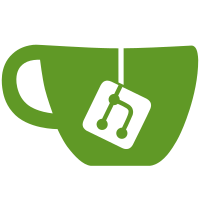
Header update FindFFmpeg.cmake has been changed in order to support the new header include format for FFmpeg. In the 1.0 release, a new file had been added with the name “time.h” in the avutil library. The previous method of adding includes caused conflicts with the ANSI C “time.h” file. Now the include directive will only use the main include folder. All files using the old include format have been updated to reflect the change. Added __STDC_CONSTANT_MACROS define to CMakeLists.txt Since there is no guarantee that FFmpegHeaders.hpp will be included before stdint.h is included, the define has been moved from FFmpegHeaders.hpp to be part of the CMakeLists.txt for the FFmpeg plugin. This will allow the define to work on all compilers regardless of include order. Replaced AVFormatParameters with AVDictionary AVFormatParameters is no longer supported in FFmpeg and has been replaced with a key/value map of strings for each setting. FFmpegParameters and FFmpegDecoder has been updated to reflect this. Replaced av_open_input_file with avformat_open_input FFmpeg now opens files using avformat_open_input. Since the av_open_input_file method is deprecated, the FFmpegDecoder class has been updated to reflect this change. Added custom AVIOContext field to options Since some formats and inputs may not be supported by FFmpeg, I have added a new parameter that allows a user to allocate their own AVIOContext. This class will allow for creating a read, seek, and write callback if they desire. Checking for start_time validity It is possible for some file formats to not provide a start_time to FFmpeg. This would cause stuttering in the video since the clocks class would be incorrect. Removed findVideoStream and findAudioStream The new FFmpeg release already has a function that will find the best audio and video stream. The code has been replaced with this function. Updated error reporting Some functions would not log an error when opening a file or modifying a file failed. New logs have been added as well as a function to convert error numbers to their string descriptions. decode_video has been replaced The old decode_video function would remove extra data that some decoders use in order to properly decode a packet. Now av_codec_decode_video2 has replaced that function. Picture format changed from RGBA32 to RGB24 Since most video will not contain an alpha channel, using a 24 bit texture will use less memory."
164 lines
4.9 KiB
CMake
164 lines
4.9 KiB
CMake
# Locate ffmpeg
|
|
# This module defines
|
|
# FFMPEG_LIBRARIES
|
|
# FFMPEG_FOUND, if false, do not try to link to ffmpeg
|
|
# FFMPEG_INCLUDE_DIR, where to find the headers
|
|
#
|
|
# $FFMPEG_DIR is an environment variable that would
|
|
# correspond to the ./configure --prefix=$FFMPEG_DIR
|
|
#
|
|
# Created by Robert Osfield.
|
|
|
|
|
|
#In ffmpeg code, old version use "#include <header.h>" and newer use "#include <libname/header.h>"
|
|
#In OSG ffmpeg plugin, we used "#include <header.h>" for compatibility with old version of ffmpeg
|
|
#With the new version of FFmpeg, a file named "time.h" was added that breaks compatability with the old version of ffmpeg.
|
|
|
|
#We have to search the path which contain the header.h (usefull for old version)
|
|
#and search the path which contain the libname/header.h (usefull for new version)
|
|
|
|
#Then we need to include ${FFMPEG_libname_INCLUDE_DIRS} (in old version case, use by ffmpeg header and osg plugin code)
|
|
# (in new version case, use by ffmpeg header)
|
|
#and ${FFMPEG_libname_INCLUDE_DIRS/libname} (in new version case, use by osg plugin code)
|
|
|
|
|
|
# Macro to find header and lib directories
|
|
