
|
virtual int getButton() const = 0;
|
|
|
|
/** window minimum x. */
|
|
virtual int getXmin() const = 0;
|
|
|
|
/** window maximum x. */
|
|
virtual int getXmax() const = 0;
|
|
|
|
/** window minimum y. */
|
|
virtual int getYmin() const = 0;
|
|
|
|
/** window maximum y. */
|
|
virtual int getYmax() const = 0;
|
|
|
|
/** current mouse x position.*/
|
|
virtual int getX() const = 0;
|
|
|
|
/** current mouse y position.*/
|
|
virtual int getY() const = 0;
|
|
|
|
/** current mouse button state */
|
|
virtual unsigned int getButtonMask() const = 0;
|
|
|
|
/** time in seconds of event. */
|
|
virtual double time() const = 0;
|
|
|
|
|
|
protected:
|
|
|
|
/** Force users to create on heap, so that multiple referencing is safe.*/
|
|
virtual ~GUIEventAdapter() {}
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|
|
|