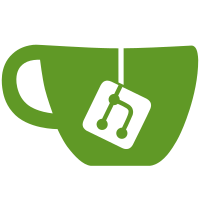
1. type converters created automatically by the I_BaseType macro use static_cast<> even for base-to-derived conversions. dynamic_cast<> should be used instead. 2. as a consequence of the above fix, I_BaseType must now differentiate between polymorphic and non-polymorphic base classes, because the latter can't be dynamic_cast'd to derived classes. Some template magic (see is_polymorphic<> in ReflectionMacros) is used to detect polymorphism at compile time (I'm NOT sure it works on all platforms as it's partly implementation-dependent. Please test!). 3. predefined custom property getters/setters/counters/etc. (as those defined for STL containers) only work on Value objects that contain non-pointer instances. This was an unwanted restriction that no longer exists. Wrappers will need to be recompiled. This is a good time to give them a fresh update with genwrapper. NOTE: fix #1 should get rid of those crashes and strange behaviours that some users noticed while using osgIntrospection through osgTcl or in their own code."
64 lines
2.1 KiB
C++
64 lines
2.1 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2005 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
//osgIntrospection - Copyright (C) 2005 Marco Jez
|
|
|
|
#ifndef OSGINTROSPECTION_UTILITY_
|
|
#define OSGINTROSPECTION_UTILITY_
|
|
|
|
#include <osgIntrospection/Export>
|
|
#include <osgIntrospection/Value>
|
|
#include <osgIntrospection/ParameterInfo>
|
|
#include <osgIntrospection/MethodInfo>
|
|
#include <osgIntrospection/variant_cast>
|
|
|
|
#include <vector>
|
|
|
|
namespace osgIntrospection
|
|
{
|
|
|
|
bool OSGINTROSPECTION_EXPORT areParametersCompatible(const ParameterInfoList& pl1, const ParameterInfoList& pl2);
|
|
bool OSGINTROSPECTION_EXPORT areArgumentsCompatible(const ValueList& vl, const ParameterInfoList& pl, float &match);
|
|
|
|
template<typename T>
|
|
void convertArgument(ValueList& src, ValueList& dest, const ParameterInfoList& pl, int index)
|
|
{
|
|
if (index >= static_cast<int>(src.size()))
|
|
{
|
|
dest[index] = pl[index]->getDefaultValue();
|
|
}
|
|
else
|
|
{
|
|
Value& sv = src[index];
|
|
if (requires_conversion<T>(sv))
|
|
dest[index] = sv.convertTo(pl[index]->getParameterType());
|
|
else
|
|
dest[index].swap(sv);
|
|
}
|
|
}
|
|
|
|
template<typename T> const T &getInstance(const Value &instance)
|
|
{
|
|
return instance.isTypedPointer() ?
|
|
*variant_cast<const T*>(instance) : variant_cast<const T&>(instance);
|
|
}
|
|
|
|
template<typename T> T &getInstance(Value &instance)
|
|
{
|
|
return instance.isTypedPointer() ?
|
|
*variant_cast<T*>(instance) : variant_cast<T&>(instance);
|
|
}
|
|
|
|
}
|
|
|
|
#endif
|