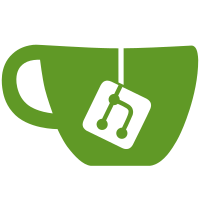
The major modification concern the LineProjector class in Projector.cpp. The intersection was previously done in window space, I've modified it to compute it in object space."
64 lines
2.2 KiB
C++
64 lines
2.2 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2006 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
//osgManipulator - Copyright (C) 2007 Fugro-Jason B.V.
|
|
|
|
#ifndef OSGMANIPULATOR_COMMANDMANAGER
|
|
#define OSGMANIPULATOR_COMMANDMANAGER 1
|
|
|
|
#include <osgManipulator/Dragger>
|
|
#include <osgManipulator/Selection>
|
|
#include <osgManipulator/Constraint>
|
|
|
|
namespace osgManipulator {
|
|
|
|
/**
|
|
* Command manager receives commands from draggers and dispatches them to selections.
|
|
*/
|
|
class OSGMANIPULATOR_EXPORT CommandManager : public osg::Referenced
|
|
{
|
|
public:
|
|
CommandManager();
|
|
|
|
/**
|
|
* Connect a dragger to a selection. The selection will begin listening
|
|
* to commands generated by the dragger. This can be called multiple
|
|
* times to connect many selections to a dragger.
|
|
*/
|
|
virtual bool connect(Dragger& dragger, Selection& selection);
|
|
|
|
virtual bool connect(Dragger& dragger, Constraint& constrain);
|
|
|
|
/** Disconnect the selections from a dragger. */
|
|
virtual bool disconnect(Dragger& dragger);
|
|
|
|
/** Dispatches a command. Usually called from a dragger. */
|
|
virtual void dispatch(MotionCommand& command);
|
|
|
|
/** Add all selections connected to the dragger to the command. */
|
|
void addSelectionsToCommand(MotionCommand& command, Dragger& dragger);
|
|
|
|
protected:
|
|
|
|
virtual ~CommandManager();
|
|
|
|
typedef std::multimap< osg::ref_ptr<Dragger>, osg::ref_ptr<Selection> > DraggerSelectionMap;
|
|
DraggerSelectionMap _draggerSelectionMap;
|
|
|
|
typedef std::multimap< osg::ref_ptr<Dragger>, osg::ref_ptr<Constraint> > DraggerConstraintMap;
|
|
DraggerConstraintMap _draggerConstraintMap;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|