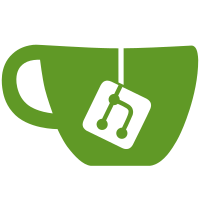
memory manager published at flipcode.com. This can be turned on with the OSG_USE_MEMORY_MANGER option which then uses custom global new and delete operators as well as provide osgNew and osgDelete macro's which add ability to log line and file from which calls are made. Updated osg,osgUtil,osgDB,osgText and osgPlugins/osg to use osgNew/osgDelete, and fixed memory leaks highlighted by the new memory manager.
243 lines
9.6 KiB
Plaintext
243 lines
9.6 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSG_NODE
|
|
#define OSG_NODE 1
|
|
|
|
#include <osg/Object>
|
|
#include <osg/StateSet>
|
|
#include <osg/BoundingSphere>
|
|
#include <osg/NodeCallback>
|
|
#include <osg/ref_ptr>
|
|
|
|
#include <string>
|
|
#include <vector>
|
|
|
|
namespace osg {
|
|
|
|
class NodeVisitor;
|
|
class Group;
|
|
|
|
/** META_Node macro define the standard clone, isSameKindAs, className
|
|
* and accept methods. Use when subclassing from Node to make it
|
|
* more convinient to define the required pure virtual methods.*/
|
|
#define META_Node(name) \
|
|
virtual osg::Object* cloneType() const { return osgNew name (); } \
|
|
virtual osg::Object* clone(const osg::CopyOp& copyop) const { return osgNew name (*this,copyop); } \
|
|
virtual bool isSameKindAs(const osg::Object* obj) const { return dynamic_cast<const name *>(obj)!=NULL; } \
|
|
virtual const char* className() const { return #name; } \
|
|
virtual void accept(osg::NodeVisitor& nv) { if (nv.validNodeMask(*this)) { nv.pushOntoNodePath(this); nv.apply(*this); nv.popFromNodePath(); } } \
|
|
|
|
|
|
/** Base class for all internal nodes in the scene graph.
|
|
Provides interface for most common node operations (Composite Pattern).
|
|
*/
|
|
class SG_EXPORT Node : public Object
|
|
{
|
|
public:
|
|
|
|
/** Construct a node.
|
|
Initialize the parent list to empty, node name to "" and
|
|
bounding sphere dirty flag to true.*/
|
|
Node();
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
Node(const Node&,const CopyOp& copyop=CopyOp::SHALLOW_COPY);
|
|
|
|
/** clone the an object of the same type as the node.*/
|
|
virtual Object* cloneType() const { return osgNew Node(); }
|
|
|
|
/** return a clone of a node, with Object* return type.*/
|
|
virtual Object* clone(const CopyOp& copyop) const { return osgNew Node(*this,copyop); }
|
|
|
|
/** return true if this and obj are of the same kind of object.*/
|
|
virtual bool isSameKindAs(const Object* obj) const { return dynamic_cast<const Node*>(obj)!=NULL; }
|
|
|
|
/** return the name of the node's class type.*/
|
|
virtual const char* className() const { return "Node"; }
|
|
|
|
/** Visitor Pattern : calls the apply method of a NodeVisitor with this node's type.*/
|
|
virtual void accept(NodeVisitor& nv);
|
|
/** Traverse upwards : calls parents' accept method with NodeVisitor.*/
|
|
virtual void ascend(NodeVisitor& nv);
|
|
/** Traverse downwards : calls children's accept method with NodeVisitor.*/
|
|
virtual void traverse(NodeVisitor& /*nv*/) {}
|
|
|
|
|
|
/** Set the name of node using C++ style string.*/
|
|
inline void setName( const std::string& name ) { _name = name; }
|
|
/** Set the name of node using a C style string.*/
|
|
inline void setName( const char* name ) { _name = name; }
|
|
/** Get the name of node.*/
|
|
inline const std::string& getName() const { return _name; }
|
|
|
|
|
|
/** A vector of osg::Group pointers which is used to store the parent(s) of node.*/
|
|
typedef std::vector<Group*> ParentList;
|
|
|
|
/** Get the parent list of node. */
|
|
inline const ParentList& getParents() const { return _parents; }
|
|
|
|
/** Get the a copy of parent list of node. A copy is returned to
|
|
* prevent modification of the parent list.*/
|
|
inline ParentList getParents() { return _parents; }
|
|
|
|
inline Group* getParent(const int i) { return _parents[i]; }
|
|
/**
|
|
* Get a single const parent of node.
|
|
* @param i index of the parent to get.
|
|
* @return the parent i.
|
|
*/
|
|
inline const Group* getParent(const int i) const { return _parents[i]; }
|
|
|
|
/**
|
|
* Get the number of parents of node.
|
|
* @return the number of parents of this node.
|
|
*/
|
|
inline const int getNumParents() const { return _parents.size(); }
|
|
|
|
|
|
/** Set app node callback, called during app traversal. */
|
|
void setAppCallback(NodeCallback* nc);
|
|
|
|
/** Get app node callback, called during app traversal. */
|
|
inline NodeCallback* getAppCallback() { return _appCallback.get(); }
|
|
|
|
/** Get const app node callback, called during app traversal. */
|
|
inline const NodeCallback* getAppCallback() const { return _appCallback.get(); }
|
|
|
|
/** Get the number of Children of this node which require App traversal,
|
|
* since they have an AppCallback attached to them or their children.*/
|
|
inline const int getNumChildrenRequiringAppTraversal() const { return _numChildrenRequiringAppTraversal; }
|
|
|
|
|
|
/** Set the view frustum/small feature culling of this node to be active or inactive.
