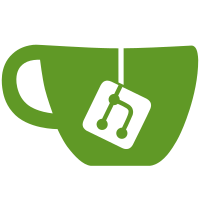
that a single class can be used for two different types of callbacks whithout incurring issues of multiple ref counts and ref()/unref() methods existing.
767 lines
29 KiB
Plaintext
767 lines
29 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2002 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSG_DRAWABLE
|
|
#define OSG_DRAWABLE 1
|
|
|
|
#include <osg/BoundingBox>
|
|
#include <osg/State>
|
|
#include <osg/Types>
|
|
#include <osg/NodeVisitor>
|
|
#include <osg/Shape>
|
|
#include <osg/buffered_value>
|
|
|
|
namespace osg {
|
|
|
|
class Vec2;
|
|
class Vec3;
|
|
class Vec4;
|
|
class UByte4;
|
|
class Geometry;
|
|
|
|
// this is define to alter the way display lists are compiled inside the
|
|
// the draw method, it has been found that the NVidia drivers fail completely
|
|
// to optimize COMPILE_AND_EXECUTE in fact make it go slower than for no display
|
|
// lists, but optimize a separate COMPILE very well?! Define it as default
|
|
// the use of a sperate COMPILE, then glCallList rather than use COMPILE_AND_EXECUTE.
|
|
|
|
#define USE_SEPARATE_COMPILE_AND_EXECUTE
|
|
|
|
/** Pure virtual base class for drawable Geometry. Contains no drawing primitives
|
|
directly, these are provided by subclasses such as osg::Geometry. State attributes
|
|
for a Drawable are maintained in StateSet which the Drawable maintains
|
|
a referenced counted pointer to. Both Drawable's and StateSet's can
|
|
be shared for optimal memory usage and graphics performance.
|
|
*/
|
|
class SG_EXPORT Drawable : public Object
|
|
{
|
|
public:
|
|
|
|
Drawable();
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
Drawable(const Drawable& drawable,const CopyOp& copyop=CopyOp::SHALLOW_COPY);
|
|
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const Drawable*>(obj)!=NULL; }
|
|
virtual const char* libraryName() const { return "osg"; }
|
|
virtual const char* className() const { return "Drawable"; }
|
|
|
|
/** convert 'this' into a Geometry pointer if Drawable is a Geometry, otherwise return 0.
|
|
* Equivalent to dynamic_cast<Geometry*>(this).*/
|
|
virtual Geometry* asGeometry() { return 0; }
|
|
/** convert 'const this' into a const Geometry pointer if Drawable is a Geometry, otherwise return 0.
|
|
* Equivalent to dynamic_cast<const Geometry*>(this).*/
|
|
virtual const Geometry* asGeometry() const { return 0; }
|
|
|
|
|
|
/** A vector of osg::Node pointers which is used to store the parent(s) of drawable.*/
|
|
typedef std::vector<Node*> ParentList;
|
|
|
|
/** Get the parent list of drawable. */
|
|
inline const ParentList& getParents() const { return _parents; }
|
|
|
|
/** Get the a copy of parent list of node. A copy is returned to
|
|
* prevent modification of the parent list.*/
|
|
inline ParentList getParents() { return _parents; }
|
|
|
|
/** Get a single parent of Drawable.
|
|
* @param i index of the parent to get.
|
|
* @return the parent i.
|
|
*/
|
|
inline Node* getParent(unsigned int i) { return _parents[i]; }
|
|
/** Get a single const parent of Drawable.
|
|
* @param i index of the parent to get.
|
|
* @return the parent i.
|
|
*/
|
|
inline const Node* getParent(unsigned int i) const { return _parents[i]; }
|
|
|
|
/**
|
|
* Get the number of parents of node.
|
|
* @return the number of parents of this node.
|
|
*/
|
|
inline unsigned int getNumParents() const { return _parents.size(); }
|
|
|
|
|
|
|
|
/** Set the StateSet attached to the Drawable.
