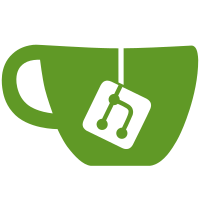
the functionality clear given the name. This will break user code unfortunately so please be away of the following mapping. osg::Matrix::makeTrans(..)?\026 -> osg::Matrix::makeTranslate(..) osg::Matrix::makeRot(..)?\026 -> osg::Matrix::makeRotate(..) osg::Matrix::trans(..)?\026 -> osg::Matrix::translate(..) osg::Quat::makeRot(..)?\026 -> osg::Quat::makeRotate(..) Also updated the rest of the OSG distribution to use the new names, and have removed the old deprecated Matrix methods too.
52 lines
1.5 KiB
C++
52 lines
1.5 KiB
C++
#include <osg/Transform>
|
|
|
|
#include <osgUtil/TransformCallback>
|
|
|
|
using namespace osgUtil;
|
|
|
|
TransformCallback::TransformCallback(const osg::Vec3& pivot,const osg::Vec3& axis,float angularVelocity)
|
|
{
|
|
_pivot = pivot;
|
|
_axis = axis;
|
|
_angular_velocity = angularVelocity;
|
|
|
|
_previousTraversalNumber = -1;
|
|
_previousTime = -1.0;
|
|
}
|
|
|
|
void TransformCallback::operator() (osg::Node* node, osg::NodeVisitor* nv)
|
|
{
|
|
osg::Transform* transform = dynamic_cast<osg::Transform*>(node);
|
|
if (nv && transform)
|
|
{
|
|
|
|
const osg::FrameStamp* fs = nv->getFrameStamp();
|
|
if (!fs) return; // not frame stamp, no handle on the time so can't move.
|
|
|
|
|
|
// ensure that we do not operate on this node more than
|
|
// once during this traversal. This is an issue since node
|
|
// can be shared between multiple parents.
|
|
if (nv->getTraversalNumber()!=_previousTraversalNumber)
|
|
{
|
|
double newTime = fs->getReferenceTime();
|
|
float delta_angle = _angular_velocity*(newTime-_previousTime);
|
|
|
|
osg::Matrix mat = osg::Matrix::translate(-_pivot)*
|
|
osg::Matrix::rotate(delta_angle,_axis)*
|
|
osg::Matrix::translate(_pivot);
|
|
|
|
|
|
// update the specified transform
|
|
transform->preMult(mat);
|
|
|
|
_previousTraversalNumber = nv->getTraversalNumber();
|
|
_previousTime = newTime;
|
|
}
|
|
}
|
|
|
|
// must call any nested node callbacks and continue subgraph traversal.
|
|
traverse(node,nv);
|
|
|
|
}
|