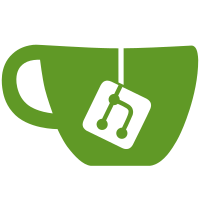
them more consistent with each other. This does mean an API change, so dependanct code in the OSG has been updated accordingly.
357 lines
13 KiB
Plaintext
357 lines
13 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2002 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSG_PRIMTIVE
|
|
#define OSG_PRIMTIVE 1
|
|
|
|
#include <osg/Drawable>
|
|
|
|
namespace osg {
|
|
|
|
#ifndef _MSC_VER
|
|
|
|
typedef std::vector<GLsizei> VectorSizei;
|
|
typedef std::vector<GLubyte> VectorUByte;
|
|
typedef std::vector<GLushort> VectorUShort;
|
|
typedef std::vector<GLuint> VectorUInt;
|
|
|
|
#else // _MSC_VER
|
|
|
|
// Following Vector wrapper classes are work arounds for MS linker problems with
|
|
// multiply implemented methods.
|
|
//
|
|
// An alternative, sent in by Clay Fowler, is workaround in VS to prevent the problem:
|
|
// the following changes have to be made to the project to make it compile,
|
|
// but NO changes are required to the actual source code:
|
|
// In the osgUtil project, go to the project properties, select the Linker/Command Line property page,
|
|
// and add the following switch in the "Additional Options" field:
|
|
// FORCE:MULTIPLE
|
|
|
|
class VectorSizei: public std::vector<GLsizei> {
|
|
typedef std::vector<value_type> inherited;
|
|
public:
|
|
VectorSizei(): inherited() {}
|
|
explicit VectorSizei(size_type n): inherited(n) {}
|
|
VectorSizei(const VectorSizei ©): inherited(copy) {}
|
|
//VectorSizei(value_type *beg_, value_type *end_): inherited(beg_, end_) {}
|
|
template<class InputIterator>
|
|
VectorSizei(InputIterator beg_, InputIterator end_): inherited(beg_, end_) {}
|
|
};
|
|
|
|
class VectorUByte: public std::vector<GLubyte> {
|
|
typedef std::vector<value_type> inherited;
|
|
public:
|
|
VectorUByte(): inherited() {}
|
|
explicit VectorUByte(size_type n): inherited(n) {}
|
|
VectorUByte(const VectorUByte ©): inherited(copy) {}
|
|
//VectorUByte(value_type *beg_, value_type *end_): inherited(beg_, end_) {}
|
|
template<class InputIterator>
|
|
VectorUByte(InputIterator beg_, InputIterator end_): inherited(beg_, end_) {}
|
|
};
|
|
|
|
class VectorUShort: public std::vector<GLushort> {
|
|
typedef std::vector<value_type> inherited;
|
|
public:
|
|
VectorUShort(): inherited() {}
|
|
explicit VectorUShort(size_type n): inherited(n) {}
|
|
VectorUShort(const VectorUShort ©): inherited(copy) {}
|
|
//VectorUShort(value_type *beg_, value_type *end_): inherited(beg_, end_) {}
|
|
template<class InputIterator>
|
|
VectorUShort(InputIterator beg_, InputIterator end_): inherited(beg_, end_) {}
|
|
};
|
|
|
|
class VectorUInt: public std::vector<GLuint> {
|
|
typedef std::vector<value_type> inherited;
|
|
public:
|
|
VectorUInt(): inherited() {}
|
|
explicit VectorUInt(size_type n): inherited(n) {}
|
|
VectorUInt(const VectorUInt ©): inherited(copy) {}
|
|
//VectorUInt(value_type *beg_, value_type *end_): inherited(beg_, end_) {}
|
|
template<class InputIterator>
|
|
VectorUInt(InputIterator beg_, InputIterator end_): inherited(beg_, end_) {}