|
|
Previously attached StateSet are automatically unreferenced on
|
|
assignment of a new drawstate.*/
|
|
inline void setStateSet(StateSet *state) { _stateset = state; }
|
|
|
|
/** Get the attached StateSet.*/
|
|
inline StateSet* getStateSet() { return _stateset.get();}
|
|
|
|
/** Get the attached const StateSet.*/
|
|
inline const StateSet* getStateSet() const { return _stateset.get();}
|
|
|
|
/** Get the attached const StateSet,
|
|
* if one is not already attach create one,
|
|
* attach it to the drawable and return a pointer to it.*/
|
|
StateSet* getOrCreateStateSet();
|
|
|
|
|
|
/** Dirty the bounding box, forcing a computeBound() on the next call
|
|
* to getBound(). Should be called in the internal geometry of the Drawable
|
|
* is modified.*/
|
|
void dirtyBound();
|
|
|
|
/** get bounding box of geoset.
|
|
* Note, now made virtual to make it possible to implement user-drawn
|
|
* objects albeit so what crudely, to be improved later.
|
|
*/
|
|
inline const BoundingBox& getBound() const
|
|
{
|
|
if( !_bbox_computed)
|
|
computeBound();
|
|
return _bbox;
|
|
}
|
|
|
|
|
|
/** Set the Shape of the drawable. The shape can be used to
|
|
* speed up collision detection or as a guide for produral
|
|
* geometry generation - see osg::ProduralGeometry.*/
|
|
inline void setShape(Shape* shape) { _shape = shape; }
|
|
|
|
/** Get the Shape of the Drawable.*/
|
|
inline Shape* getShape() { return _shape.get(); }
|
|
|
|
/** Get the const Shape of the const Drawable.*/
|
|
inline const Shape* getShape() const { return _shape.get(); }
|
|
|
|
|
|
|
|
/** Set the drawable to it can or cannot be used in conjunction with OpenGL
|
|
* display lists. With set to true, calls to Drawable::setUseDisplayList,
|
|
* whereas when set to false, no display lists can be created and calls
|
|
* to setUseDisplayList are ignored, and a warning is produced. The later
|
|
* is typically used to guard against the switching on of display lists
|
|
* on objects with dynamic internal data such as continuous Level of Detail
|
|
* algorithms.*/
|
|
void setSupportsDisplayList(bool flag);
|
|
|
|
/** Get whether display lists are supported for this drawable instance.*/
|
|
inline bool getSupportsDisplayList() const { return _supportsDisplayList; }
|
|
|
|
|
|
/** When set to true, force the draw method to use OpenGL Display List for rendering.
|
|
If false rendering directly. If the display list has not been already
|
|
compile the next call to draw will automatically create the display list.*/
|
|
void setUseDisplayList(bool flag);
|
|
|
|
/** Return whether OpenGL display lists are being used for rendering.*/
|
|
inline bool getUseDisplayList() const { return _useDisplayList; }
|
|
|
|
/** Force a recompile on next draw() of any OpenGL display list associated with this geoset.*/
|
|
void dirtyDisplayList();
|
|
|
|
|
|
|
|
/** draw OpenGL primitives.
|
|
* If the drawable has _useDisplayList set to true then use an OpenGL display
|
|
* list, automatically compiling one if required.
|
|
* Otherwise call drawImplementation().
|
|
* Note, draw method should *not* be overridden in subclasses as it
|
|
* manages the optional display list.
|
|
*/
|
|
inline void draw(State& state);
|
|
|
|
/** Immediately compile this drawable into an OpenGL Display List.
|
|
Note I, operation is ignored if _useDisplayList to false.