# example: FFMPEG_FIND(AVFORMAT avformat avformat.h)
|
|
MACRO(FFMPEG_FIND varname shortname headername)
|
|
# old version of ffmpeg put header in $prefix/include/[ffmpeg]
|
|
# so try to find header in include directory
|
|
|
|
FIND_PATH(FFMPEG_${varname}_INCLUDE_DIRS lib${shortname}/${headername}
|
|
PATHS
|
|
${FFMPEG_ROOT}/include
|
|
$ENV{FFMPEG_DIR}/include
|
|
~/Library/Frameworks
|
|
/Library/Frameworks
|
|
/usr/local/include
|
|
/usr/include
|
|
/sw/include # Fink
|
|
/opt/local/include # DarwinPorts
|
|
/opt/csw/include # Blastwave
|
|
/opt/include
|
|
/usr/freeware/include
|
|
PATH_SUFFIXES ffmpeg
|
|
DOC "Location of FFMPEG Headers"
|
|
)
|
|
|
|
FIND_PATH(FFMPEG_${varname}_INCLUDE_DIRS ${headername}
|
|
PATHS
|
|
${FFMPEG_ROOT}/include
|
|
$ENV{FFMPEG_DIR}/include
|
|
~/Library/Frameworks
|
|
/Library/Frameworks
|
|
/usr/local/include
|
|
/usr/include
|
|
/sw/include # Fink
|
|
/opt/local/include # DarwinPorts
|
|
/opt/csw/include # Blastwave
|
|
/opt/include
|
|
/usr/freeware/include
|
|
PATH_SUFFIXES ffmpeg
|
|
DOC "Location of FFMPEG Headers"
|
|
)
|
|
|
|
FIND_LIBRARY(FFMPEG_${varname}_LIBRARIES
|
|
NAMES ${shortname}
|
|
PATHS
|
|
${FFMPEG_ROOT}/lib
|
|
$ENV{FFMPEG_DIR}/lib
|
|
~/Library/Frameworks
|
|
/Library/Frameworks
|
|
/usr/local/lib
|
|
/usr/local/lib64
|
|
/usr/lib
|
|
/usr/lib64
|
|
/sw/lib
|
|
/opt/local/lib
|
|
/opt/csw/lib
|
|
/opt/lib
|
|
/usr/freeware/lib64
|
|
DOC "Location of FFMPEG Libraries"
|
|
)
|
|
|
|
IF (FFMPEG_${varname}_LIBRARIES AND FFMPEG_${varname}_INCLUDE_DIRS)
|
|
SET(FFMPEG_${varname}_FOUND 1)
|
|
ENDIF(FFMPEG_${varname}_LIBRARIES AND FFMPEG_${varname}_INCLUDE_DIRS)
|
|
|
|
ENDMACRO(FFMPEG_FIND)
|
|
|
|
SET(FFMPEG_ROOT "$ENV{FFMPEG_DIR}" CACHE PATH "Location of FFMPEG")
|
|
|
|
# find stdint.h
|
|
IF(WIN32)
|
|
|
|
FIND_PATH(FFMPEG_STDINT_INCLUDE_DIR stdint.h
|
|
PATHS
|
|
${FFMPEG_ROOT}/include
|
|
$ENV{FFMPEG_DIR}/include
|
|
~/Library/Frameworks
|
|
/Library/Frameworks
|
|
/usr/local/include
|
|
/usr/include
|
|
/sw/include # Fink
|
|
/opt/local/include # DarwinPorts
|
|
/opt/csw/include # Blastwave
|
|
/opt/include
|
|
/usr/freeware/include
|
|
PATH_SUFFIXES ffmpeg
|
|
DOC "Location of FFMPEG stdint.h Header"
|
|
)
|
|
|
|
IF (FFMPEG_STDINT_INCLUDE_DIR)
|
|
SET(STDINT_OK TRUE)
|
|
ENDIF()
|
|
|
|
ELSE()
|
|
|
|
SET(STDINT_OK TRUE)
|
|
|
|
ENDIF()
|
|
|
|
FFMPEG_FIND(LIBAVFORMAT avformat avformat.h)
|
|
FFMPEG_FIND(LIBAVDEVICE avdevice avdevice.h)
|
|
FFMPEG_FIND(LIBAVCODEC avcodec avcodec.h)
|
|
FFMPEG_FIND(LIBAVUTIL avutil avutil.h)
|
|
FFMPEG_FIND(LIBSWSCALE swscale swscale.h) # not sure about the header to look for here.
|
|
|
|
SET(FFMPEG_FOUND "NO")
|
|
# Note we don't check FFMPEG_LIBSWSCALE_FOUND here, it's optional.
|
|
IF (FFMPEG_LIBAVFORMAT_FOUND AND FFMPEG_LIBAVDEVICE_FOUND AND FFMPEG_LIBAVCODEC_FOUND AND FFMPEG_LIBAVUTIL_FOUND AND STDINT_OK)
|
|
|
|
SET(FFMPEG_FOUND "YES")
|
|
|
|
SET(FFMPEG_INCLUDE_DIRS
|
|
${FFMPEG_LIBAVFORMAT_INCLUDE_DIRS}
|
|
${FFMPEG_LIBAVDEVICE_INCLUDE_DIRS}
|
|
${FFMPEG_LIBAVCODEC_INCLUDE_DIRS}
|
|
${FFMPEG_LIBAVUTIL_INCLUDE_DIRS}
|
|
)
|
|
|
|
# Using the new include style for FFmpeg prevents issues with #include <time.h>
|
|
IF (FFMPEG_STDINT_INCLUDE_DIR)
|
|
SET(FFMPEG_INCLUDE_DIRS
|
|
${FFMPEG_INCLUDE_DIRS}
|
|
${FFMPEG_STDINT_INCLUDE_DIR}
|
|
)
|
|
ENDIF()
|
|
|
|
|
|
SET(FFMPEG_LIBRARY_DIRS ${FFMPEG_LIBAVFORMAT_LIBRARY_DIRS})
|
|
|
|
# Note we don't add FFMPEG_LIBSWSCALE_LIBRARIES here, it will be added if found later.
|
|
SET(FFMPEG_LIBRARIES
|
|
${FFMPEG_LIBAVFORMAT_LIBRARIES}
|
|
${FFMPEG_LIBAVDEVICE_LIBRARIES}
|
|
${FFMPEG_LIBAVCODEC_LIBRARIES}
|
|
${FFMPEG_LIBAVUTIL_LIBRARIES})
|
|
|
|
ELSE ()
|
|
|
|
# MESSAGE(STATUS "Could not find FFMPEG")
|
|
|
|
ENDIF()
|