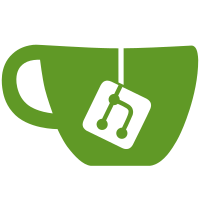
Note, this required adding a unsigned int context ID to the osg::isGLUExtensionSupported(,) and osg::isGLExtensionSupported(,) functions. This may require reimplementation of end user code to accomodate the new calling convention.
121 lines
4.1 KiB
C++
121 lines
4.1 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2005 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSG_BLENDCOLOR
|
|
#define OSG_BLENDCOLOR 1
|
|
|
|
#include <osg/GL>
|
|
#include <osg/StateAttribute>
|
|
#include <osg/ref_ptr>
|
|
#include <osg/Vec4>
|
|
|
|
|
|
|
|
namespace osg {
|
|
|
|
/** Encapsulates OpenGL blend/transparency state. */
|
|
class OSG_EXPORT BlendColor : public StateAttribute
|
|
{
|
|
public :
|
|
|
|
BlendColor();
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
BlendColor(const BlendColor& trans,const CopyOp& copyop=CopyOp::SHALLOW_COPY):
|
|
StateAttribute(trans,copyop),
|
|
_constantColor(trans._constantColor) {}
|
|
|
|
META_StateAttribute(osg, BlendColor,BLENDCOLOR);
|
|
|
|
/** Return -1 if *this < *rhs, 0 if *this==*rhs, 1 if *this>*rhs. */
|
|
virtual int compare(const StateAttribute& sa) const
|
|
{
|
|
// Check for equal types, then create the rhs variable
|
|
// used by the COMPARE_StateAttribute_Paramter macros below.
|
|
COMPARE_StateAttribute_Types(BlendColor,sa)
|
|
|
|
// Compare each parameter in turn against the rhs.
|
|
COMPARE_StateAttribute_Parameter(_constantColor)
|
|
|
|
return 0; // Passed all the above comparison macros, so must be equal.
|
|
}
|
|
|
|
virtual bool getModeUsage(ModeUsage& usage) const
|
|
{
|
|
usage.usesMode(GL_BLEND);
|
|
return true;
|
|
}
|
|
|
|
void setConstantColor(const osg::Vec4& color) { _constantColor = color; }
|
|
inline osg::Vec4 getConstantColor() const { return _constantColor; }
|
|
|
|
virtual void apply(State& state) const;
|
|
|
|
|
|
|
|
|
|
/** Encapsulates queries of extension availability, obtains extension
|
|
* function pointers, and provides convinience wrappers for
|
|
* calling extension functions. */
|
|
class OSG_EXPORT Extensions : public osg::Referenced
|
|
{
|
|
public:
|
|
Extensions(unsigned int contextID);
|
|
|
|
Extensions(const Extensions& rhs);
|
|
|
|
void lowestCommonDenominator(const Extensions& rhs);
|
|
|
|
void setupGLExtenions(unsigned int contextID);
|
|
|
|
void setBlendColorSupported(bool flag) { _isBlendColorSupported=flag; }
|
|
bool isBlendColorSupported() const { return _isBlendColorSupported; }
|
|
|
|
void setBlendColorProc(void* ptr) { _glBlendColor = ptr; }
|
|
void glBlendColor(GLclampf red , GLclampf green , GLclampf blue , GLclampf alpha) const;
|
|
|
|
protected:
|
|
|
|
~Extensions() {}
|
|
|
|
bool _isBlendColorSupported;
|
|
|
|
void* _glBlendColor;
|
|
|
|
};
|
|
|
|
/** Returns the Extensions object for the given context.
|
|
* If createIfNotInitalized is true and the Exentsions object doesn't
|
|
* exist, getExtensions() creates it on the given context.
|
|
* Returns NULL if createIfNotInitalized is false and the Extensions
|
|
* object doesn't exist. */
|
|
static Extensions* getExtensions(unsigned int contextID,bool createIfNotInitalized);
|
|
|
|
/** setExtensions() allows users to override the extensions across graphics contexts.
|
|
* Typically used when you have different extensions supported across graphics pipes,
|
|
* but need to ensure that they all use the same low common denominator extensions. */
|
|
static void setExtensions(unsigned int contextID,Extensions* extensions);
|
|
|
|
|
|
|
|
protected :
|
|
|
|
virtual ~BlendColor();
|
|
|
|
osg::Vec4 _constantColor;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|