|
|
Note II, compile is not intended to be overridden in subclasses.*/
|
|
void compile(State& state);
|
|
|
|
|
|
struct AppCallback : public virtual osg::Referenced
|
|
{
|
|
/** do customized app code.*/
|
|
virtual void app(osg::NodeVisitor *visitor, osg::Drawable* drawable) = 0;
|
|
};
|
|
|
|
/** Set the AppCallback which allows users to attach customize the undating of an object during the app traversal.*/
|
|
void setAppCallback(AppCallback* ac);
|
|
|
|
/** Get the non const AppCallback.*/
|
|
AppCallback* getAppCallback() { return _appCallback.get(); }
|
|
|
|
/** Get the const AppCallback.*/
|
|
const AppCallback* getAppCallback() const { return _appCallback.get(); }
|
|
|
|
|
|
struct CullCallback : public virtual osg::Referenced
|
|
{
|
|
/** do customized cull code.*/
|
|
virtual bool cull(osg::NodeVisitor *visitor, osg::Drawable* drawable, osg::State *state=NULL) const = 0;
|
|
};
|
|
|
|
/** Set the CullCallback which allows users to attach customize the culling of Drawable during the cull traversal.*/
|
|
void setCullCallback(CullCallback* cc) { _cullCallback=cc; }
|
|
|
|
/** Get the non const CullCallback.*/
|
|
CullCallback* getCullCallback() { return _cullCallback.get(); }
|
|
|
|
/** Get the const CullCallback.*/
|
|
const CullCallback* getCullCallback() const { return _cullCallback.get(); }
|
|
|
|
|
|
/** Callback attached to an Drawable which allows the users to customize the drawing of an exist Drawable object.
|
|
* The draw callback is implement as a replacement to the Drawable's own drawImplementation() method, if the
|
|
* the user intends to decorate the exist draw code then simple call the drawable->drawImplementation() from
|
|
* with the callbacks drawImplementation() method. This allows the users to do both pre and post callbacks
|
|
* without fuss and can even diable the inner draw in required.*/
|
|
struct DrawCallback : public virtual osg::Referenced
|
|
{
|
|
/** do customized draw code.*/
|
|
virtual void drawImplementation(State& state,const osg::Drawable* drawable) const = 0;
|
|
};
|
|
|
|
/** Set the DrawCallback which allows users to attach customize the drawing of existing Drawable object.*/
|
|
void setDrawCallback(DrawCallback* dc) { _drawCallback=dc; dirtyDisplayList(); }
|
|
|
|
/** Get the non const DrawCallback.*/
|
|
DrawCallback* getDrawCallback() { return _drawCallback.get(); }
|
|
|
|
/** Get the const DrawCallback.*/
|
|
const DrawCallback* getDrawCallback() const { return _drawCallback.get(); }
|
|
|
|
|
|
|
|
/** draw directly ignoring an OpenGL display list which could be attached.
|
|
* This is the internal draw method which does the drawing itself,
|
|
* and is the method to override when deriving from Drawable.
|
|
*/
|
|
virtual void drawImplementation(State& state) const = 0;
|
|
|
|
|
|
|
|
/** use deleteDisplayList instead of glDeleteList to allow
|
|
* OpenGL display list to cached until they can be deleted
|
|
* by the OpenGL context in which they were created, specified
|
|
* by contextID.*/
|
|
static void deleteDisplayList(uint contextID,uint globj);
|
|
|
|
/** flush all the cached display list which need to be deleted
|
|
* in the OpenGL context related to contextID.*/
|
|
static void flushDeletedDisplayLists(uint contextID);
|
|
|
|
|
|
enum AttributeType
|
|
{
|
|
VERTICES,
|
|
NORMALS,
|
|
COLORS,
|
|
TEXTURE_COORDS,
|
|
TEXTURE_COORDS_0 = TEXTURE_COORDS,
|
|
TEXTURE_COORDS_1 = TEXTURE_COORDS_0+1,
|
|
TEXTURE_COORDS_2 = TEXTURE_COORDS_0+2,
|
|
TEXTURE_COORDS_3 = TEXTURE_COORDS_0+3,
|
|
TEXTURE_COORDS_4 = TEXTURE_COORDS_0+4,
|
|
TEXTURE_COORDS_5 = TEXTURE_COORDS_0+5,
|
|
TEXTURE_COORDS_6 = TEXTURE_COORDS_0+6,
|
|
TEXTURE_COORDS_7 = TEXTURE_COORDS_0+7
|
|
// only eight texture coord examples provided here, but underlying code can handle any no of texure units,
|
|
// simply co them as (TEXTURE_COORDS_0+unit).
