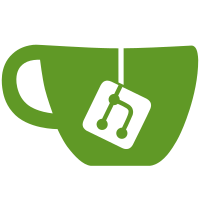
osgWidget::Input: [Functional changes] - Previously, the field would be filled with spaces up to its max length, and typing would just replace the spaces. Also, there was a _textLength variable that kept track of the real length of text in the field, since the osgText::Text's length just reflected the length of spaces+text entered. This was not great, as you could still select the spaces with the mouse and it just feels hacky. So I changed it to only contain the text entered, no spaces, and _textLength was removed since it's now redundant (the osgText::Text's length is used instead). - Fixed the selection size which (visually only) showed one more character selected than what was really selected. - Fixed selection by dragging the mouse, it would sometimes not select the last character of the string. - Cursor will now accurately reflect whether insert mode is activated (block cursor) or we're in normal mode (line cursor) like in most editors. - Implemented Ctrl-X (cut) - Added a new clear() method that allows the field to be emptied correctly. Useful for a command line interface, for example (hint, hint). - Mouse and keyboard event handler methods would always return false, which meant selecting with the mouse would also rotate the trackball, and typing an 's' would turn on stats. [Code cleanup] - Renamed the (local) _selectionMin and _selectionMax variables which are used in a lot of places, as the underscores would lead to think they were members. Either I called them selection{Min|Max} or delete{Min|Max} where it made more sense. - Fixed some indenting which was at 3 spaces (inconsistently), I'm sure I didn't catch all the lines where this was the case though. - Put spaces between variable, operator and value where missing, especially in for()s. Again I only did this where I made changes, there are probably others left. The result is that delete, backspace, Ctrl-X, Ctrl-C, Ctrl-V, and typing behaviour should now be consistent with text editor conventions, whether insert mode is enabled or not. I hope. :-) Note, there's a nasty const_cast in there. Why isn't osgText::Font::getGlyph() declared const? Also, as a note, the current implementation of cut, copy and paste (in addition to being Windows only, yuck) gets and puts the data into an std::string, thus if the osgText::String in the field contains unicode characters I think it won't work correctly. Perhaps someone could implement a proper clipboard class that would be cross-platform and support osgText::String (more precisely other languages like Chinese) correctly? Cut, copy and paste are not critical to what I'm doing so I won't invest the time to do that, but I just thought I'd mention it. "
113 lines
3.3 KiB
C++
113 lines
3.3 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2008 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
// Code by: Jeremy Moles (cubicool) 2007-2008
|
|
|
|
#ifndef OSGWIDGET_INPUT
|
|
#define OSGWIDGET_INPUT
|
|
|
|
#include <osgWidget/Label>
|
|
|
|
namespace osgWidget {
|
|
|
|
// This is a string of values we use to try and determine the best Y
|
|
// descent value (yoffset); you're welcome to use what works best for
|
|
// your font.
|
|
const std::string DESCENT_STRING("qpl");
|
|
|
|
class OSGWIDGET_EXPORT Input: public Label
|
|
{
|
|
public:
|
|
|
|
Input(const std::string& = "", const std::string& = "", unsigned int = 20);
|
|
|
|
virtual void parented (Window*);
|
|
virtual void positioned ();
|
|
|
|
virtual bool focus (const WindowManager*);
|
|
virtual bool unfocus (const WindowManager*);
|
|
virtual bool keyUp (int, int, const WindowManager*);
|
|
virtual bool keyDown (int, int, const WindowManager*);
|
|
virtual bool mouseDrag (double, double, const WindowManager*);
|
|
virtual bool mousePush (double x, double y, const WindowManager*);
|
|
virtual bool mouseRelease (double, double, const WindowManager*);
|
|
|
|
void setCursor (Widget*);
|
|
unsigned int calculateBestYOffset (const std::string& = "qgl");
|
|
void clear();
|
|
|
|
void setXOffset(point_type xo) {
|
|
_xoff = xo;
|
|
}
|
|
|
|
void setYOffset(point_type yo) {
|
|
_yoff = yo;
|
|
}
|
|
|
|
void setXYOffset(point_type xo, point_type yo) {
|
|
_xoff = xo;
|
|
_yoff = yo;
|
|
}
|
|
|
|
osg::Drawable* getCursor() {
|
|
return _cursor.get();
|
|
}
|
|
|
|
const osg::Drawable* getCursor() const {
|
|
return _cursor.get();
|
|
}
|
|
|
|
point_type getXOffset() const {
|
|
return _xoff;
|
|
}
|
|
|
|
point_type getYOffset() const {
|
|
return _yoff;
|
|
}
|
|
|
|
XYCoord getXYOffset() const {
|
|
return XYCoord(_xoff, _yoff);
|
|
}
|
|
|
|
protected:
|
|
virtual void _calculateSize(const XYCoord&);
|
|
|
|
void _calculateCursorOffsets();
|
|
|
|
point_type _xoff;
|
|
point_type _yoff;
|
|
|
|
unsigned int _index;
|
|
unsigned int _size;
|
|
unsigned int _cursorIndex;
|
|
unsigned int _maxSize;
|
|
|
|
std::vector<point_type> _offsets;
|
|
std::vector<unsigned int> _wordsOffsets;
|
|
std::vector<point_type> _widths;
|
|
osg::ref_ptr<Widget> _cursor;
|
|
|
|
bool _insertMode; // Insert was pressed --> true --> typing will overwrite existing text
|
|
|
|
osg::ref_ptr<Widget> _selection;
|
|
unsigned int _selectionStartIndex;
|
|
unsigned int _selectionEndIndex;
|
|
unsigned int _selectionIndex;
|
|
|
|
point_type _mouseClickX;
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|