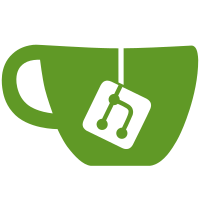
the names of the operations to be logged for stats purposes, or used when do searches of the operation list. The keep member variable tells the graphics thread run loop wether to remove the entry from the list once its been called.
80 lines
2.7 KiB
C++
80 lines
2.7 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2005 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGPRODUCER_GRAPHICSCONTEXTIMPLEMENTATION
|
|
#define OSGPRODUCER_GRAPHICSCONTEXTIMPLEMENTATION 1
|
|
|
|
|
|
#include <osg/GraphicsContext>
|
|
|
|
#include <Producer/RenderSurface>
|
|
|
|
#include <osgProducer/Export>
|
|
|
|
|
|
|
|
namespace osgProducer {
|
|
|
|
class OSGPRODUCER_EXPORT GraphicsContextImplementation : public osg::GraphicsContext
|
|
{
|
|
public :
|
|
|
|
/** Construct a graphics context to specified traits.*/
|
|
GraphicsContextImplementation(Traits* traits);
|
|
|
|
/** Construct a graphics context with specified RenderSurface.*/
|
|
GraphicsContextImplementation(Producer::RenderSurface* rs);
|
|
|
|
/** Return true of graphics context is realized.*/
|
|
|
|
/** Return the RenderSurface that implements the graphics context.*/
|
|
Producer::RenderSurface* getRenderSurface() { return _rs.get(); }
|
|
|
|
/** Return the const RenderSurface that implements the graphics context.*/
|
|
const Producer::RenderSurface* getRenderSurface() const { return _rs.get(); }
|
|
|
|
|
|
/** Realise the GraphicsContext.*/
|
|
virtual bool realizeImplementation();
|
|
|
|
/** Return true if the graphics context has been realised and is ready to use.*/
|
|
virtual bool isRealizedImplementation() const { return _rs.valid() && _rs->isRealized(); }
|
|
|
|
/** Close the graphics context.*/
|
|
virtual void closeImplementation();
|
|
|
|
/** Make this graphics context current.*/
|
|
virtual void makeCurrentImplementation();
|
|
|
|
/** Make this graphics context current with specified read context.*/
|
|
virtual void makeContextCurrentImplementation(GraphicsContext* readContext);
|
|
|
|
/** Bind the graphics context to associated texture.*/
|
|
virtual void bindPBufferToTextureImplementation(GLenum buffer);
|
|
|
|
/** swap the front and back buffers.*/
|
|
virtual void swapBuffersImplementation();
|
|
|
|
|
|
protected:
|
|
|
|
virtual ~GraphicsContextImplementation();
|
|
|
|
bool _closeOnDestruction;
|
|
osg::ref_ptr<Producer::RenderSurface> _rs;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|