|
|
};
|
|
|
|
class AttributeFunctor
|
|
{
|
|
public:
|
|
|
|
virtual ~AttributeFunctor() {}
|
|
|
|
virtual void apply(AttributeType,unsigned int,GLbyte*) {}
|
|
virtual void apply(AttributeType,unsigned int,GLshort*) {}
|
|
virtual void apply(AttributeType,unsigned int,GLint*) {}
|
|
|
|
virtual void apply(AttributeType,unsigned int,GLubyte*) {}
|
|
virtual void apply(AttributeType,unsigned int,GLushort*) {}
|
|
virtual void apply(AttributeType,unsigned int,GLuint*) {}
|
|
|
|
virtual void apply(AttributeType,unsigned int,float*) {}
|
|
virtual void apply(AttributeType,unsigned int,Vec2*) {}
|
|
virtual void apply(AttributeType,unsigned int,Vec3*) {}
|
|
virtual void apply(AttributeType,unsigned int,Vec4*) {}
|
|
virtual void apply(AttributeType,unsigned int,UByte4*) {}
|
|
};
|
|
|
|
|
|
/** return true if the Drawable subclass supports accept(AttributeFunctor&).*/
|
|
virtual bool supports(AttributeFunctor&) const { return false; }
|
|
|
|
/** accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has.
|
|
* return true if functor handled by drawable, return false on failure of drawable to generate functor calls.*/
|
|
virtual void accept(AttributeFunctor&) {}
|
|
|
|
|
|
class ConstAttributeFunctor
|
|
{
|
|
public:
|
|
|
|
virtual ~ConstAttributeFunctor() {}
|
|
|
|
virtual void apply(AttributeType,const unsigned int,const GLbyte*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const GLshort*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const GLint*) {}
|
|
|
|
virtual void apply(AttributeType,const unsigned int,const GLubyte*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const GLushort*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const GLuint*) {}
|
|
|
|
virtual void apply(AttributeType,const unsigned int,const float*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const Vec2*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const Vec3*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const Vec4*) {}
|
|
virtual void apply(AttributeType,const unsigned int,const UByte4*) {}
|
|
};
|
|
|
|
/** return true if the Drawable subclass supports accept(ConstAttributeFunctor&).*/
|
|
virtual bool supports(ConstAttributeFunctor&) const { return false; }
|
|
|
|
/** accept an AttributeFunctor and call its methods to tell it about the interal attributes that this Drawable has.
|
|
* return true if functor handled by drawable, return false on failure of drawable to generate functor calls.*/
|
|
virtual void accept(ConstAttributeFunctor&) const {}
|
|
|
|
|
|
class PrimitiveFunctor
|
|
{
|
|
public:
|
|
|
|
virtual ~PrimitiveFunctor() {}
|
|
|
|
virtual void setVertexArray(unsigned int count,const Vec3* vertices) = 0;
|
|
|
|
virtual void drawArrays(GLenum mode,GLint first,GLsizei count) = 0;
|
|
virtual void drawElements(GLenum mode,GLsizei count,const GLubyte* indices) = 0;
|
|
virtual void drawElements(GLenum mode,GLsizei count,const GLushort* indices) = 0;
|
|
virtual void drawElements(GLenum mode,GLsizei count,const GLuint* indices) = 0;
|
|
|
|
virtual void begin(GLenum mode) = 0;
|
|
virtual void vertex(const Vec3& vert) = 0;
|
|
virtual void vertex(float x,float y,float z) = 0;
|
|
virtual void end() = 0;
|
|
|
|
};
|
|
|
|
/** return true if the Drawable subclass supports accept(PrimitiveFunctor&).*/
|
|
virtual bool supports(PrimitiveFunctor&) const { return false; }
|
|
|
|
/** accept a PrimtiveFunctor and call its methods to tell it about the interal primtives that this Drawable has.
|
|
* return true if functor handled by drawable, return false on failure of drawable to generate functor calls.
