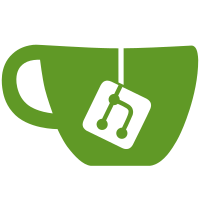
forcing users to use osgDB::readRef*File() methods. The later is preferable as it closes a potential threading bug when using paging databases in conjunction with the osgDB::Registry Object Cache. This threading bug occurs when one thread gets an object from the Cache via an osgDB::read*File() call where only a pointer to the object is passed back, so taking a reference to the object is delayed till it gets reassigned to a ref_ptr<>, but at the same time another thread calls a flush of the Object Cache deleting this object as it's referenceCount is now zero. Using osgDB::readREf*File() makes sure the a ref_ptr<> is passed back and the referenceCount never goes to zero. To ensure the OSG builds when OSG_PROVIDE_READFILE is to OFF the many cases of osgDB::read*File() usage had to be replaced with a ref_ptr<> osgDB::readRef*File() usage. The avoid this change causing lots of other client code to be rewritten to handle the use of ref_ptr<> in place of C pointer I introduced a serious of templte methods in various class to adapt ref_ptr<> to the underly C pointer to be passed to old OSG API's, example of this is found in include/osg/Group: bool addChild(Node* child); // old method which can only be used with a Node* tempalte<class T> bool addChild(const osg::ref_ptr<T>& child) { return addChild(child.get()); } // adapter template method These changes together cover 149 modified files, so it's a large submission. This extent of changes are warrent to make use of the Object Cache and multi-threaded loaded more robust. git-svn-id: http://svn.openscenegraph.org/osg/OpenSceneGraph/trunk@15164 16af8721-9629-0410-8352-f15c8da7e697
184 lines
6.9 KiB
C++
184 lines
6.9 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2010 Robert Osfield
|
|
*
|
|
* This application is open source and may be redistributed and/or modified
|
|
* freely and without restriction, both in commercial and non commercial applications,
|
|
* as long as this copyright notice is maintained.
|
|
*
|
|
* This application is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
|
|
*/
|
|
|
|
#include <osgDB/ReadFile>
|
|
#include <osgUtil/Optimizer>
|
|
#include <osg/CoordinateSystemNode>
|
|
|
|
#include <osg/Switch>
|
|
#include <osg/Types>
|
|
#include <osgText/Text>
|
|
|
|
#include <osgViewer/Viewer>
|
|
#include <osgViewer/ViewerEventHandlers>
|
|
|
|
#include <osgGA/TrackballManipulator>
|
|
#include <osgGA/FlightManipulator>
|
|
#include <osgGA/DriveManipulator>
|
|
#include <osgGA/KeySwitchMatrixManipulator>
|
|
#include <osgGA/StateSetManipulator>
|
|
#include <osgGA/AnimationPathManipulator>
|
|
#include <osgGA/TerrainManipulator>
|
|
#include <osgGA/SphericalManipulator>
|
|
|
|
#include <osgGA/Device>
|
|
|
|
#include <iostream>
|
|
|
|
|
|
int main(int argc, char** argv)
|
|
{
|
|
// use an ArgumentParser object to manage the program arguments.
|
|
osg::ArgumentParser arguments(&argc,argv);
|
|
|
|
arguments.getApplicationUsage()->setApplicationName(arguments.getApplicationName());
|
|
arguments.getApplicationUsage()->setDescription(arguments.getApplicationName()+" is the standard OpenSceneGraph example which loads and visualises 3d models.");
|
|
arguments.getApplicationUsage()->setCommandLineUsage(arguments.getApplicationName()+" [options] filename ...");
|
|
arguments.getApplicationUsage()->addCommandLineOption("--image <filename>","Load an image and render it on a quad");
|
|
arguments.getApplicationUsage()->addCommandLineOption("--dem <filename>","Load an image/DEM and render it on a HeightField");
|
|
arguments.getApplicationUsage()->addCommandLineOption("--login <url> <username> <password>","Provide authentication information for http file access.");
|
|
arguments.getApplicationUsage()->addCommandLineOption("-p <filename>","Play specified camera path animation file, previously saved with 'z' key.");
|
|
arguments.getApplicationUsage()->addCommandLineOption("--speed <factor>","Speed factor for animation playing (1 == normal speed).");
|
|
arguments.getApplicationUsage()->addCommandLineOption("--device <device-name>","add named device to the viewer");
|
|
|
|
osgViewer::Viewer viewer(arguments);
|
|
|
|
unsigned int helpType = 0;
|
|
if ((helpType = arguments.readHelpType()))
|
|
{
|
|
arguments.getApplicationUsage()->write(std::cout, helpType);
|
|
return 1;
|
|
}
|
|
|
|
// report any errors if they have occurred when parsing the program arguments.
