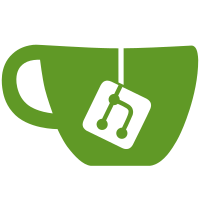
loader, still need to fill in the saving and loading of paramters. Went through the distribution remove old API usage.
139 lines
4.6 KiB
Plaintext
139 lines
4.6 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2002 Robert Osfield
|
|
//created by Sasa Bistrovic
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#if !defined(OSG_DOFTRANSFORM)
|
|
#define OSG_DOFTRANSFORM
|
|
|
|
//base class:
|
|
#include <osg/MatrixTransform>
|
|
|
|
namespace osg{
|
|
/** DOFTransform - encapsulates Multigen DOF behavior*/
|
|
class SG_EXPORT DOFTransform : public Transform
|
|
{
|
|
public:
|
|
/** constructor*/
|
|
DOFTransform();
|
|
|
|
/**copy constructor*/
|
|
DOFTransform(const DOFTransform& dof, const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Transform(dof, copyop),
|
|
_minHPR(dof._minHPR),
|
|
_maxHPR(dof._maxHPR),
|
|
_currentHPR(dof._currentHPR),
|
|
_incrementHPR(dof._incrementHPR),
|
|
_minTranslate(dof._minTranslate),
|
|
_maxTranslate(dof._maxTranslate),
|
|
_currentTranslate(dof._currentTranslate),
|
|
_incrementTranslate(dof._incrementTranslate),
|
|
_minScale(dof._minScale),
|
|
_maxScale(dof._maxScale),
|
|
_currentScale(dof._currentScale),
|
|
_incrementScale(dof._incrementScale),
|
|
_Put(dof._Put),
|
|
_inversePut(dof._inversePut),
|
|
_limitationFlags(dof._limitationFlags),
|
|
_animationOn(dof._animationOn),
|
|
_increasingFlags(dof._increasingFlags) {}
|
|
|
|
META_Node(osg, DOFTransform);
|
|
|
|
void loadMinHPR(const Vec3& hpr) {_minHPR = hpr;}
|
|
void loadMaxHPR(const Vec3& hpr) {_maxHPR = hpr;}
|
|
void loadCurrentHPR(const Vec3& hpr) {_currentHPR = hpr;}
|
|
void loadIncrementHPR(const Vec3& hpr) {_incrementHPR = hpr;}
|
|
|
|
void setCurrentHPR(const Vec3& hpr);
|
|
|
|
void loadMinTranslate(const Vec3& translate) {_minTranslate = translate;}
|
|
void loadMaxTranslate(const Vec3& translate) {_maxTranslate = translate;}
|
|
void loadCurrentTranslate(const Vec3& translate){_currentTranslate = translate;}
|
|
void loadIncrementTranslate(const Vec3& translate) {_incrementTranslate = translate;}
|
|
|
|
void setCurrentTranslate(const Vec3& translate);
|
|
|
|
void loadMinScale(const Vec3& scale) {_minScale = scale;}
|
|
void loadMaxScale(const Vec3& scale) {_maxScale = scale;}
|
|
void loadCurrentScale(const Vec3& scale) {_currentScale = scale;}
|
|
void loadIncrementScale(const Vec3& scale) {_incrementScale = scale;}
|
|
|
|
void setCurrentScale(const Vec3& scale);
|
|
|
|
void setPutMatrix(const Matrix& put) {_Put = put;}
|
|
void setInversePutMatrix(const Matrix& inversePut) {_inversePut = inversePut;}
|
|
|
|
void setLimitationFlags(const unsigned long flags) {_limitationFlags = flags;}
|
|
|
|
inline const Vec3& getCurrentHPR() const {return _currentHPR;}
|
|
inline const Vec3& getCurrentTranslate() const {return _currentTranslate;}
|
|
inline const Vec3& getCurrentScale() const {return _currentScale;}
|
|
|
|
inline const Matrix& getPutMatrix() const {return _Put;}
|
|
inline const Matrix& getInversePutMatrix() const {return _inversePut;}
|
|
|
|
inline const unsigned long getLimitationFlags() const {return _limitationFlags;}
|
|
|
|
inline void setAnimationOn(bool do_animate) {_animationOn = do_animate;}
|
|
inline const bool animationOn() const {return _animationOn;}
|
|
|
|
// inline const bool increasing() const {return _increasing;}
|
|
|
|
void animate();
|
|
|
|
protected:
|
|
virtual ~DOFTransform() {}
|
|
|
|
Vec3 _minHPR;
|
|
Vec3 _maxHPR;
|
|
Vec3 _currentHPR;
|
|
Vec3 _incrementHPR;
|
|
|
|
Vec3 _minTranslate;
|
|
Vec3 _maxTranslate;
|
|
Vec3 _currentTranslate;
|
|
Vec3 _incrementTranslate;
|
|
|
|
Vec3 _minScale;
|
|
Vec3 _maxScale;
|
|
Vec3 _currentScale;
|
|
Vec3 _incrementScale;
|
|
|
|
Matrix _Put;
|
|
Matrix _inversePut;
|
|
|
|
unsigned long _limitationFlags;
|
|
/* bits from left to right
|
|
0 = x translation limited (2^31)
|
|
1 = y translation limited (2^30)
|
|
2 = z translation limited (2^29)
|
|
3 = pitch limited (2^28)
|
|
4 = roll limited (2^27)
|
|
5 = yaw limited (2^26)
|
|
6 = x scale limited (2^25)
|
|
7 = y scale limited (2^24)
|
|
8 = z scale limited (2^23)
|
|
|
|
else reserved
|
|
*/
|
|
|
|
bool _animationOn;
|
|
/** flags indicating whether value is incerasing or decreasing in animation
|
|
bits form right to left, 1 means increasing while 0 is decreasing
|
|
0 = x translation
|
|
1 = y translation
|
|
2 = z translation
|
|
3 = pitch
|
|
4 = roll
|
|
5 = yaw
|
|
6 = x scale
|
|
7 = y scale
|
|
8 = z scale
|
|
*/
|
|
unsigned short _increasingFlags;
|
|
};
|
|
|
|
}
|
|
#endif
|