|
|
* Note, PrimtiveFunctor only provide const access of the primtives, as primitives may be procedurally generated
|
|
* so one cannot modify it.*/
|
|
virtual void accept(PrimitiveFunctor&) const {}
|
|
|
|
protected:
|
|
|
|
Drawable& operator = (const Drawable&) { return *this;}
|
|
|
|
virtual ~Drawable();
|
|
|
|
/** compute the bounding box of the drawable. Method must be
|
|
implemented by subclasses.*/
|
|
virtual bool computeBound() const;
|
|
|
|
void addParent(osg::Node* node);
|
|
void removeParent(osg::Node* node);
|
|
|
|
ParentList _parents;
|
|
friend class Node;
|
|
friend class Geode;
|
|
|
|
ref_ptr<StateSet> _stateset;
|
|
|
|
mutable BoundingBox _bbox;
|
|
mutable bool _bbox_computed;
|
|
|
|
ref_ptr<Shape> _shape;
|
|
|
|
bool _supportsDisplayList;
|
|
bool _useDisplayList;
|
|
|
|
typedef osg::buffered_value<uint> GLObjectList;
|
|
mutable GLObjectList _globjList;
|
|
|
|
ref_ptr<AppCallback> _appCallback;
|
|
ref_ptr<DrawCallback> _drawCallback;
|
|
ref_ptr<CullCallback> _cullCallback;
|
|
|
|
|
|
};
|
|
|
|
inline void Drawable::draw(State& state)
|
|
{
|
|
if (_useDisplayList)
|
|
{
|
|
|
|
// get the contextID (user defined ID of 0 upwards) for the
|
|
// current OpenGL context.
|
|
uint contextID = state.getContextID();
|
|
|
|
// get the globj for the current contextID.
|
|
uint& globj = _globjList[contextID];
|
|
|
|
// call the globj if already set otherwise compile and execute.
|
|
if( globj != 0 )
|
|
{
|
|
glCallList( globj );
|
|
}
|
|
else if (_useDisplayList)
|
|
{
|
|
#ifdef USE_SEPARATE_COMPILE_AND_EXECUTE
|
|
globj = glGenLists( 1 );
|
|
glNewList( globj, GL_COMPILE );
|
|
if (_drawCallback.valid())
|
|
_drawCallback->drawImplementation(state,this);
|
|
else
|
|
drawImplementation(state);
|
|
glEndList();
|
|
|
|
glCallList( globj);
|
|
#else
|
|
globj = glGenLists( 1 );
|
|
glNewList( globj, GL_COMPILE_AND_EXECUTE );
|
|
if (_drawCallback.valid())
|
|
_drawCallback->drawImplementation(state,this);
|
|
else
|
|
drawImplementation(state);
|
|
glEndList();
|
|
#endif
|
|
}
|
|
|
|
}
|
|
else
|
|
{
|
|
// draw object as nature intended..
|
|
if (_drawCallback.valid())
|
|
_drawCallback->drawImplementation(state,this);
|
|
else
|
|
drawImplementation(state);
|
|
}
|
|
};
|
|
|
|
template<class T>
|
|
class TriangleFunctor : public Drawable::PrimitiveFunctor, public T
|
|
{
|
|
public:
|
|
|
|
TriangleFunctor()
|
|
{
|
|
_vertexArraySize=0;
|
|
_vertexArrayPtr=0;
|
|
_modeCache=0;
|
|
}
|
|
|
|
virtual ~TriangleFunctor() {}
|
|
|
|
virtual void setVertexArray(unsigned int count,const Vec3* vertices)
|
|
{
|
|
_vertexArraySize = count;
|
|
_vertexArrayPtr = vertices;
|
|
}
|
|
|
|
virtual void drawArrays(GLenum mode,GLint first,GLsizei count)
|
|
{
|
|
if (_vertexArrayPtr==0 && count==0) return;
|
|
|
|
switch(mode)
|
|
{
|
|
case(GL_TRIANGLES):
|
|
{
|
|
const Vec3* vlast = &_vertexArrayPtr[first+count];
|
|
for(const Vec3* vptr=&_vertexArrayPtr[first];vptr<vlast;vptr+=3)
|
|
operator()(*(vptr),*(vptr+1),*(vptr+2));
|
|
break;
|
|
}
|
|
case(GL_TRIANGLE_STRIP):
|
|
{
|
|
const Vec3* vptr = &_vertexArrayPtr[first];
|
|
for(GLsizei i=2;i<count;++i,++vptr)
|
|
{
|
|
if ((i%2)) operator()(*(vptr),*(vptr+2),*(vptr+1));
|
|
else operator()(*(vptr),*(vptr+1),*(vptr+2));
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUADS):
|
|
{
|
|
const Vec3* vptr = &_vertexArrayPtr[first];
|
|
for(GLsizei i=3;i<count;i+=4,vptr+=4)
|
|
{
|
|
operator()(*(vptr),*(vptr+1),*(vptr+2));
|
|
operator()(*(vptr),*(vptr+2),*(vptr+3));
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUAD_STRIP):
|
|
{
|
|
const Vec3* vptr = &_vertexArrayPtr[first];
|
|
for(GLsizei i=3;i<count;i+=2,vptr+=2)
|
|
{
|
|
operator()(*(vptr),*(vptr+1),*(vptr+2));
|
|
operator()(*(vptr+1),*(vptr+3),*(vptr+2));
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POLYGON): // treat polygons as GL_TRIANGLE_FAN
