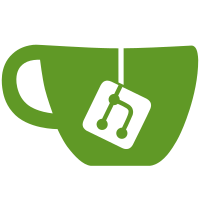
GLObjectVisitor to better fit its function, and added support for releasing objects as well as compiling them.
262 lines
11 KiB
C++
262 lines
11 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2003 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGDB_DATABASEPAGER
|
|
#define OSGDB_DATABASEPAGER 1
|
|
|
|
#include <osg/NodeVisitor>
|
|
#include <osg/Group>
|
|
#include <osg/PagedLOD>
|
|
#include <osg/Drawable>
|
|
|
|
#include <OpenThreads/Thread>
|
|
#include <OpenThreads/Mutex>
|
|
#include <OpenThreads/ScopedLock>
|
|
#include <OpenThreads/Condition>
|
|
|
|
#include <osgDB/SharedStateManager>
|
|
#include <osgDB/Export>
|
|
|
|
#include <map>
|
|
#include <set>
|
|
|
|
namespace osgDB {
|
|
|
|
class Block: public osg::Referenced {
|
|
public:
|
|
Block():_released(false) {}
|
|
|
|
void block()
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> mutlock(_mut);
|
|
if( !_released )
|
|
_cond.wait(&_mut);
|
|
}
|
|
|
|
void release()
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> mutlock(_mut);
|
|
if (!_released)
|
|
{
|
|
_released = true;
|
|
_cond.broadcast();
|
|
}
|
|
}
|
|
|
|
void reset()
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> mutlock(_mut);
|
|
_released = false;
|
|
}
|
|
|
|
protected:
|
|
|
|
~Block()
|
|
{
|
|
release();
|
|
}
|
|
|
|
private:
|
|
OpenThreads::Mutex _mut;
|
|
OpenThreads::Condition _cond;
|
|
bool _released;
|
|
};
|
|
|
|
/** Database paging class which manages the loading of files in a background thread,
|
|
* and syncronizing of loaded models with the main scene graph.*/
|
|
class OSGDB_EXPORT DatabasePager : public osg::NodeVisitor::DatabaseRequestHandler, public OpenThreads::Thread
|
|
{
|
|
public :
|
|
|
|
DatabasePager();
|
|
|
|
/** Add a request to load a node file to end the the database request list.*/
|
|
virtual void requestNodeFile(const std::string& fileName,osg::Group* group, float priority, const osg::FrameStamp* framestamp);
|
|
|
|
/** run does the database paging.*/
|
|
virtual void run();
|
|
|
|
/** Set the use of the frame block which, if enabled, blocks the DatabasePager
|
|
* from executing which the current frame is being drawn.
|
|
* When a single processor machine is being used it can be useful to block on
|
|
* frame to help prevent the database paging thread from slowing the cull and draw
|
|
* traversals which in turn can cause frame drops.*/
|
|
void setUseFrameBlock(bool useFrameBlock) { _useFrameBlock = useFrameBlock; }
|
|
|
|
/** Get the whether UseFrameBlock is on or off.*/
|
|
bool getUseFrameBlock() const { return _useFrameBlock; }
|
|
|
|
Block* getFrameBlock() { return _frameBlock.get(); }
|
|
|
|
/** Set the priority of the database pager thread during the frame (i.e. while cull and draw are running.)*/
|
|
void setThreadPriorityDuringFrame(ThreadPriority duringFrame) { _threadPriorityDuringFrame = duringFrame; }
|
|
|
|
/** Get the priority of the database pager thread during the frame*/
|
|
ThreadPriority getThreadPriorityDuringFrame() const { return _threadPriorityDuringFrame; }
|
|
|
|
/** Set the priority of the database pager thread when the frame is not being exectuted (i.e. before or after cull and draw have run.)*/
|
|
void setThreadPriorityOutwithFrame(ThreadPriority outwithFrame) { _threadPriorityOutwithFrame = outwithFrame; }
|
|
|
|
/** Get the priority of the database pager thread when the frame is not being exectuted.*/
|
|
ThreadPriority getThreadPriorityOutwithFrame() const { return _threadPriorityOutwithFrame; }
|
|
|
|
|
|
/** Signal the database thread that the update, cull and draw has begun for a new frame.
