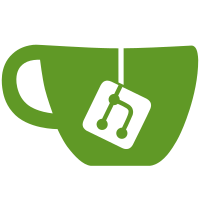
Changes: - new mouse wheel zoom/movement/center functionality - ability to fix vertical axis (important for CAD) - possibility to specify values as absolute values or relative to model size - kind of backward compatibility by flags passed to constructor - and much more - restructuring classes to use kind of hierarchy and standard way of event processing (handle methods). This way, there is much more code reusability and it is more easy to develop new kinds of manipulators. Briefly, the new architecture keeps MatrixManipulator as base abstract class. StandardManipulator is the feature-rich standard manipulator with two main descendant classes: OrbitManipulator and FirstPersonManipulator. OrbitManipulator is base class for all trackball style manipulators, based on center, rotation and distance from center. FirstPersonManipulator is base for walk or fly style manipulators, using position and rotation for camera manipulation. " Changes by Robert: Replaced osg::Vec3 by osg::Vec3d, introduced DEFAULT_SETTINGS enum and usage. Added frame time member variables in prep for improving throw animation when vysync is off.
83 lines
3.1 KiB
C++
83 lines
3.1 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2006 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGGA_FLIGHT_MANIPULATOR
|
|
#define OSGGA_FLIGHT_MANIPULATOR 1
|
|
|
|
#include <osgGA/FirstPersonManipulator>
|
|
|
|
|
|
namespace osgGA {
|
|
|
|
|
|
/** FlightManipulator is a MatrixManipulator which provides flight simulator-like
|
|
* updating of the camera position & orientation. By default, the left mouse
|
|
* button accelerates, the right mouse button decelerates, and the middle mouse
|
|
* button (or left and right simultaneously) stops dead.
|
|
*/
|
|
class OSGGA_EXPORT FlightManipulator : public FirstPersonManipulator
|
|
{
|
|
typedef FirstPersonManipulator inherited;
|
|
|
|
public:
|
|
|
|
enum YawControlMode {
|
|
YAW_AUTOMATICALLY_WHEN_BANKED,
|
|
NO_AUTOMATIC_YAW
|
|
};
|
|
|
|
FlightManipulator( int flags = UPDATE_MODEL_SIZE | COMPUTE_HOME_USING_BBOX );
|
|
FlightManipulator( const FlightManipulator& fpm,
|
|
const osg::CopyOp& copyOp = osg::CopyOp::SHALLOW_COPY );
|
|
|
|
META_Object( osgGA, FlightManipulator );
|
|
|
|
virtual void setYawControlMode( YawControlMode ycm );
|
|
inline YawControlMode getYawControlMode() const;
|
|
|
|
virtual void home( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual void init( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual void getUsage( osg::ApplicationUsage& usage ) const;
|
|
|
|
protected:
|
|
|
|
virtual bool handleFrame( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual bool handleMouseMove( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual bool handleMouseDrag( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual bool handleMousePush( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual bool handleMouseRelease( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual bool handleKeyDown( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
virtual bool flightHandleEvent( const osgGA::GUIEventAdapter& ea, osgGA::GUIActionAdapter& us );
|
|
|
|
virtual bool performMovement();
|
|
virtual bool performMovementLeftMouseButton( const double dt, const double dx, const double dy );
|
|
virtual bool performMovementMiddleMouseButton( const double dt, const double dx, const double dy );
|
|
virtual bool performMovementRightMouseButton( const double dt, const double dx, const double dy );
|
|
|
|
YawControlMode _yawMode;
|
|
|
|
};
|
|
|
|
|
|
//
|
|
// inline methods
|
|
//
|
|
|
|
/// Returns the Yaw control for the flight model.
|
|
inline FlightManipulator::YawControlMode FlightManipulator::getYawControlMode() const { return _yawMode; }
|
|
|
|
|
|
}
|
|
|
|
#endif /* OSGGA_FLIGHT_MANIPULATOR */
|