|
|
case(GL_TRIANGLE_FAN):
|
|
{
|
|
const Vec3* vfirst = &_vertexArrayPtr[first];
|
|
const Vec3* vptr = vfirst+1;
|
|
for(GLsizei i=2;i<count;++i,++vptr)
|
|
{
|
|
operator()(*(vfirst),*(vptr),*(vptr+1));
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POINTS):
|
|
case(GL_LINES):
|
|
case(GL_LINE_STRIP):
|
|
case(GL_LINE_LOOP):
|
|
default:
|
|
// can't be converted into to triangles.
|
|
break;
|
|
}
|
|
}
|
|
|
|
virtual void drawElements(GLenum mode,GLsizei count,const GLubyte* indices)
|
|
{
|
|
if (indices==0 || count==0) return;
|
|
|
|
typedef const GLubyte* IndexPointer;
|
|
|
|
switch(mode)
|
|
{
|
|
case(GL_TRIANGLES):
|
|
{
|
|
IndexPointer ilast = &indices[count];
|
|
for(IndexPointer iptr=indices;iptr<ilast;iptr+=3)
|
|
operator()(_vertexArrayPtr[*iptr],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
break;
|
|
}
|
|
case(GL_TRIANGLE_STRIP):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=2;i<count;++i,++iptr)
|
|
{
|
|
if ((i%2)) operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+2)],_vertexArrayPtr[*(iptr+1)]);
|
|
else operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUADS):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=3;i<count;i+=4,iptr+=4)
|
|
{
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+2)],_vertexArrayPtr[*(iptr+3)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUAD_STRIP):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=3;i<count;i+=2,iptr+=2)
|
|
{
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
operator()(_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+3)],_vertexArrayPtr[*(iptr+2)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POLYGON): // treat polygons as GL_TRIANGLE_FAN
|
|
case(GL_TRIANGLE_FAN):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
const Vec3& vfirst = _vertexArrayPtr[*iptr];
|
|
++iptr;
|
|
for(GLsizei i=2;i<count;++i,++iptr)
|
|
{
|
|
operator()(vfirst,_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POINTS):
|
|
case(GL_LINES):
|
|
case(GL_LINE_STRIP):
|
|
case(GL_LINE_LOOP):
|
|
default:
|
|
// can't be converted into to triangles.
|
|
break;
|
|
}
|
|
}
|
|
|
|
virtual void drawElements(GLenum mode,GLsizei count,const GLushort* indices)
|
|
{
|
|
if (indices==0 || count==0) return;
|
|
|
|
typedef const GLushort* IndexPointer;
|
|
|
|
switch(mode)
|
|
{
|
|
case(GL_TRIANGLES):
|
|
{
|
|
IndexPointer ilast = &indices[count];
|
|
for(IndexPointer iptr=indices;iptr<ilast;iptr+=3)
|
|
{
|
|
operator()(_vertexArrayPtr[*iptr],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_TRIANGLE_STRIP):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=2;i<count;++i,++iptr)
|
|
{
|
|
if ((i%2)) operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+2)],_vertexArrayPtr[*(iptr+1)]);
|
|
else operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUADS):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=3;i<count;i+=4,iptr+=4)
|
|
{
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+2)],_vertexArrayPtr[*(iptr+3)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUAD_STRIP):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=3;i<count;i+=2,iptr+=2)
|
|
{
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
operator()(_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+3)],_vertexArrayPtr[*(iptr+2)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POLYGON): // treat polygons as GL_TRIANGLE_FAN
|
|
case(GL_TRIANGLE_FAN):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
const Vec3& vfirst = _vertexArrayPtr[*iptr];
|
|
++iptr;
|
|
for(GLsizei i=2;i<count;++i,++iptr)
|
|
{
|
|
operator()(vfirst,_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POINTS):
|
|
case(GL_LINES):
|
|
case(GL_LINE_STRIP):
|
|
case(GL_LINE_LOOP):
|
|
default:
|
|
// can't be converted into to triangles.