|
|
* Note, this is called by the application so that the database pager can go to sleep while the CPU is busy on the main rendering threads. */
|
|
void signalBeginFrame(const osg::FrameStamp* framestamp);
|
|
|
|
/** Signal the database thread that the update, cull and draw dispatch has completed.
|
|
* Note, this is called by the application so that the database pager can go to wake back up now the main rendering threads are iddle waiting for the next frame.*/
|
|
void signalEndFrame();
|
|
|
|
|
|
/** Find all PagedLOD nodes in a subgraph and register them with
|
|
* the DatabasePager so it can keep track of expired nodes.
|
|
* note, should be only be called from the update thread. */
|
|
void registerPagedLODs(osg::Node* subgraph);
|
|
|
|
|
|
/** Set the amount of time that a subgraph will be kept without being visited in the cull traversal
|
|
* before being removed.*/
|
|
void setExpiryDelay(double expiryDelay) { _expiryDelay = expiryDelay; }
|
|
|
|
/** Get the amount of time that a subgraph will be kept without being visited in the cull traversal
|
|
* before being removed.*/
|
|
double getExpiryDelay() const { return _expiryDelay; }
|
|
|
|
/** set whether the removed subgraphs should be deleted in the database thread or not.*/
|
|
void setDeleteRemovedSubgraphsInDatabaseThread(bool flag) { _deleteRemovedSubgraphsInDatabaseThread = flag; }
|
|
|
|
/** get whether the removed subgraphs should be deleted in the database thread or not.*/
|
|
bool getDeleteRemovedSubgraphsInDatabaseThread() const { return _deleteRemovedSubgraphsInDatabaseThread; }
|
|
|
|
|
|
/** set whether newly loaded textures should have their UnrefImageDataAfterApply set to a specified value.*/
|
|
void setUnrefImageDataAfterApplyPolicy(bool changeAutoUnRef, bool valueAutoUnRef) { _changeAutoUnRef = changeAutoUnRef; _valueAutoUnRef = valueAutoUnRef; }
|
|
|
|
/** get whether newly loaded textures should have their UnrefImageDataAfterApply set to a specified value.*/
|
|
void getUnrefImageDataAfterApplyPolicy(bool& changeAutoUnRef, bool& valueAutoUnRef) const { changeAutoUnRef = _changeAutoUnRef; valueAutoUnRef = _valueAutoUnRef; }
|
|
|
|
|
|
/** set whether newly loaded textures should have their MaxAnisotopy set to a specified value.*/
|
|
void setMaxAnisotropyPolicy(bool changeAnisotropy, float valueAnisotropy) { _changeAnisotropy = changeAnisotropy; _valueAnisotropy = valueAnisotropy; }
|
|
|
|
/** set whether newly loaded textures should have their MaxAnisotopy set to a specified value.*/
|
|
void getMaxAnisotropyPolicy(bool& changeAnisotropy, float& valueAnisotropy) const { changeAnisotropy = _changeAnisotropy; valueAnisotropy = _valueAnisotropy; }
|
|
|
|
|
|
/** Iterate through the active PagedLOD nodes children removing
|
|
* children which havn't been visited since specified expiryTime.
