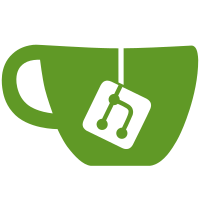
any reference to these in the distribution across to using unsigned char, unsigned short etc. This has been done to keep the OSG code more opaque to what types are.
109 lines
3.2 KiB
C++
109 lines
3.2 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2003 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSG_DEPTH
|
|
#define OSG_DEPTH 1
|
|
|
|
#include <osg/StateAttribute>
|
|
|
|
namespace osg {
|
|
|
|
/** Encapsulate OpenGL glDepthFunc/Mask/Range functions.
|
|
*/
|
|
class SG_EXPORT Depth : public StateAttribute
|
|
{
|
|
public :
|
|
|
|
|
|
Depth();
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
Depth(const Depth& dp,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
StateAttribute(dp,copyop),
|
|
_func(dp._func),
|
|
_depthWriteMask(dp._depthWriteMask),
|
|
_zNear(dp._zNear),
|
|
_zFar(dp._zFar) {}
|
|
|
|
|
|
META_StateAttribute(osg, Depth, DEPTH);
|
|
|
|
/** return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs.*/
|
|
virtual int compare(const StateAttribute& sa) const
|
|
{
|
|
// check the types are equal and then create the rhs variable
|
|
// used by the COMPARE_StateAttribute_Paramter macro's below.
|
|
COMPARE_StateAttribute_Types(Depth,sa)
|
|
|
|
// compare each paramter in turn against the rhs.
|
|
COMPARE_StateAttribute_Parameter(_func)
|
|
COMPARE_StateAttribute_Parameter(_depthWriteMask)
|
|
COMPARE_StateAttribute_Parameter(_zNear)
|
|
COMPARE_StateAttribute_Parameter(_zFar)
|
|
|
|
return 0; // passed all the above comparison macro's, must be equal.
|
|
}
|
|
|
|
virtual void getAssociatedModes(std::vector<GLMode>& modes) const
|
|
{
|
|
modes.push_back(GL_DEPTH_TEST);
|
|
}
|
|
|
|
enum Function
|
|
{
|
|
NEVER = GL_NEVER,
|
|
LESS = GL_LESS,
|
|
EQUAL = GL_EQUAL,
|
|
LEQUAL = GL_LEQUAL,
|
|
GREATER = GL_GREATER,
|
|
NOTEQUAL = GL_NOTEQUAL,
|
|
GEQUAL = GL_GEQUAL,
|
|
ALWAYS = GL_ALWAYS
|
|
};
|
|
|
|
inline void setFunction(Function func) { _func = func; }
|
|
|
|
inline Function getFunction() const { return _func; }
|
|
|
|
|
|
inline void setWriteMask(bool mask) { _depthWriteMask = mask; }
|
|
|
|
inline bool getWriteMask() const { return _depthWriteMask; }
|
|
|
|
inline void setRange(double zNear, double zFar)
|
|
{
|
|
_zNear = zNear;
|
|
_zFar = zFar;
|
|
}
|
|
|
|
inline double getZNear() const { return _zNear; }
|
|
inline double getZFar() const { return _zFar; }
|
|
|
|
virtual void apply(State& state) const;
|
|
|
|
protected:
|
|
|
|
virtual ~Depth();
|
|
|
|
Function _func;
|
|
bool _depthWriteMask;
|
|
|
|
double _zNear;
|
|
double _zFar;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|