|
|
break;
|
|
}
|
|
}
|
|
|
|
virtual void drawElements(GLenum mode,GLsizei count,const GLuint* indices)
|
|
{
|
|
if (indices==0 || count==0) return;
|
|
|
|
typedef const GLuint* IndexPointer;
|
|
|
|
switch(mode)
|
|
{
|
|
case(GL_TRIANGLES):
|
|
{
|
|
IndexPointer ilast = &indices[count];
|
|
for(IndexPointer iptr=indices;iptr<ilast;iptr+=3)
|
|
operator()(_vertexArrayPtr[*iptr],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
break;
|
|
}
|
|
case(GL_TRIANGLE_STRIP):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=2;i<count;++i,++iptr)
|
|
{
|
|
if ((i%2)) operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+2)],_vertexArrayPtr[*(iptr+1)]);
|
|
else operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUADS):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=3;i<count;i+=4,iptr+=4)
|
|
{
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+2)],_vertexArrayPtr[*(iptr+3)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_QUAD_STRIP):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
for(GLsizei i=3;i<count;i+=2,iptr+=2)
|
|
{
|
|
operator()(_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+2)]);
|
|
operator()(_vertexArrayPtr[*(iptr+1)],_vertexArrayPtr[*(iptr+3)],_vertexArrayPtr[*(iptr+2)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POLYGON): // treat polygons as GL_TRIANGLE_FAN
|
|
case(GL_TRIANGLE_FAN):
|
|
{
|
|
IndexPointer iptr = indices;
|
|
const Vec3& vfirst = _vertexArrayPtr[*iptr];
|
|
++iptr;
|
|
for(GLsizei i=2;i<count;++i,++iptr)
|
|
{
|
|
operator()(vfirst,_vertexArrayPtr[*(iptr)],_vertexArrayPtr[*(iptr+1)]);
|
|
}
|
|
break;
|
|
}
|
|
case(GL_POINTS):
|
|
case(GL_LINES):
|
|
case(GL_LINE_STRIP):
|
|
case(GL_LINE_LOOP):
|
|
default:
|
|
// can't be converted into to triangles.
|
|
break;
|
|
}
|
|
}
|
|
|
|
|
|
|
|
/** begin(..),vertex(..) & end() are convinience methods for adapting
|
|
* non vertex array primitives to vertex array based primitives.
|
|
* this is done to simplify the implementation of primtive functor
|
|
* subclasses - users only need override drawArray and drawElements.*/
|
|
inline void begin(GLenum mode)
|
|
{
|
|
_modeCache = mode;
|
|
_vertexCache.clear();
|
|
}
|
|
|
|
inline void vertex(const Vec3& vert) { _vertexCache.push_back(vert); }
|
|
inline void vertex(float x,float y,float z) { _vertexCache.push_back(osg::Vec3(x,y,z)); }
|
|
inline void end()
|
|
{
|
|
if (!_vertexCache.empty())
|
|
{
|
|
setVertexArray(_vertexCache.size(),&_vertexCache.front());
|
|
drawArrays(_modeCache,0,_vertexCache.size());
|
|
}
|
|
}
|
|
|
|
protected:
|
|
|
|
|
|
unsigned int _vertexArraySize;
|
|
const Vec3* _vertexArrayPtr;
|
|
|
|
GLenum _modeCache;
|
|
std::vector<Vec3> _vertexCache;
|
|
|
|
};
|
|
|
|
|
|
}
|
|
|
|
#endif
|