|
|
};
|
|
|
|
#endif
|
|
|
|
|
|
class Primitive : public Object
|
|
{
|
|
public:
|
|
|
|
enum Type
|
|
{
|
|
PrimitiveType,
|
|
DrawArraysPrimitiveType,
|
|
DrawArrayLengthsPrimitiveType,
|
|
DrawElementsUBytePrimitiveType,
|
|
DrawElementsUShortPrimitiveType,
|
|
DrawElementsUIntPrimitiveType
|
|
};
|
|
|
|
enum Mode
|
|
{
|
|
POINTS = GL_POINTS,
|
|
LINES = GL_LINES,
|
|
LINE_STRIP = GL_LINE_STRIP,
|
|
LINE_LOOP = GL_LINE_LOOP,
|
|
TRIANGLES = GL_TRIANGLES,
|
|
TRIANGLE_STRIP = GL_TRIANGLE_STRIP,
|
|
TRIANGLE_FAN = GL_TRIANGLE_FAN,
|
|
QUADS = GL_QUADS,
|
|
QUAD_STRIP = GL_QUAD_STRIP,
|
|
POLYGON = GL_POLYGON
|
|
};
|
|
|
|
Primitive(Type primType=PrimitiveType,GLenum mode=0):
|
|
_primitiveType(primType),
|
|
_mode(mode) {}
|
|
|
|
Primitive(const Primitive& prim,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Object(prim,copyop),
|
|
_primitiveType(prim._primitiveType),
|
|
_mode(prim._mode) {}
|
|
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const Primitive*>(obj)!=NULL; }
|
|
virtual const char* libraryName() const { return "osg"; }
|
|
virtual const char* className() const { return "Primitve"; }
|
|
|
|
Type getType() const { return _primitiveType; }
|
|
|
|
void setMode(GLenum mode) { _mode = mode; }
|
|
GLenum getMode() const { return _mode; }
|
|
|
|
virtual void draw() const = 0;
|
|
|
|
virtual void accept(Drawable::PrimitiveFunctor&) {}
|
|
|
|
virtual void offsetIndices(int offset) = 0;
|
|
|
|
protected:
|
|
|
|
Type _primitiveType;
|
|
GLenum _mode;
|
|
};
|
|
|
|
class SG_EXPORT DrawArrays : public Primitive
|
|
{
|
|
public:
|
|
|
|
DrawArrays(GLenum mode=0):
|
|
Primitive(DrawArraysPrimitiveType,mode),
|
|
_first(0),
|
|
_count(0) {}
|
|
|
|
DrawArrays(GLenum mode, GLint first, GLsizei count):
|
|
Primitive(DrawArraysPrimitiveType,mode),
|
|
_first(first),
|
|
_count(count) {}
|
|
|
|
DrawArrays(const DrawArrays& da,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Primitive(da,copyop),
|
|
_first(da._first),
|
|
_count(da._count) {}
|
|
|
|
virtual Object* cloneType() const { return osgNew DrawArrays(); }
|
|
virtual Object* clone(const CopyOp& copyop) const { return osgNew DrawArrays(*this,copyop); }
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const DrawArrays*>(obj)!=NULL; }
|
|
virtual const char* libraryName() const { return "osg"; }
|
|
virtual const char* className() const { return "DrawArrays"; }
|
|
|
|
|
|
void set(GLenum mode,GLint first, GLsizei count)
|
|
{
|
|
_mode = mode;
|
|
_first = first;
|
|
_count = count;
|
|
}
|
|
|
|
void setFirst(GLint first) { _first = first; }
|
|
GLint getFirst() const { return _first; }
|
|
|
|
void setCount(GLsizei count) { _count = count; }
|
|
GLsizei getCount() const { return _count; }
|
|
|
|
virtual void draw() const;
|
|
|
|
virtual void accept(Drawable::PrimitiveFunctor& functor);
|
|
|
|
virtual void offsetIndices(int offset) { _first += offset; }
|
|
|
|
protected:
|
|
|
|
GLint _first;
|
|
GLsizei _count;
|
|
};
|
|
|
|
class SG_EXPORT DrawArrayLengths : public Primitive, public VectorSizei
|
|
