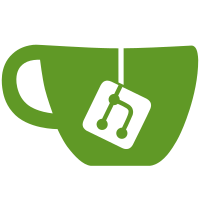
easily retesselate osg::Primitive::POLYGONS found in Geometry objects. Added calls to the tesselate to the lwo and flt loaders.
105 lines
2.6 KiB
Plaintext
105 lines
2.6 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGUTIL_Tesselator
|
|
#define OSGUTIL_Tesselator
|
|
|
|
#include <osg/Geometry>
|
|
|
|
#include <osgUtil/Export>
|
|
|
|
#include <osg/GLU>
|
|
|
|
#include <vector>
|
|
|
|
/* Win32 calling conventions. (or a least thats what the GLUT example tess.c uses.)*/
|
|
#ifndef CALLBACK
|
|
#define CALLBACK
|
|
#endif
|
|
|
|
|
|
namespace osgUtil {
|
|
|
|
/** A simple class for tessellating a single polygon boundary.
|
|
* Currently uses old style glu tessellation functions for portability.
|
|
* It be nice to use the modern glu tessellation functions or to find
|
|
* a small set of code for doing this job better.*/
|
|
class OSGUTIL_EXPORT Tesselator
|
|
{
|
|
public:
|
|
|
|
Tesselator();
|
|
~Tesselator();
|
|
|
|
enum InputBoundaryDirection
|
|
{
|
|
CLOCK_WISE,
|
|
COUNTER_CLOCK_WISE
|
|
};
|
|
|
|
typedef std::vector<osg::Vec3*> VertexPointList;
|
|
|
|
struct Prim : public osg::Referenced
|
|
{
|
|
Prim(GLenum mode):_mode(mode) {}
|
|
|
|
typedef std::vector<osg::Vec3*> VecList;
|
|
|
|
GLenum _mode;
|
|
VecList _vertices;
|
|
};
|
|
|
|
void beginTesselation();
|
|
|
|
void beginContour();
|
|
void addVertex(osg::Vec3* vertex);
|
|
void endContour();
|
|
|
|
void endTesselation();
|
|
|
|
typedef std::vector< osg::ref_ptr<Prim> > PrimList;
|
|
|
|
PrimList& getPrimList() { return _primList; }
|
|
|
|
void retesselatePolygons(osg::Geometry& geom);
|
|
|
|
void reset();
|
|
|
|
protected:
|
|
|
|
void begin(GLenum mode);
|
|
void vertex(osg::Vec3* vertex);
|
|
void combine(osg::Vec3* vertex);
|
|
void end();
|
|
void error(GLenum errorCode);
|
|
|
|
|
|
static void beginCallback(GLenum which, void* userData);
|
|
static void vertexCallback(GLvoid *data, void* userData);
|
|
static void combineCallback(GLdouble coords[3], void* vertex_data[4],
|
|
GLfloat weight[4], void** outData,
|
|
void* useData);
|
|
static void endCallback(void* userData);
|
|
static void errorCallback(GLenum errorCode, void* userData);
|
|
|
|
|
|
struct Vec3d
|
|
{
|
|
double _v[3];
|
|
};
|
|
|
|
|
|
typedef std::vector<Vec3d*> Vec3dList;
|
|
|
|
GLUtesselator* _tobj;
|
|
PrimList _primList;
|
|
Vec3dList _coordData;
|
|
GLenum _errorCode;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|