|
|
if (arguments.errors())
|
|
{
|
|
arguments.writeErrorMessages(std::cout);
|
|
return 1;
|
|
}
|
|
|
|
if (arguments.argc()<=1)
|
|
{
|
|
arguments.getApplicationUsage()->write(std::cout,osg::ApplicationUsage::COMMAND_LINE_OPTION);
|
|
return 1;
|
|
}
|
|
|
|
std::string url, username, password;
|
|
while(arguments.read("--login",url, username, password))
|
|
{
|
|
if (!osgDB::Registry::instance()->getAuthenticationMap())
|
|
{
|
|
osgDB::Registry::instance()->setAuthenticationMap(new osgDB::AuthenticationMap);
|
|
osgDB::Registry::instance()->getAuthenticationMap()->addAuthenticationDetails(
|
|
url,
|
|
new osgDB::AuthenticationDetails(username, password)
|
|
);
|
|
}
|
|
}
|
|
|
|
std::string device;
|
|
while(arguments.read("--device", device))
|
|
{
|
|
osg::ref_ptr<osgGA::Device> dev = osgDB::readRefFile<osgGA::Device>(device);
|
|
if (dev.valid())
|
|
{
|
|
viewer.addDevice(dev);
|
|
}
|
|
}
|
|
|
|
// set up the camera manipulators.
|
|
{
|
|
osg::ref_ptr<osgGA::KeySwitchMatrixManipulator> keyswitchManipulator = new osgGA::KeySwitchMatrixManipulator;
|
|
|
|
keyswitchManipulator->addMatrixManipulator( '1', "Trackball", new osgGA::TrackballManipulator() );
|
|
keyswitchManipulator->addMatrixManipulator( '2', "Flight", new osgGA::FlightManipulator() );
|
|
keyswitchManipulator->addMatrixManipulator( '3', "Drive", new osgGA::DriveManipulator() );
|
|
keyswitchManipulator->addMatrixManipulator( '4', "Terrain", new osgGA::TerrainManipulator() );
|
|
keyswitchManipulator->addMatrixManipulator( '5', "Orbit", new osgGA::OrbitManipulator() );
|
|
keyswitchManipulator->addMatrixManipulator( '6', "FirstPerson", new osgGA::FirstPersonManipulator() );
|
|
keyswitchManipulator->addMatrixManipulator( '7', "Spherical", new osgGA::SphericalManipulator() );
|
|
|
|
std::string pathfile;
|
|
double animationSpeed = 1.0;
|
|
while(arguments.read("--speed",animationSpeed) ) {}
|
|
char keyForAnimationPath = '8';
|
|
while (arguments.read("-p",pathfile))
|
|
{
|
|
osgGA::AnimationPathManipulator* apm = new osgGA::AnimationPathManipulator(pathfile);
|
|
if (apm || !apm->valid())
|
|
{
|
|
apm->setTimeScale(animationSpeed);
|
|
|
|
unsigned int num = keyswitchManipulator->getNumMatrixManipulators();
|
|
keyswitchManipulator->addMatrixManipulator( keyForAnimationPath, "Path", apm );
|
|
keyswitchManipulator->selectMatrixManipulator(num);
|
|
++keyForAnimationPath;
|
|
}
|
|
}
|
|
|
|
viewer.setCameraManipulator( keyswitchManipulator.get() );
|
|
}
|
|
|
|
// add the state manipulator
|
|
viewer.addEventHandler( new osgGA::StateSetManipulator(viewer.getCamera()->getOrCreateStateSet()) );
|
|
|
|
// add the thread model handler
|
|
viewer.addEventHandler(new osgViewer::ThreadingHandler);
|
|
|
|
// add the window size toggle handler
|
|
viewer.addEventHandler(new osgViewer::WindowSizeHandler);
|
|
|
|
// add the stats handler
|
|
viewer.addEventHandler(new osgViewer::StatsHandler);
|
|
|
|
// add the help handler
|
|
viewer.addEventHandler(new osgViewer::HelpHandler(arguments.getApplicationUsage()));
|
|
|
|
// add the record camera path handler
|
|
viewer.addEventHandler(new osgViewer::RecordCameraPathHandler);
|
|
|
|
// add the LOD Scale handler
|
|
viewer.addEventHandler(new osgViewer::LODScaleHandler);
|
|
|
|
// add the screen capture handler
|
|
viewer.addEventHandler(new osgViewer::ScreenCaptureHandler);
|
|
|
|
// load the data
|
|
osg::ref_ptr<osg::Node> loadedModel = osgDB::readRefNodeFiles(arguments);
|
|
if (!loadedModel)
|
|
{
|
|
std::cout << arguments.getApplicationName() <<": No data loaded" << std::endl;
|
|
return 1;
|
|
}
|
|
|
|
// any option left unread are converted into errors to write out later.
|
|
arguments.reportRemainingOptionsAsUnrecognized();
|
|
|
|
// report any errors if they have occurred when parsing the program arguments.
|
|
if (arguments.errors())
|
|
{
|
|
arguments.writeErrorMessages(std::cout);
|
|
return 1;
|
|
}
|
|
|
|
|
|
// optimize the scene graph, remove redundant nodes and state etc.
|
|
osgUtil::Optimizer optimizer;
|
|
optimizer.optimize(loadedModel);
|
|
|
|
viewer.setSceneData(loadedModel);
|
|
|
|
viewer.realize();
|
|
|
|
return viewer.run();
|
|
|
|
}
|