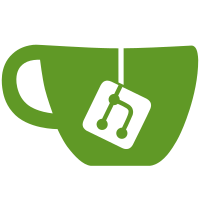
forcing users to use osgDB::readRef*File() methods. The later is preferable as it closes a potential threading bug when using paging databases in conjunction with the osgDB::Registry Object Cache. This threading bug occurs when one thread gets an object from the Cache via an osgDB::read*File() call where only a pointer to the object is passed back, so taking a reference to the object is delayed till it gets reassigned to a ref_ptr<>, but at the same time another thread calls a flush of the Object Cache deleting this object as it's referenceCount is now zero. Using osgDB::readREf*File() makes sure the a ref_ptr<> is passed back and the referenceCount never goes to zero. To ensure the OSG builds when OSG_PROVIDE_READFILE is to OFF the many cases of osgDB::read*File() usage had to be replaced with a ref_ptr<> osgDB::readRef*File() usage. The avoid this change causing lots of other client code to be rewritten to handle the use of ref_ptr<> in place of C pointer I introduced a serious of templte methods in various class to adapt ref_ptr<> to the underly C pointer to be passed to old OSG API's, example of this is found in include/osg/Group: bool addChild(Node* child); // old method which can only be used with a Node* tempalte<class T> bool addChild(const osg::ref_ptr<T>& child) { return addChild(child.get()); } // adapter template method These changes together cover 149 modified files, so it's a large submission. This extent of changes are warrent to make use of the Object Cache and multi-threaded loaded more robust. git-svn-id: http://svn.openscenegraph.org/osg/OpenSceneGraph/trunk@15164 16af8721-9629-0410-8352-f15c8da7e697
184 lines
5.5 KiB
C++
184 lines
5.5 KiB
C++
/* OpenSceneGraph example, osglogicop.
|
|
*
|
|
* Permission is hereby granted, free of charge, to any person obtaining a copy
|
|
* of this software and associated documentation files (the "Software"), to deal
|
|
* in the Software without restriction, including without limitation the rights
|
|
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
|
* copies of the Software, and to permit persons to whom the Software is
|
|
* furnished to do so, subject to the following conditions:
|
|
*
|
|
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
|
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
|
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
|
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
|
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
|
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
|
* THE SOFTWARE.
|
|
*/
|
|
|
|
#include <osg/Geode>
|
|
#include <osg/Group>
|
|
#include <osg/Notify>
|
|
#include <osg/LogicOp>
|
|
|
|
#include <osgDB/Registry>
|
|
#include <osgDB/ReadFile>
|
|
|
|
#include <osgViewer/Viewer>
|
|
|
|
#include <osgUtil/Optimizer>
|
|
|
|
#include <iostream>
|
|
|
|
const int _ops_nb=16;
|
|
const osg::LogicOp::Opcode _operations[_ops_nb]=
|
|
{
|
|
osg::LogicOp::CLEAR,
|
|
osg::LogicOp::SET,
|
|
osg::LogicOp::COPY,
|
|
osg::LogicOp::COPY_INVERTED,
|
|
osg::LogicOp::NOOP,
|
|
osg::LogicOp::INVERT,
|
|
osg::LogicOp::AND,
|
|
osg::LogicOp::NAND,
|
|
osg::LogicOp::OR,
|
|
osg::LogicOp::NOR,
|
|
osg::LogicOp::XOR,
|
|
osg::LogicOp::EQUIV,
|
|
osg::LogicOp::AND_REVERSE,
|
|
osg::LogicOp::AND_INVERTED,
|
|
osg::LogicOp::OR_REVERSE,
|
|
osg::LogicOp::OR_INVERTED
|
|
};
|
|
|
|
const char* _ops_name[_ops_nb]=
|
|
{
|
|
"osg::LogicOp::CLEAR",
|
|
"osg::LogicOp::SET",
|
|
"osg::LogicOp::COPY",
|
|
"osg::LogicOp::COPY_INVERTED",
|
|
"osg::LogicOp::NOOP",
|
|
"osg::LogicOp::INVERT",
|
|
"osg::LogicOp::AND",
