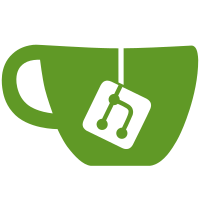
Fix to the update of the error metrics on the edges in the simplification mesh that are affected by an edge collapse. Addition of 'n' and 'p' keyboard control in osgsimplifier example to allow users to control the sample ratio manually.
104 lines
4.0 KiB
C++
104 lines
4.0 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2005 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGUTIL_SIMPLIFIER
|
|
#define OSGUTIL_SIMPLIFIER 1
|
|
|
|
#include <osg/NodeVisitor>
|
|
#include <osg/Geode>
|
|
#include <osg/Geometry>
|
|
|
|
#include <osgUtil/Export>
|
|
|
|
namespace osgUtil {
|
|
|
|
/** A simplifier for reducing the number of traingles in osg::Geometry.
|
|
*/
|
|
class OSGUTIL_EXPORT Simplifier : public osg::NodeVisitor
|
|
{
|
|
public:
|
|
|
|
Simplifier(float sampleRatio=1.0f, float maximumError=0.0f);
|
|
|
|
|
|
void setSampleRatio(float sampleRatio) { _sampleRatio = sampleRatio; }
|
|
float getSampleRatio() const { return _sampleRatio; }
|
|
|
|
void setMaximumError(float error) { _maximumError = error; }
|
|
float getMaximumError() const { return _maximumError; }
|
|
|
|
class ContinueSimplificationCallback : public osg::Referenced
|
|
{
|
|
public:
|
|
/** return true if mesh should be continued to be simplified, return false to stop simplification.*/
|
|
virtual bool continueSimplification(const Simplifier& simplifier, float nextError, unsigned int numOriginalPrimitives, unsigned int numRemainingPrimitives) const
|
|
{
|
|
return simplifier.continueSimplificationImplementation(nextError, numOriginalPrimitives, numRemainingPrimitives);
|
|
}
|
|
|
|
protected:
|
|
virtual ~ContinueSimplificationCallback() {}
|
|
};
|
|
|
|
void setContinueSimplificationCallback(ContinueSimplificationCallback* cb) { _continueSimplificationCallback = cb; }
|
|
ContinueSimplificationCallback* getContinueSimplificationCallback() { return _continueSimplificationCallback.get(); }
|
|
const ContinueSimplificationCallback* getContinueSimplificationCallback() const { return _continueSimplificationCallback.get(); }
|
|
|
|
|
|
bool continueSimplification(float nextError, unsigned int numOriginalPrimitives, unsigned int numRemainingPrimitives) const
|
|
{
|
|
if (_continueSimplificationCallback.valid()) return _continueSimplificationCallback->continueSimplification(*this, nextError, numOriginalPrimitives, numRemainingPrimitives);
|
|
else return continueSimplificationImplementation(nextError, numOriginalPrimitives, numRemainingPrimitives);
|
|
}
|
|
|
|
virtual bool continueSimplificationImplementation(float nextError, unsigned int numOriginalPrimitives, unsigned int numRemainingPrimitives) const
|
|
{
|
|
if (nextError<=getMaximumError()) return true;
|
|
return (float)numRemainingPrimitives > (float)numOriginalPrimitives * getSampleRatio();
|
|
}
|
|
|
|
|
|
virtual void apply(osg::Geode& geode)
|
|
{
|
|
for(unsigned int i=0;i<geode.getNumDrawables();++i)
|
|
{
|
|
osg::Geometry* geometry = geode.getDrawable(i)->asGeometry();
|
|
if (geometry)
|
|
{
|
|
simplify(*geometry);
|
|
}
|
|
}
|
|
}
|
|
|
|
/** simply the geometry.*/
|
|
void simplify(osg::Geometry& geometry);
|
|
|
|
typedef std::vector<unsigned int> IndexList; /// a list of point indices
|
|
|
|
/** simply the geometry, whilst protecting key points from being modified.*/
|
|
void simplify(osg::Geometry& geometry, const IndexList& protectedPoints);
|
|
|
|
protected:
|
|
|
|
float _sampleRatio;
|
|
float _maximumError;
|
|
|
|
osg::ref_ptr<ContinueSimplificationCallback> _continueSimplificationCallback;
|
|
|
|
};
|
|
|
|
|
|
}
|
|
|
|
#endif
|