{
|
|
public:
|
|
|
|
DrawArrayLengths(GLenum mode=0):
|
|
Primitive(DrawArrayLengthsPrimitiveType,mode),
|
|
_first(0) {}
|
|
|
|
DrawArrayLengths(const DrawArrayLengths& dal,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Primitive(dal,copyop),
|
|
VectorSizei(dal),
|
|
_first(dal._first) {}
|
|
|
|
DrawArrayLengths(GLenum mode, GLint first, unsigned int no, GLsizei* ptr) :
|
|
Primitive(DrawArrayLengthsPrimitiveType,mode),
|
|
VectorSizei(ptr,ptr+no),
|
|
_first(first) {}
|
|
|
|
DrawArrayLengths(GLenum mode,GLint first, unsigned int no) :
|
|
Primitive(DrawArrayLengthsPrimitiveType,mode),
|
|
VectorSizei(no),
|
|
_first(first) {}
|
|
|
|
template <class InputIterator>
|
|
DrawArrayLengths(GLenum mode, GLint first, InputIterator firstItr,InputIterator lastItr) :
|
|
Primitive(DrawArrayLengthsPrimitiveType,mode),
|
|
VectorSizei(firstItr,lastItr),
|
|
_first(first) {}
|
|
|
|
virtual Object* cloneType() const { return osgNew DrawArrayLengths(); }
|
|
virtual Object* clone(const CopyOp& copyop) const { return osgNew DrawArrayLengths(*this,copyop); }
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const DrawArrayLengths*>(obj)!=NULL; }
|
|
virtual const char* libraryName() const { return "osg"; }
|
|
virtual const char* className() const { return "DrawArrayLengths"; }
|
|
|
|
|
|
void setFirst(GLint first) { _first = first; }
|
|
GLint getFirst() const { return _first; }
|
|
|
|
virtual void draw() const;
|
|
|
|
virtual void accept(Drawable::PrimitiveFunctor& functor);
|
|
|
|
virtual void offsetIndices(int offset) { _first += offset; }
|
|
|
|
protected:
|
|
|
|
GLint _first;
|
|
};
|
|
|
|
class SG_EXPORT DrawElementsUByte : public Primitive, public VectorUByte
|
|
{
|
|
public:
|
|
|
|
DrawElementsUByte(GLenum mode=0):
|
|
Primitive(DrawElementsUBytePrimitiveType,mode) {}
|
|
|
|
DrawElementsUByte(const DrawElementsUByte& array,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Primitive(array,copyop),
|
|
VectorUByte(array) {}
|
|
|
|
DrawElementsUByte(GLenum mode,unsigned int no,GLubyte* ptr) :
|
|
Primitive(DrawElementsUBytePrimitiveType,mode),
|
|
VectorUByte(ptr,ptr+no) {}
|
|
|
|
DrawElementsUByte(GLenum mode,unsigned int no) :
|
|
Primitive(DrawElementsUBytePrimitiveType,mode),
|
|
VectorUByte(no) {}
|
|
|
|
template <class InputIterator>
|
|
DrawElementsUByte(GLenum mode, InputIterator first,InputIterator last) :
|
|
Primitive(DrawElementsUBytePrimitiveType,mode),
|
|
VectorUByte(first,last) {}
|
|
|
|
virtual Object* cloneType() const { return osgNew DrawElementsUByte(); }
|
|
virtual Object* clone(const CopyOp& copyop) const { return osgNew DrawElementsUByte(*this,copyop); }
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const DrawElementsUByte*>(obj)!=NULL; }
|
|
virtual const char* libraryName() const { return "osg"; }
|
|
virtual const char* className() const { return "DrawElementsUByte"; }
|
|
|
|
virtual void draw() const ;
|
|
|
|
virtual void accept(Drawable::PrimitiveFunctor& functor);
|
|
|
|
virtual void offsetIndices(int offset);
|
|
};
|
|
|
|
|
|