|
|
* The default value to true for _cullingActive. Used a guide
|
|
* to the cull traversal.*/
|
|
void setCullingActive(const bool active);
|
|
|
|
/** Get the view frustum/small feature _cullingActive flag. Used a guide
|
|
* to the cull traversal.*/
|
|
inline const bool getCullingActive() const { return _cullingActive; }
|
|
|
|
/** Get the number of Children of this node which have culling disabled.*/
|
|
inline const int getNumChildrenWithCullingDisabled() const { return _numChildrenWithCullingDisabled; }
|
|
|
|
|
|
/**
|
|
* Set user data, data must be subclased from Referenced to allow
|
|
* automatic memory handling. If you own data isn't directly
|
|
* subclassed from Referenced then create and adapter object
|
|
* which points to your own objects and handles the memory addressing.
|
|
*/
|
|
inline void setUserData(osg::Referenced* obj) { _userData = obj; }
|
|
/** Get user data.*/
|
|
inline Referenced* getUserData() { return _userData.get(); }
|
|
|
|
/** Get const user data.*/
|
|
inline const Referenced* getUserData() const { return _userData.get(); }
|
|
|
|
|
|
|
|
typedef unsigned int NodeMask;
|
|
/** Set the node mask. Note, node mask is will be replaced by TraversalMask.*/
|
|
inline void setNodeMask(const NodeMask nm) { _nodeMask = nm; }
|
|
/** Get the node Mask. Note, node mask is will be replaced by TraversalMask.*/
|
|
inline const NodeMask getNodeMask() const { return _nodeMask; }
|
|
|
|
|
|
|
|
/** A vector of std::string's which are used to describe the object.*/
|
|
typedef std::vector<std::string> DescriptionList;
|
|
|
|
/** Get the description list of the const node.*/
|
|
inline const DescriptionList& getDescriptions() const { return _descriptions; }
|
|
/** Get the description list of the const node.*/
|
|
inline DescriptionList& getDescriptions() { return _descriptions; }
|
|
/** Get a single const description of the const node.*/
|
|
inline const std::string& getDescription(const int i) const { return _descriptions[i]; }
|
|
/** Get a single description of the node.*/
|
|
inline std::string& getDescription(const int i) { return _descriptions[i]; }
|
|
/** Get the number of descriptions of the node.*/
|
|
inline const int getNumDescriptions() const { return _descriptions.size(); }
|
|
/** Add a description string to the node.*/
|
|
void addDescription(const std::string& desc) { _descriptions.push_back(desc); }
|
|
|
|
|
|
/** set the node's StateSet.*/
|
|
inline void setStateSet(osg::StateSet* dstate) { _dstate = dstate; }
|
|
|
|
/** return the node's StateSet.*/
|
|
inline osg::StateSet* getStateSet() { return _dstate.get(); }
|
|
|
|
/** return the node's const StateSet.*/
|
|
inline const osg::StateSet* getStateSet() const { return _dstate.get(); }
|
|
|
|
/** get the bounding sphere of node.
|
|
Using lazy evaluation computes the bounding sphere if it is 'dirty'.*/
|
|
inline const BoundingSphere& getBound() const
|
|
{
|
|
if(!_bsphere_computed) computeBound();
|
|
return _bsphere;
|
|
}
|
|
|
|
|
|
/** Mark this node's bounding sphere dirty.
|
|
Forcing it to be computed on the next call to getBound().*/
|
|
void dirtyBound();
|
|
|
|
|
|
protected:
|
|
|
|
/** Node destructor. Note, is protected so that Nodes cannot
|
|
be deleted other than by being dereferenced and the reference
|
|
count being zero (see osg::Referenced), preventing the deletion
|
|
of nodes which are still in use. This also means that
|
|
Node's cannot be created on stack i.e Node node will not compile,
|
|
forcing all nodes to be created on the heap i.e Node* node
|
|
= new Node().*/
|
|
virtual ~Node();
|
|
|
|
|
|
/** Compute the bounding sphere around Node's geometry or children.
|
|
This method is automatically called by getBound() when the bounding
|
|
sphere has been marked dirty via dirtyBound().*/
|
|
virtual const bool computeBound() const;
|
|
|
|
mutable BoundingSphere _bsphere;
|
|
mutable bool _bsphere_computed;
|
|
|
|
std::string _name;
|
|
|
|
void addParent(osg::Group* node);
|
|
void removeParent(osg::Group* node);
|
|
|
|
ParentList _parents;
|
|
friend class osg::Group;
|
|
|
|
ref_ptr<NodeCallback> _appCallback;
|
|
int _numChildrenRequiringAppTraversal;
|
|
void setNumChildrenRequiringAppTraversal(const int num);
|
|
|
|
bool _cullingActive;
|
|
int _numChildrenWithCullingDisabled;
|
|
void setNumChildrenWithCullingDisabled(const int num);
|
|
|
|
osg::ref_ptr<Referenced> _userData;
|
|
|
|
NodeMask _nodeMask;
|
|
|
|
DescriptionList _descriptions;
|
|
|
|
ref_ptr<StateSet> _dstate;
|
|
|
|
};
|
|
|
|
/** A vector of Nodes pointers which is used to describe the path from a root node to a descendant.*/
|
|
typedef std::vector<Node*> NodePath;
|
|
|
|
}
|
|
|
|
#endif
|