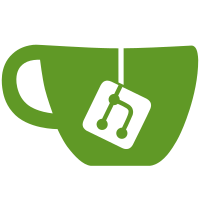
mode by using a _texParamtersDirty flag in combination with an applyTexParamters(State&) method which does the paramters setting in one tidy bundle. This new implementations replaces the CompileFlags submitted yesterday. Simplified NodeCallback by remove osg::NodeCallback::Requirements as they are no longer needed. Fixed comments in Drawable. Put guards around cosf definations so that they are only used under Win32/Mac. Fixed warning in CullVisitor.
75 lines
1.9 KiB
Plaintext
75 lines
1.9 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSG_NODECALLBACK
|
|
#define OSG_NODECALLBACK 1
|
|
|
|
#include <osg/Referenced>
|
|
|
|
namespace osg {
|
|
|
|
class Node;
|
|
class NodeVisitor;
|
|
|
|
class SG_EXPORT NodeCallback : public Referenced {
|
|
|
|
public :
|
|
|
|
|
|
NodeCallback(){}
|
|
virtual ~NodeCallback() {}
|
|
|
|
|
|
/** Callback method call by the NodeVisitor when visiting a node.*/
|
|
virtual void operator()(Node*, NodeVisitor*) {}
|
|
|
|
/** Call any nested callbacks and then traverse the scene graph. */
|
|
void traverse(Node* node,NodeVisitor* nv);
|
|
|
|
void setNestedCallback(NodeCallback* nc) { _nestedCallback = nc; }
|
|
NodeCallback* getNestedCallback() { return _nestedCallback.get(); }
|
|
|
|
inline void addNestedCallback(NodeCallback* nc)
|
|
{
|
|
if (nc)
|
|
{
|
|
if (_nestedCallback.valid())
|
|
{
|
|
nc->addNestedCallback(_nestedCallback.get());
|
|
_nestedCallback = nc;
|
|
}
|
|
else
|
|
{
|
|
_nestedCallback = nc;
|
|
}
|
|
}
|
|
}
|
|
|
|
inline void removeNestedCallback(NodeCallback* nc)
|
|
{
|
|
if (nc)
|
|
{
|
|
if (_nestedCallback==nc)
|
|
{
|
|
NodeCallback* nested_nc = _nestedCallback->getNestedCallback();
|
|
if (nested_nc) _nestedCallback = nc;
|
|
else _nestedCallback = 0;
|
|
}
|
|
else
|
|
{
|
|
_nestedCallback->removeNestedCallback(nc);
|
|
}
|
|
}
|
|
}
|
|
|
|
public:
|
|
|
|
ref_ptr<NodeCallback> _nestedCallback;
|
|
};
|
|
|
|
} // namespace
|
|
|
|
#endif
|
|
|