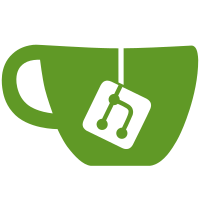
Normally the automatic setup is useful, but in the case of the .osg support this automatic update was forcing premature loading of imagery that wasn't necessarily, and can lead to reports of looking for files that arn't present.
57 lines
1.8 KiB
C++
57 lines
1.8 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2005 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGPARTICLE_EXPLOSIONEFFECT
|
|
#define OSGPARTICLE_EXPLOSIONEFFECT
|
|
|
|
#include <osgParticle/ParticleEffect>
|
|
#include <osgParticle/ModularEmitter>
|
|
#include <osgParticle/FluidProgram>
|
|
|
|
namespace osgParticle
|
|
{
|
|
|
|
class OSGPARTICLE_EXPORT ExplosionEffect : public ParticleEffect
|
|
{
|
|
public:
|
|
|
|
ExplosionEffect(bool automaticSetup=true);
|
|
|
|
ExplosionEffect(const osg::Vec3& position, float scale=1.0f, float intensity=1.0f);
|
|
|
|
ExplosionEffect(const ExplosionEffect& copy, const osg::CopyOp& copyop = osg::CopyOp::SHALLOW_COPY);
|
|
|
|
META_Node(osgParticle,ExplosionEffect);
|
|
|
|
virtual void setDefaults();
|
|
|
|
virtual void setUpEmitterAndProgram();
|
|
|
|
virtual Emitter* getEmitter() { return _emitter.get(); }
|
|
virtual const Emitter* getEmitter() const { return _emitter.get(); }
|
|
|
|
virtual Program* getProgram() { return _program.get(); }
|
|
virtual const Program* getProgram() const { return _program.get(); }
|
|
|
|
protected:
|
|
|
|
virtual ~ExplosionEffect() {}
|
|
|
|
osg::ref_ptr<ModularEmitter> _emitter;
|
|
osg::ref_ptr<FluidProgram> _program;
|
|
|
|
};
|
|
}
|
|
|
|
#endif
|