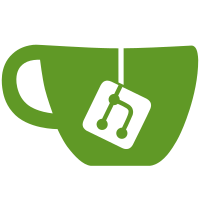
using osgDB::XmlParser. The extension for XML-formatted scenes is .osgx, corresponding to .osgb for binary and .osgt for ascii. It could either be rendered in osgviewer or edited by common web browsers and xml editors because of a range of changes to fit the XML syntax. For example, the recorded class names are slight modified, from 'osg::Geode' to 'osg--Geode'. To quickly get an XML file: # ./osgconv cow.osg cow.osgx The StreamOperator header, InputStreram and OutputStream classes are modified to be more portable for triple ascii/binary/XML formats. I also fixed a bug in readImage()/writeImage() to share image objects if needed. The ReaderWriterOSG2 class now supports all three formats and reading/writing scene objects (not nodes or images), thanks to Torben's advice before. "
98 lines
3.3 KiB
Plaintext
98 lines
3.3 KiB
Plaintext
#ifndef OSGDB_STREAMOPERATOR
|
|
#define OSGDB_STREAMOPERATOR
|
|
|
|
#include <iostream>
|
|
#include <sstream>
|
|
#include <osgDB/DataTypes>
|
|
|
|
namespace osgDB
|
|
{
|
|
|
|
class OSGDB_EXPORT OutputIterator : public osg::Referenced
|
|
{
|
|
public:
|
|
OutputIterator() : _out(0) {}
|
|
virtual ~OutputIterator() {}
|
|
|
|
void setStream( std::ostream* ostream ) { _out = ostream; }
|
|
std::ostream* getStream() { return _out; }
|
|
const std::ostream* getStream() const { return _out; }
|
|
|
|
virtual bool isBinary() const = 0;
|
|
|
|
virtual void writeBool( bool b ) = 0;
|
|
virtual void writeChar( char c ) = 0;
|
|
virtual void writeUChar( unsigned char c ) = 0;
|
|
virtual void writeShort( short s ) = 0;
|
|
virtual void writeUShort( unsigned short s ) = 0;
|
|
virtual void writeInt( int i ) = 0;
|
|
virtual void writeUInt( unsigned int i ) = 0;
|
|
virtual void writeLong( long l ) = 0;
|
|
virtual void writeULong( unsigned long l ) = 0;
|
|
virtual void writeFloat( float f ) = 0;
|
|
virtual void writeDouble( double d ) = 0;
|
|
virtual void writeString( const std::string& s ) = 0;
|
|
virtual void writeStream( std::ostream& (*fn)(std::ostream&) ) = 0;
|
|
virtual void writeBase( std::ios_base& (*fn)(std::ios_base&) ) = 0;
|
|
|
|
virtual void writeGLenum( const ObjectGLenum& value ) = 0;
|
|
virtual void writeProperty( const ObjectProperty& prop ) = 0;
|
|
virtual void writeMark( const ObjectMark& mark ) = 0;
|
|
virtual void writeCharArray( const char* s, unsigned int size ) = 0;
|
|
virtual void writeWrappedString( const std::string& str ) = 0;
|
|
|
|
virtual void flush() { _out->flush(); }
|
|
|
|
protected:
|
|
std::ostream* _out;
|
|
};
|
|
|
|
class OSGDB_EXPORT InputIterator : public osg::Referenced
|
|
{
|
|
public:
|
|
InputIterator() : _in(0), _failed(false) {}
|
|
virtual ~InputIterator() {}
|
|
|
|
void setStream( std::istream* istream ) { _in = istream; }
|
|
std::istream* getStream() { return _in; }
|
|
const std::istream* getStream() const { return _in; }
|
|
|
|
void checkStream() const { if (_in->rdstate()&_in->failbit) _failed = true; }
|
|
bool isFailed() const { return _failed; }
|
|
|
|
virtual bool isBinary() const = 0;
|
|
|
|
virtual void readBool( bool& b ) = 0;
|
|
virtual void readChar( char& c ) = 0;
|
|
virtual void readSChar( signed char& c ) = 0;
|
|
virtual void readUChar( unsigned char& c ) = 0;
|
|
virtual void readShort( short& s ) = 0;
|
|
virtual void readUShort( unsigned short& s ) = 0;
|
|
virtual void readInt( int& i ) = 0;
|
|
virtual void readUInt( unsigned int& i ) = 0;
|
|
virtual void readLong( long& l ) = 0;
|
|
virtual void readULong( unsigned long& l ) = 0;
|
|
virtual void readFloat( float& f ) = 0;
|
|
virtual void readDouble( double& d ) = 0;
|
|
virtual void readString( std::string& s ) = 0;
|
|
virtual void readStream( std::istream& (*fn)(std::istream&) ) = 0;
|
|
virtual void readBase( std::ios_base& (*fn)(std::ios_base&) ) = 0;
|
|
|
|
virtual void readGLenum( ObjectGLenum& value ) = 0;
|
|
virtual void readProperty( ObjectProperty& prop ) = 0;
|
|
virtual void readMark( ObjectMark& mark ) = 0;
|
|
virtual void readCharArray( char* s, unsigned int size ) = 0;
|
|
virtual void readWrappedString( std::string& str ) = 0;
|
|
|
|
virtual bool matchString( const std::string& str ) { return false; }
|
|
virtual void advanceToCurrentEndBracket() {}
|
|
|
|
protected:
|
|
std::istream* _in;
|
|
mutable bool _failed;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|