|
|
* note, should be only be called from the update thread. */
|
|
void removeExpiredSubgraphs(double currentFrameTime);
|
|
|
|
/** Add the loaded data to the scene graph.*/
|
|
void addLoadedDataToSceneGraph(double currentFrameTime);
|
|
|
|
/** Merge the changes to the scene graph by calling calling removeExpiredSubgraphs then addLoadedDataToSceneGraph*/
|
|
void updateSceneGraph(double currentFrameTime)
|
|
{
|
|
removeExpiredSubgraphs(currentFrameTime);
|
|
addLoadedDataToSceneGraph(currentFrameTime);
|
|
}
|
|
|
|
|
|
/** Turn the compilation of rendering objects for specfied graphics context on (true) or off(false).*/
|
|
void setCompileGLObjectsForContexID(unsigned int contextID, bool on);
|
|
|
|
/** Get whether the compilation of rendering objects for specfied graphics context on (true) or off(false).*/
|
|
bool getCompileGLObjectsForContexID(unsigned int contextID);
|
|
|
|
/** Compile the rendering objects (display lists,texture objects, VBO's) on loaded subgraph.
|
|
* note, should only be called from the draw thread.*/
|
|
void compileGLObjects(osg::State& state,double& availableTime);
|
|
|
|
|
|
public:
|
|
|
|
typedef std::vector< osg::ref_ptr<osg::PagedLOD> > PagedLODList;
|
|
|
|
typedef std::vector< osg::ref_ptr<osg::StateSet> > StateSetList;
|
|
typedef std::vector< osg::ref_ptr<osg::Drawable> > DrawableList;
|
|
typedef std::pair<StateSetList,DrawableList> DataToCompile;
|
|
typedef std::map< unsigned int, DataToCompile > DataToCompileMap;
|
|
|
|
typedef std::set<unsigned int> ActiveGraphicsContexts;
|
|
|
|
|
|
|
|
struct DatabaseRequest : public osg::Referenced
|
|
{
|
|
DatabaseRequest():
|
|
_numOfRequests(0)
|
|
{}
|
|
|
|
std::string _fileName;
|
|
int _frameNumberFirstRequest;
|
|
double _timestampFirstRequest;
|
|
float _priorityFirstRequest;
|
|
int _frameNumberLastRequest;
|
|
double _timestampLastRequest;
|
|
float _priorityLastRequest;
|
|
unsigned int _numOfRequests;
|
|
osg::ref_ptr<osg::Group> _groupForAddingLoadedSubgraph;
|
|
osg::ref_ptr<osg::Node> _loadedModel;
|
|
DataToCompileMap _dataToCompileMap;
|
|
|
|
};
|
|
|
|
typedef std::vector< osg::ref_ptr<DatabaseRequest> > DatabaseRequestList;
|
|
typedef std::vector< osg::ref_ptr<osg::Object> > ObjectList;
|
|
|
|
protected :
|
|
|
|
virtual ~DatabasePager();
|
|
|
|
bool _useFrameBlock;
|
|
osg::ref_ptr<Block> _frameBlock;
|
|
int _frameNumber;
|
|
|
|
ThreadPriority _threadPriorityDuringFrame;
|
|
ThreadPriority _threadPriorityOutwithFrame;
|
|
|
|
DatabaseRequestList _fileRequestList;
|
|
OpenThreads::Mutex _fileRequestListMutex;
|
|
osg::ref_ptr<Block> _fileRequestListEmptyBlock;
|
|
|
|
DatabaseRequestList _dataToCompileList;
|
|
OpenThreads::Mutex _dataToCompileListMutex;
|
|
|
|
bool _changeAutoUnRef;
|
|
bool _valueAutoUnRef;
|
|
bool _changeAnisotropy;
|
|
float _valueAnisotropy;
|
|
|
|
bool _deleteRemovedSubgraphsInDatabaseThread;
|
|
ObjectList _childrenToDeleteList;
|
|
OpenThreads::Mutex _childrenToDeleteListMutex;
|
|
|
|
DatabaseRequestList _dataToMergeList;
|
|
OpenThreads::Mutex _dataToMergeListMutex;
|
|
|
|
|
|
PagedLODList _pagedLODList;
|
|
|
|
double _expiryDelay;
|
|
|
|
ActiveGraphicsContexts _activeGraphicsContexts;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|