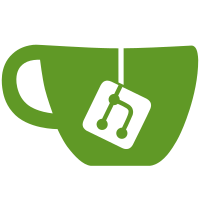
be a StateAttribute. This allows alternative implementations of Lights other than the standard osg::Light.
56 lines
1.5 KiB
Plaintext
56 lines
1.5 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSG_LIGHTSOURCE
|
|
#define OSG_LIGHTSOURCE 1
|
|
|
|
#include <osg/NodeVisitor>
|
|
#include <osg/Light>
|
|
#include <osg/Group>
|
|
|
|
namespace osg {
|
|
|
|
/** Leaf Node for defining a light in the scene.*/
|
|
class SG_EXPORT LightSource : public Group
|
|
{
|
|
public:
|
|
|
|
LightSource();
|
|
|
|
LightSource(const LightSource& ls, const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
Group(ls,copyop),
|
|
_value(ls._value),
|
|
_light(dynamic_cast<osg::Light*>(copyop(ls._light.get()))) {}
|
|
|
|
META_Node(osg, LightSource);
|
|
|
|
/** Set the attached light.*/
|
|
void setLight(StateAttribute* light);
|
|
|
|
/** Get the attached light.*/
|
|
inline StateAttribute* getLight() { return _light.get(); }
|
|
|
|
/** Get the const attached light.*/
|
|
inline const StateAttribute* getLight() const { return _light.get(); }
|
|
|
|
/** Set the GLModes on StateSet associated with the LightSource.*/
|
|
void setStateSetModes(StateSet&,const StateAttribute::GLModeValue) const;
|
|
|
|
/** Set up the local StateSet */
|
|
void setLocalStateSetModes(const StateAttribute::GLModeValue=StateAttribute::ON);
|
|
|
|
protected:
|
|
|
|
virtual ~LightSource();
|
|
|
|
virtual const bool computeBound() const;
|
|
|
|
StateAttribute::GLModeValue _value;
|
|
ref_ptr<StateAttribute> _light;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|