class SG_EXPORT DrawElementsUShort : public Primitive, public VectorUShort
|
|
{
|
|
public:
|
|
|
|
DrawElementsUShort(GLenum mode=0):
|
|
Primitive(DrawElementsUShortPrimitiveType,mode) {}
|
|
|
|
DrawElementsUShort(const DrawElementsUShort& array,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Primitive(array,copyop),
|
|
VectorUShort(array) {}
|
|
|
|
DrawElementsUShort(GLenum mode,unsigned int no,GLushort* ptr) :
|
|
Primitive(DrawElementsUShortPrimitiveType,mode),
|
|
VectorUShort(ptr,ptr+no) {}
|
|
|
|
DrawElementsUShort(GLenum mode,unsigned int no) :
|
|
Primitive(DrawElementsUShortPrimitiveType,mode),
|
|
VectorUShort(no) {}
|
|
|
|
template <class InputIterator>
|
|
DrawElementsUShort(GLenum mode, InputIterator first,InputIterator last) :
|
|
Primitive(DrawElementsUShortPrimitiveType,mode),
|
|
VectorUShort(first,last) {}
|
|
|
|
virtual Object* cloneType() const { return osgNew DrawElementsUShort(); }
|
|
virtual Object* clone(const CopyOp& copyop) const { return osgNew DrawElementsUShort(*this,copyop); }
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const DrawElementsUShort*>(obj)!=NULL; }
|
|
virtual const char* libraryName() const { return "osg"; }
|
|
virtual const char* className() const { return "DrawElementsUShort"; }
|
|
|
|
virtual void draw() const;
|
|
|
|
virtual void accept(Drawable::PrimitiveFunctor& functor);
|
|
|
|
virtual void offsetIndices(int offset);
|
|
};
|
|
|
|
class SG_EXPORT DrawElementsUInt : public Primitive, public VectorUInt
|
|
{
|
|
public:
|
|
|
|
DrawElementsUInt(GLenum mode=0):
|
|
Primitive(DrawElementsUIntPrimitiveType,mode) {}
|
|
|
|
DrawElementsUInt(const DrawElementsUInt& array,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Primitive(array,copyop),
|
|
VectorUInt(array) {}
|
|
|
|
DrawElementsUInt(GLenum mode,unsigned int no,GLuint* ptr) :
|
|
Primitive(DrawElementsUIntPrimitiveType,mode),
|
|
VectorUInt(ptr,ptr+no) {}
|
|
|
|
DrawElementsUInt(GLenum mode,unsigned int no) :
|
|
Primitive(DrawElementsUIntPrimitiveType,mode),
|
|
VectorUInt(no) {}
|
|
|
|
template <class InputIterator>
|
|
DrawElementsUInt(GLenum mode, InputIterator first,InputIterator last) :
|
|
Primitive(DrawElementsUIntPrimitiveType,mode),
|
|
VectorUInt(first,last) {}
|
|
|
|
virtual Object* cloneType() const { return osgNew DrawElementsUInt(); }
|
|
virtual Object* clone(const CopyOp& copyop) const { return osgNew DrawElementsUInt(*this,copyop); }
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const DrawElementsUInt*>(obj)!=NULL; }
|
|
virtual const char* libraryName() const { return "osg"; }
|
|
virtual const char* className() const { return "DrawElementsUInt"; }
|
|
|
|
virtual void draw() const;
|
|
|
|
virtual void accept(Drawable::PrimitiveFunctor& functor);
|
|
|
|
virtual void offsetIndices(int offset);
|
|
};
|
|
|
|
// backwards compatibility with first incarnation of DrawElements nameing convention.
|
|
typedef DrawElementsUByte UByteDrawElements;
|
|
typedef DrawElementsUShort UShortDrawElements;
|
|
typedef DrawElementsUInt UIntDrawElements;
|
|
|
|
}
|
|
|
|
#endif
|