|
|
"osg::LogicOp::NAND",
|
|
"osg::LogicOp::OR",
|
|
"osg::LogicOp::NOR",
|
|
"osg::LogicOp::XOR",
|
|
"osg::LogicOp::EQUIV",
|
|
"osg::LogicOp::AND_REVERSE",
|
|
"osg::LogicOp::AND_INVERTED",
|
|
"osg::LogicOp::OR_REVERSE",
|
|
"osg::LogicOp::OR_INVERTED"
|
|
};
|
|
|
|
class TechniqueEventHandler : public osgGA::GUIEventHandler
|
|
{
|
|
public:
|
|
|
|
TechniqueEventHandler(osg::LogicOp* logicOp) { _logicOp =logicOp;_ops_index=_ops_nb-1;}
|
|
TechniqueEventHandler() { std::cerr<<"Error, can't initialize it!";}
|
|
|
|
META_Object(osglogicopApp,TechniqueEventHandler);
|
|
|
|
virtual bool handle(const osgGA::GUIEventAdapter& ea,osgGA::GUIActionAdapter&);
|
|
|
|
virtual void getUsage(osg::ApplicationUsage& usage) const;
|
|
|
|
protected:
|
|
|
|
~TechniqueEventHandler() {}
|
|
|
|
TechniqueEventHandler(const TechniqueEventHandler&,const osg::CopyOp&) {}
|
|
|
|
osg::LogicOp* _logicOp;
|
|
int _ops_index;
|
|
|
|
};
|
|
|
|
bool TechniqueEventHandler::handle(const osgGA::GUIEventAdapter& ea,osgGA::GUIActionAdapter&)
|
|
{
|
|
switch(ea.getEventType())
|
|
{
|
|
case(osgGA::GUIEventAdapter::KEYDOWN):
|
|
{
|
|
if (ea.getKey()==osgGA::GUIEventAdapter::KEY_Right ||
|
|
ea.getKey()==osgGA::GUIEventAdapter::KEY_KP_Right)
|
|
{
|
|
_ops_index++;
|
|
if (_ops_index>=_ops_nb) _ops_index=0;
|
|
_logicOp->setOpcode(_operations[_ops_index]);
|
|
std::cout<<"Operation name = "<<_ops_name[_ops_index]<<std::endl;
|
|
return true;
|
|
}
|
|
else if (ea.getKey()==osgGA::GUIEventAdapter::KEY_Left ||
|
|
ea.getKey()==osgGA::GUIEventAdapter::KEY_KP_Left)
|
|
{
|
|
_ops_index--;
|
|
if (_ops_index<0) _ops_index=_ops_nb-1;
|
|
_logicOp->setOpcode(_operations[_ops_index]);
|
|
std::cout<<"Operation name = "<<_ops_name[_ops_index]<<std::endl;
|
|
return true;
|
|
}
|
|
return false;
|
|
}
|
|
|
|
default:
|
|
return false;
|
|
}
|
|
}
|
|
|
|
void TechniqueEventHandler::getUsage(osg::ApplicationUsage& usage) const
|
|
{
|
|
usage.addKeyboardMouseBinding("- or Left Arrow","Advance to next opcode");
|
|
usage.addKeyboardMouseBinding("+ or Right Array","Move to previous opcode");
|
|
}
|
|
|
|
|
|
|
|
|
|
int main( int argc, char **argv )
|
|
{
|
|
// use an ArgumentParser object to manage the program arguments.
|
|
osg::ArgumentParser arguments(&argc,argv);
|
|
|
|
// load the nodes from the commandline arguments.
|
|
osg::ref_ptr<osg::Node> loadedModel = osgDB::readRefNodeFiles(arguments);
|
|
|
|
// if not loaded assume no arguments passed in, try use default mode instead.
|
|
if (!loadedModel) loadedModel = osgDB::readRefNodeFile("glider.osgt");
|
|
|
|
if (!loadedModel)
|
|
{
|
|
osg::notify(osg::NOTICE)<<"Please specify model filename on the command line."<<std::endl;
|
|
return 1;
|
|
}
|
|
|
|
osg::ref_ptr<osg::Group> root = new osg::Group;
|
|
root->addChild(loadedModel);
|
|
|
|
osg::ref_ptr<osg::StateSet> stateset = new osg::StateSet;
|
|
osg::ref_ptr<osg::LogicOp> logicOp = new osg::LogicOp(osg::LogicOp::OR_INVERTED);
|
|
|
|
stateset->setAttributeAndModes(logicOp,osg::StateAttribute::OVERRIDE|osg::StateAttribute::ON);
|
|
|
|
//tell to sort the mesh before displaying it
|
|
stateset->setRenderingHint(osg::StateSet::TRANSPARENT_BIN);
|
|
|
|
|
|
loadedModel->setStateSet(stateset);
|
|
|
|
// construct the viewer.
|
|
osgViewer::Viewer viewer;
|
|
|
|
viewer.addEventHandler(new TechniqueEventHandler(logicOp.get()));
|
|
|
|
// run optimization over the scene graph
|
|
osgUtil::Optimizer optimzer;
|
|
optimzer.optimize(root);
|
|
|
|
// add a viewport to the viewer and attach the scene graph.
|
|
viewer.setSceneData( root );
|
|
|
|
return viewer.run();
|
|
}
|