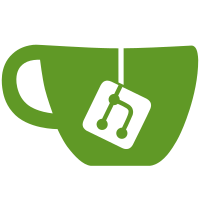
In my example case, there are 2 threads - one is a worker thread created by OpenThreads::Thread. The other thread is the main thread i.e. the thread that is intrinsically created when you execute the application. The crucial problem is that for the main thread, OpenThreads::Thread::CurrentThread() will return null. I'll demonstrate this by breaking ReentrantMutex::lock() into sub-statements: 1.) if (_threadHoldingMutex==OpenThreads::Thread::CurrentThread()) 2.) if (_lockCount>0){ 3.) OpenThreads::ScopedLock<OpenThreads::Mutex> lock(_lockCountMutex); ++_lockCount; return 0; 4.) int result = Mutex::lock(); if (result==0) { OpenThreads::ScopedLock<OpenThreads::Mutex> lock(_lockCountMutex); 5.) _threadHoldingMutex = OpenThreads::Thread::CurrentThread(); _lockCount = 1; return result; An error will occur in the following case: 1) The worker thread calls lock(), it gets to the start of statement 5. 2) The main thread calls lock(). Statement 1 is evaluated as true as _threadHoldingMutex is null, and OpenThreads::Thread::CurrentThread() returns null. 3) The worker thread executes statement 5. 4) The main thread executes statement 2 and evaluates it as true, because the worker thread has set _lockCount to 1. The main thread executes statement 3, and now can access the mutexed-data at the same time as the worker thread! The simple solution to this is to always protect access to _lockCount and _threadHoldingMutex using _lockCountMutex. I have done this in the file I am submitting."
126 lines
3.5 KiB
C++
126 lines
3.5 KiB
C++
/* -*-c++-*- OpenThreads - Copyright (C) 1998-2007 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef _OPENTHREADS_REENTRANTMUTEX_
|
|
#define _OPENTHREADS_REENTRANTMUTEX_
|
|
|
|
#include <OpenThreads/Thread>
|
|
#include <OpenThreads/Mutex>
|
|
#include <OpenThreads/ScopedLock>
|
|
|
|
namespace OpenThreads {
|
|
|
|
class ReentrantMutex : public OpenThreads::Mutex
|
|
{
|
|
public:
|
|
|
|
ReentrantMutex():
|
|
_threadHoldingMutex(0),
|
|
_lockCount(0) {}
|
|
|
|
virtual ~ReentrantMutex() {}
|
|
|
|
virtual int lock()
|
|
{
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> lock(_lockCountMutex);
|
|
if (_threadHoldingMutex==OpenThreads::Thread::CurrentThread() && _lockCount>0)
|
|
{
|
|
++_lockCount;
|
|
return 0;
|
|
}
|
|
}
|
|
|
|
int result = Mutex::lock();
|
|
if (result==0)
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> lock(_lockCountMutex);
|
|
|
|
_threadHoldingMutex = OpenThreads::Thread::CurrentThread();
|
|
_lockCount = 1;
|
|
}
|
|
return result;
|
|
}
|
|
|
|
|
|
virtual int unlock()
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> lock(_lockCountMutex);
|
|
#if 0
|
|
if (_threadHoldingMutex==OpenThreads::Thread::CurrentThread() && _lockCount>0)
|
|
{
|
|
--_lockCount;
|
|
if (_lockCount<=0)
|
|
{
|
|
_threadHoldingMutex = 0;
|
|
return Mutex::unlock();
|
|
}
|
|
}
|
|
else
|
|
{
|
|
osg::notify(osg::NOTICE)<<"Error: ReentrantMutex::unlock() - unlocking from the wrong thread."<<std::endl;
|
|
}
|
|
#else
|
|
if (_lockCount>0)
|
|
{
|
|
--_lockCount;
|
|
if (_lockCount<=0)
|
|
{
|
|
_threadHoldingMutex = 0;
|
|
return Mutex::unlock();
|
|
}
|
|
}
|
|
#endif
|
|
return 0;
|
|
}
|
|
|
|
|
|
virtual int trylock()
|
|
{
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> lock(_lockCountMutex);
|
|
if (_threadHoldingMutex==OpenThreads::Thread::CurrentThread() && _lockCount>0)
|
|
{
|
|
++_lockCount;
|
|
return 0;
|
|
}
|
|
}
|
|
|
|
int result = Mutex::trylock();
|
|
if (result==0)
|
|
{
|
|
OpenThreads::ScopedLock<OpenThreads::Mutex> lock(_lockCountMutex);
|
|
|
|
_threadHoldingMutex = OpenThreads::Thread::CurrentThread();
|
|
_lockCount = 1;
|
|
}
|
|
return result;
|
|
}
|
|
|
|
private:
|
|
|
|
ReentrantMutex(const ReentrantMutex&):OpenThreads::Mutex() {}
|
|
|
|
ReentrantMutex& operator =(const ReentrantMutex&) { return *(this); }
|
|
|
|
OpenThreads::Thread* _threadHoldingMutex;
|
|
|
|
OpenThreads::Mutex _lockCountMutex;
|
|
unsigned int _lockCount;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|