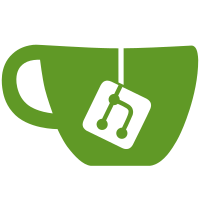
Added support for automatic aliasing of vertex, normal, color etc. arrays to Vertex Attribute equivelants. Added new osg::GLBeginEndAdapter class for runtime conversion from glBegin/glEnd codes to vertex arrray equivelants. Added automatic shader source conversion from gl_ to osg_ builtins.
110 lines
3.5 KiB
C++
110 lines
3.5 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2006 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSG_GLBeginEndAdapter
|
|
#define OSG_GLBeginEndAdapter 1
|
|
|
|
#include <osg/ref_ptr>
|
|
#include <osg/Array>
|
|
#include <osg/Matrixd>
|
|
|
|
namespace osg {
|
|
|
|
// forward declare
|
|
class State;
|
|
|
|
/** A class adapting OpenGL 1.0 glBegin()/glEnd() style code to vertex array based code */
|
|
class OSG_EXPORT GLBeginEndAdapter
|
|
{
|
|
public:
|
|
|
|
GLBeginEndAdapter(State* state=0);
|
|
|
|
enum MatrixMode
|
|
{
|
|
APPLY_LOCAL_MATRICES_TO_VERTICES,
|
|
APPLY_LOCAL_MATRICES_TO_MODELVIEW
|
|
};
|
|
|
|
void setMatrixMode(MatrixMode mode) { _mode = mode; }
|
|
MatrixMode setMatrixMode() const { return _mode; }
|
|
|
|
void PushMatrix();
|
|
void PopMatrix();
|
|
|
|
void LoadIdentity();
|
|
void LoadMatrixd(const GLdouble* m);
|
|
void MultMatrixd(const GLdouble* m);
|
|
|
|
void Translated(GLdouble x, GLdouble y, GLdouble z);
|
|
void Scaled(GLdouble x, GLdouble y, GLdouble z);
|
|
void Rotated(GLdouble angle, GLdouble x, GLdouble y, GLdouble z);
|
|
|
|
void Color4f(GLfloat red, GLfloat green, GLfloat blue, GLfloat alpha);
|
|
void Color4fv(const GLfloat* c) { Color4f(c[0], c[1], c[2], c[3]); }
|
|
|
|
void Vertex3f(GLfloat x, GLfloat y, GLfloat z);
|
|
void Vertex3fv(const GLfloat* v) { Vertex3f(v[0], v[1], v[2]); }
|
|
|
|
void Normal3f(GLfloat x, GLfloat y, GLfloat z);
|
|
void Normal3fv(const GLfloat* n) { Normal3f(n[0], n[1], n[2]); }
|
|
|
|
void TexCoord1f(GLfloat x);
|
|
void TexCoord1fv(const GLfloat* tc) { TexCoord1f(tc[0]); }
|
|
|
|
void TexCoord2f(GLfloat x, GLfloat y);
|
|
void TexCoord2fv(const GLfloat* tc) { TexCoord2f(tc[0],tc[1]); }
|
|
|
|
void TexCoord3f(GLfloat x, GLfloat y, GLfloat z);
|
|
void TexCoord3fv(const GLfloat* tc) { TexCoord3f(tc[0], tc[1], tc[2]); }
|
|
|
|
void TexCoord4f(GLfloat x, GLfloat y, GLfloat z, GLfloat w);
|
|
void TexCoord4fv(const GLfloat* tc) { TexCoord4f(tc[0], tc[1], tc[2], tc[3]); }
|
|
|
|
void Begin(GLenum mode);
|
|
void End();
|
|
|
|
protected:
|
|
|
|
State* _state;
|
|
|
|
MatrixMode _mode;
|
|
|
|
typedef std::list<Matrixd> MatrixStack;
|
|
MatrixStack _matrixStack;
|
|
|
|
bool _normalSet;
|
|
osg::Vec3f _normal;
|
|
|
|
bool _colorSet;
|
|
osg::Vec4f _color;
|
|
|
|
unsigned int _maxNumTexCoordComponents;
|
|
osg::Vec4f _texCoord;
|
|
|
|
|
|
osg::Vec3f _overallNormal;
|
|
osg::Vec4f _overallColor;
|
|
|
|
GLenum _primitiveMode;
|
|
osg::ref_ptr<osg::Vec3Array> _vertices;
|
|
osg::ref_ptr<osg::Vec3Array> _normals;
|
|
osg::ref_ptr<osg::Vec4Array> _colors;
|
|
osg::ref_ptr<osg::Vec4Array> _texCoords;
|
|
};
|
|
|
|
|
|
}
|
|
|
|
#endif
|