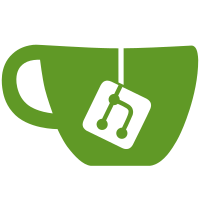
"By repurpose, I'm creating a new plugin that uses much of the .osg fileformat, but with some changes. Specifically, I'm creating a ".osgfs" plugin, which represents the scenegraph hierarchy as a filesystem of nested subdirectories and individual files for each node, rather than nested braces with everything in a single monolithic file. I intend to incorporate file alteration monitor events to watch the filesystem for modifications and automatically reload. The problem I'm running into is osgDB is too tightly coupled to the .osg format. osgDB::Output::writeObject() contains literal .osg format-specific strings like "{" to represent the Object hierarchy at too low a semantic level. I propose using virtual methods; my plugin can then derive from osgDB::Output and represent Object hiearchy differently. "
119 lines
3.8 KiB
C++
119 lines
3.8 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2006 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGDB_OUTPUT
|
|
#define OSGDB_OUTPUT 1
|
|
|
|
#include <osg/Object>
|
|
|
|
#include <osgDB/ReaderWriter>
|
|
|
|
#include <string>
|
|
#include <map>
|
|
#include <fstream>
|
|
|
|
namespace osgDB {
|
|
|
|
/** ofstream wrapper class for adding support for indenting.
|
|
Used in output of .osg ASCII files to improve their readability.*/
|
|
class OSGDB_EXPORT Output : public std::ofstream
|
|
{
|
|
public:
|
|
|
|
Output();
|
|
Output(const char* name);
|
|
|
|
virtual ~Output();
|
|
|
|
|
|
void setOptions(const ReaderWriter::Options* options) { _options = options; }
|
|
const ReaderWriter::Options* getOptions() const { return _options.get(); }
|
|
|
|
void open(const char *name);
|
|
|
|
// comment out temporarily to avoid compilation problems, RO Jan 2002.
|
|
// void open(const char *name,int mode);
|
|
|
|
Output& indent();
|
|
|
|
/** wrap a string with "" quotes and use \" for any internal quotes.*/
|
|
std::string wrapString(const std::string& str);
|
|
|
|
inline void setIndentStep(int step) { _indentStep = step; }
|
|
inline int getIndentStep() const { return _indentStep; }
|
|
|
|
inline void setIndent(int indent) { _indent = indent; }
|
|
inline int getIndent() const { return _indent; }
|
|
|
|
inline void setNumIndicesPerLine(int num) { _numIndicesPerLine = num; }
|
|
inline int getNumIndicesPerLine() const { return _numIndicesPerLine; }
|
|
|
|
void moveIn();
|
|
void moveOut();
|
|
|
|
virtual bool writeObject(const osg::Object& obj);
|
|
virtual void writeBeginObject(const std::string& name);
|
|
virtual void writeEndObject();
|
|
virtual void writeUseID(const std::string& id);
|
|
virtual void writeUniqueID(const std::string& id);
|
|
|
|
bool getUniqueIDForObject(const osg::Object* obj,std::string& uniqueID);
|
|
bool createUniqueIDForObject(const osg::Object* obj,std::string& uniqueID);
|
|
bool registerUniqueIDForObject(const osg::Object* obj,std::string& uniqueID);
|
|
|
|
enum PathNameHint
|
|
{
|
|
AS_IS,
|
|
FULL_PATH,
|
|
RELATIVE_PATH,
|
|
FILENAME_ONLY
|
|
};
|
|
|
|
inline void setPathNameHint(const PathNameHint pnh) { _pathNameHint = pnh; }
|
|
inline PathNameHint getPathNameHint() const { return _pathNameHint; }
|
|
|
|
virtual std::string getFileNameForOutput(const std::string& filename) const;
|
|
const std::string& getFileName() const { return _filename; }
|
|
|
|
void setOutputTextureFiles(bool flag) { _outputTextureFiles = flag; }
|
|
bool getOutputTextureFiles() const { return _outputTextureFiles; }
|
|
|
|
virtual std::string getTextureFileNameForOutput();
|
|
|
|
protected:
|
|
|
|
|
|
virtual void init();
|
|
|
|
osg::ref_ptr<const ReaderWriter::Options> _options;
|
|
|
|
int _indent;
|
|
int _indentStep;
|
|
|
|
int _numIndicesPerLine;
|
|
|
|
typedef std::map<const osg::Object*,std::string> UniqueIDToLabelMapping;
|
|
UniqueIDToLabelMapping _objectToUniqueIDMap;
|
|
|
|
std::string _filename;
|
|
|
|
PathNameHint _pathNameHint;
|
|
bool _outputTextureFiles;
|
|
unsigned int _textureFileNameNumber;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif // __SG_OUTPUT_H
|