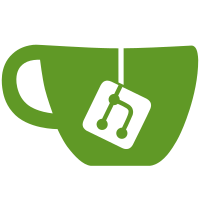
osgshape osgsimplifier osgsimulation osgslice osgspacewarp osgspheresegment osgspotlight osgstereoimage
97 lines
2.8 KiB
C++
97 lines
2.8 KiB
C++
#include <osg/Geode>
|
|
#include <osg/ShapeDrawable>
|
|
#include <osg/Material>
|
|
#include <osg/Texture2D>
|
|
|
|
#include <osgViewer/Viewer>
|
|
|
|
#include <osgDB/ReadFile>
|
|
|
|
#include <osg/Math>
|
|
|
|
// for the grid data..
|
|
#include "../osghangglide/terrain_coords.h"
|
|
|
|
osg::Geode* createShapes()
|
|
{
|
|
osg::Geode* geode = new osg::Geode();
|
|
|
|
// ---------------------------------------
|
|
// Set up a StateSet to texture the objects
|
|
// ---------------------------------------
|
|
osg::StateSet* stateset = new osg::StateSet();
|
|
|
|
osg::Image* image = osgDB::readImageFile( "Images/lz.rgb" );
|
|
|
|
if (image)
|
|
{
|
|
osg::Texture2D* texture = new osg::Texture2D;
|
|
texture->setImage(image);
|
|
stateset->setTextureAttributeAndModes(0,texture,osg::StateAttribute::ON);
|
|
}
|
|
|
|
geode->setStateSet( stateset );
|
|
|
|
float radius = 0.8f;
|
|
float height = 1.0f;
|
|
|
|
osg::TessellationHints* hints = new osg::TessellationHints;
|
|
hints->setDetailRatio(0.5f);
|
|
|
|
geode->addDrawable(new osg::ShapeDrawable(new osg::Sphere(osg::Vec3(0.0f,0.0f,0.0f),radius),hints));
|
|
geode->addDrawable(new osg::ShapeDrawable(new osg::Box(osg::Vec3(2.0f,0.0f,0.0f),2*radius),hints));
|
|
geode->addDrawable(new osg::ShapeDrawable(new osg::Cone(osg::Vec3(4.0f,0.0f,0.0f),radius,height),hints));
|
|
geode->addDrawable(new osg::ShapeDrawable(new osg::Cylinder(osg::Vec3(6.0f,0.0f,0.0f),radius,height),hints));
|
|
geode->addDrawable(new osg::ShapeDrawable(new osg::Capsule(osg::Vec3(8.0f,0.0f,0.0f),radius,height),hints));
|
|
|
|
osg::HeightField* grid = new osg::HeightField;
|
|
grid->allocate(38,39);
|
|
grid->setXInterval(0.28f);
|
|
grid->setYInterval(0.28f);
|
|
|
|
for(unsigned int r=0;r<39;++r)
|
|
{
|
|
for(unsigned int c=0;c<38;++c)
|
|
{
|
|
grid->setHeight(c,r,vertex[r+c*39][2]);
|
|
}
|
|
}
|
|
geode->addDrawable(new osg::ShapeDrawable(grid));
|
|
|
|
osg::ConvexHull* mesh = new osg::ConvexHull;
|
|
osg::Vec3Array* vertices = new osg::Vec3Array(4);
|
|
(*vertices)[0].set(9.0+0.0f,-1.0f+2.0f,-1.0f+0.0f);
|
|
(*vertices)[1].set(9.0+1.0f,-1.0f+0.0f,-1.0f+0.0f);
|
|
(*vertices)[2].set(9.0+2.0f,-1.0f+2.0f,-1.0f+0.0f);
|
|
(*vertices)[3].set(9.0+1.0f,-1.0f+1.0f,-1.0f+2.0f);
|
|
osg::UByteArray* indices = new osg::UByteArray(12);
|
|
(*indices)[0]=0;
|
|
(*indices)[1]=2;
|
|
(*indices)[2]=1;
|
|
(*indices)[3]=0;
|
|
(*indices)[4]=1;
|
|
(*indices)[5]=3;
|
|
(*indices)[6]=1;
|
|
(*indices)[7]=2;
|
|
(*indices)[8]=3;
|
|
(*indices)[9]=2;
|
|
(*indices)[10]=0;
|
|
(*indices)[11]=3;
|
|
mesh->setVertices(vertices);
|
|
mesh->setIndices(indices);
|
|
geode->addDrawable(new osg::ShapeDrawable(mesh));
|
|
|
|
return geode;
|
|
}
|
|
|
|
int main(int, char **)
|
|
{
|
|
// construct the viewer.
|
|
osgViewer::Viewer viewer;
|
|
|
|
// add model to viewer.
|
|
viewer.setSceneData( createShapes() );
|
|
|
|
return viewer.run();
|
|
}
|