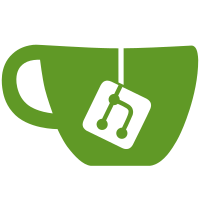
//C++ header to help scripts and editors pick up the fact that the file is a header file.
67 lines
2.0 KiB
Plaintext
67 lines
2.0 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGUTIL_SceneViewManipulator
|
|
#define OSGUTIL_SceneViewManipulator 1
|
|
|
|
#include <osgUtil/Export>
|
|
#include <osgUtil/SceneView>
|
|
#include <osgUtil/CameraManipulator>
|
|
#include <osgUtil/StateSetManipulator>
|
|
#include <osgUtil/GUIEventAdapter>
|
|
#include <osgUtil/GUIActionAdapter>
|
|
|
|
namespace osgUtil{
|
|
|
|
class OSGUTIL_EXPORT SceneViewManipulator : public GUIEventHandler
|
|
{
|
|
public:
|
|
|
|
SceneViewManipulator();
|
|
virtual ~SceneViewManipulator();
|
|
|
|
/** attach a scene view to the manipulator. */
|
|
virtual void setSceneView(SceneView*);
|
|
|
|
/** get the attached a scene view.*/
|
|
virtual SceneView * getSceneView();
|
|
|
|
/** get the attached a const scene view.*/
|
|
virtual const SceneView * getSceneView() const;
|
|
|
|
/** Set the camera manipulator on the object.*/
|
|
virtual void setCameraManipulator(CameraManipulator *cm) {_cm=cm;}
|
|
|
|
/** Get the camera manipulator on the object */
|
|
virtual CameraManipulator *getCameraManipulator() {return _cm.get();}
|
|
|
|
/** Get the const camera manipulator on the object */
|
|
virtual const CameraManipulator *getCameraManipulator() const {return _cm.get();}
|
|
|
|
/** Set the geostate manipulator on the object.*/
|
|
virtual void setStateSetManipulator(StateSetManipulator *cm) {_gm=cm;}
|
|
|
|
/** Get the geostate manipulator on the object */
|
|
virtual StateSetManipulator *getStateSetManipulator() { return _gm.get();}
|
|
|
|
/** Get the geostate manipulator on the object */
|
|
virtual const StateSetManipulator *getStateSetManipulator() const {return _gm.get();}
|
|
|
|
/** Handle events, return true if handled, false otherwise.*/
|
|
virtual bool handle(const GUIEventAdapter& ea,GUIActionAdapter& us);
|
|
|
|
protected:
|
|
|
|
// Reference pointer to our scene view
|
|
osg::ref_ptr<SceneView> _sv;
|
|
|
|
osg::ref_ptr<CameraManipulator> _cm;
|
|
osg::ref_ptr<StateSetManipulator> _gm;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|