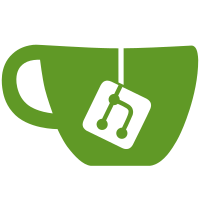
* Change ref_ptr to observer_ptr to avoid cross reference and leak in Skinning * Set invalidate to true to re run the check visitor in Skeleton * Shallow copy Sampler in channel copy constructor * Add accessor in VertexInfluence * Remove dead code in Timeline.cpp * Dont force linking in Bone::UpdateBone, the decision is done by the user or the manager * Add offset in timeline stats to display each manager on the screen * Add a flag in animation manager base to enable or not automatic link when modifying the manager
51 lines
1.8 KiB
C++
51 lines
1.8 KiB
C++
/* -*-c++-*-
|
|
* Copyright (C) 2008 Cedric Pinson <mornifle@plopbyte.net>
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGANIMATION_SKELETON_H
|
|
#define OSGANIMATION_SKELETON_H
|
|
|
|
#include <osg/MatrixTransform>
|
|
#include <osgAnimation/Bone>
|
|
#include <osgAnimation/Export>
|
|
|
|
namespace osgAnimation
|
|
{
|
|
|
|
class OSGANIMATION_EXPORT Skeleton : public Bone
|
|
{
|
|
public:
|
|
META_Node(osgAnimation, Skeleton);
|
|
|
|
class OSGANIMATION_EXPORT UpdateSkeleton : public osg::NodeCallback
|
|
{
|
|
public:
|
|
META_Object(osgAnimation, UpdateSkeleton);
|
|
UpdateSkeleton() : _needValidate(true) {}
|
|
UpdateSkeleton(const UpdateSkeleton& us, const osg::CopyOp& copyop= osg::CopyOp::SHALLOW_COPY) : osg::Object(us, copyop), osg::NodeCallback(us, copyop) { _needValidate = true;}
|
|
virtual void operator()(osg::Node* node, osg::NodeVisitor* nv);
|
|
|
|
protected:
|
|
bool _needValidate;
|
|
};
|
|
|
|
Skeleton(const Skeleton& b, const osg::CopyOp& copyop= osg::CopyOp::SHALLOW_COPY) : Bone(b,copyop) {}
|
|
Skeleton();
|
|
void setDefaultUpdateCallback(void);
|
|
void computeBindMatrix() { _invBindInSkeletonSpace = osg::Matrix::inverse(_bindInBoneSpace); _needToRecomputeBindMatrix = false; }
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|