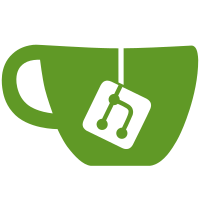
forcing users to use osgDB::readRef*File() methods. The later is preferable as it closes a potential threading bug when using paging databases in conjunction with the osgDB::Registry Object Cache. This threading bug occurs when one thread gets an object from the Cache via an osgDB::read*File() call where only a pointer to the object is passed back, so taking a reference to the object is delayed till it gets reassigned to a ref_ptr<>, but at the same time another thread calls a flush of the Object Cache deleting this object as it's referenceCount is now zero. Using osgDB::readREf*File() makes sure the a ref_ptr<> is passed back and the referenceCount never goes to zero. To ensure the OSG builds when OSG_PROVIDE_READFILE is to OFF the many cases of osgDB::read*File() usage had to be replaced with a ref_ptr<> osgDB::readRef*File() usage. The avoid this change causing lots of other client code to be rewritten to handle the use of ref_ptr<> in place of C pointer I introduced a serious of templte methods in various class to adapt ref_ptr<> to the underly C pointer to be passed to old OSG API's, example of this is found in include/osg/Group: bool addChild(Node* child); // old method which can only be used with a Node* tempalte<class T> bool addChild(const osg::ref_ptr<T>& child) { return addChild(child.get()); } // adapter template method These changes together cover 149 modified files, so it's a large submission. This extent of changes are warrent to make use of the Object Cache and multi-threaded loaded more robust. git-svn-id: http://svn.openscenegraph.org/osg/OpenSceneGraph/trunk@15164 16af8721-9629-0410-8352-f15c8da7e697
138 lines
3.8 KiB
C++
138 lines
3.8 KiB
C++
#include <cstdlib>
|
|
#include <sstream>
|
|
#include <osg/io_utils>
|
|
#include <osg/ArgumentParser>
|
|
#include <osg/Geode>
|
|
#include <osgViewer/Viewer>
|
|
#include <osgViewer/ViewerEventHandlers>
|
|
#include <osgGA/StateSetManipulator>
|
|
|
|
void textInfo(osgText::Text* text)
|
|
{
|
|
const osgText::Text::TextureGlyphQuadMap& tgqm = text->getTextureGlyphQuadMap();
|
|
|
|
const osgText::Text::TextureGlyphQuadMap::const_iterator tgqmi = tgqm.begin();
|
|
|
|
const osgText::Text::GlyphQuads& gq = tgqmi->second;
|
|
|
|
osgText::String& s = text->getText();
|
|
|
|
for(unsigned int i = 0; i < s.size(); i++)
|
|
{
|
|
osg::Vec2 ul = (*gq.getCoords())[0 + (i * 4)]; // upperLeft
|
|
osg::Vec2 ll = (*gq.getCoords())[1 + (i * 4)]; // lowerLeft
|
|
osg::Vec2 lr = (*gq.getCoords())[2 + (i * 4)]; // lowerRight
|
|
osg::Vec2 ur = (*gq.getCoords())[3 + (i * 4)]; // upperRight
|
|
|
|
osg::notify(osg::NOTICE)
|
|
<< "'" << static_cast<char>(s[i]) << "':"
|
|
<< " width(" << lr.x() - ll.x() << ")"
|
|
<< " height(" << ul.y() - ll.y() << ")" << std::endl << "\t"
|
|
<< "ul(" << ul << "), "
|
|
<< "ll(" << ll << "), "
|
|
<< "lr(" << lr << "), "
|
|
<< "ur(" << ur << ")"
|
|
<< std::endl
|
|
;
|
|
}
|
|
}
|
|
|
|
osg::Camera* createOrthoCamera(double width, double height)
|
|
{
|
|
osg::Camera* camera = new osg::Camera();
|
|
|
|
camera->getOrCreateStateSet()->setMode(
|
|
GL_LIGHTING,
|
|
osg::StateAttribute::PROTECTED | osg::StateAttribute::OFF
|
|
);
|
|
|
|
osg::Matrix m = osg::Matrix::ortho2D(0.0f, width, 0.0f, height);
|
|
|
|
camera->setProjectionMatrix(m);
|
|
camera->setReferenceFrame(osg::Transform::ABSOLUTE_RF);
|
|
camera->setViewMatrix(osg::Matrix::identity());
|
|
camera->setClearMask(GL_DEPTH_BUFFER_BIT);
|
|
camera->setRenderOrder(osg::Camera::POST_RENDER);
|
|
|
|
return camera;
|
|
}
|
|
|
|
osgText::Text* createLabel(const std::string& l, const char* f, unsigned int size)
|
|
{
|
|
static osg::Vec3 pos(10.0f, 10.0f, 0.0f);
|
|
|
|
osgText::Text* label = new osgText::Text();
|
|
osg::ref_ptr<osgText::Font> font = osgText::readRefFontFile(f);
|
|
|
|
label->setFont(font);
|
|
label->setCharacterSize(size);
|
|
label->setFontResolution(size, size);
|
|
label->setColor(osg::Vec4(1.0f, 1.0f, 1.0f, 1.0f));
|
|
label->setPosition(pos);
|
|
label->setAlignment(osgText::Text::LEFT_BOTTOM);
|
|
|
|
// It seems to be important we do this last to get best results?
|
|
label->setText(l);
|
|
|
|
textInfo(label);
|
|
|
|
pos.y() += size + 10.0f;
|
|
|
|
return label;
|
|
}
|
|
|
|
typedef std::list<unsigned int> Sizes;
|
|
|
|
int main(int argc, char** argv)
|
|
{
|
|
osgViewer::Viewer viewer;
|
|
osg::ArgumentParser args(&argc, argv);
|
|
|
|
// Make sure we have the minimum args...
|
|
if(argc <= 2)
|
|
{
|
|
osg::notify(osg::FATAL) << "usage: " << args[0] << " fontfile size1 [size2 ...]" << std::endl;
|
|
|
|
return 1;
|
|
}
|
|
|
|
|
|
viewer.addEventHandler( new osgGA::StateSetManipulator(viewer.getCamera()->getOrCreateStateSet()) );
|
|
viewer.addEventHandler(new osgViewer::StatsHandler());
|
|
viewer.addEventHandler(new osgViewer::WindowSizeHandler());
|
|
|
|
osg::Group* group = new osg::Group();
|
|
osg::Camera* camera = createOrthoCamera(1280.0f, 1024.0f);
|
|
|
|
// Create the list of desired sizes.
|
|
Sizes sizes;
|
|
|
|
for(int i = 2; i < argc; i++)
|
|
{
|
|
if(!args.isNumber(i)) continue;
|
|
|
|
sizes.push_back(std::atoi(args[i]));
|
|
}
|
|
|
|
osg::Geode* geode = new osg::Geode();
|
|
|
|
// Add all of our osgText drawables.
|
|
for(Sizes::const_iterator i = sizes.begin(); i != sizes.end(); i++)
|
|
{
|
|
std::stringstream ss;
|
|
|
|
ss << *i << " 1234567890 abcdefghijklmnopqrstuvwxyz ABCDEFGHIJKLMNOPQRSTUVWXYZ";
|
|
|
|
geode->addDrawable(createLabel(ss.str(), args[1], *i));
|
|
}
|
|
|
|
camera->addChild(geode);
|
|
|
|
group->addChild(camera);
|
|
|
|
viewer.setSceneData(group);
|
|
|
|
return viewer.run();
|
|
}
|
|
|