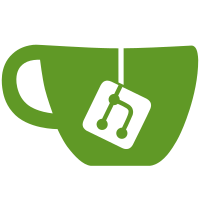
forcing users to use osgDB::readRef*File() methods. The later is preferable as it closes a potential threading bug when using paging databases in conjunction with the osgDB::Registry Object Cache. This threading bug occurs when one thread gets an object from the Cache via an osgDB::read*File() call where only a pointer to the object is passed back, so taking a reference to the object is delayed till it gets reassigned to a ref_ptr<>, but at the same time another thread calls a flush of the Object Cache deleting this object as it's referenceCount is now zero. Using osgDB::readREf*File() makes sure the a ref_ptr<> is passed back and the referenceCount never goes to zero. To ensure the OSG builds when OSG_PROVIDE_READFILE is to OFF the many cases of osgDB::read*File() usage had to be replaced with a ref_ptr<> osgDB::readRef*File() usage. The avoid this change causing lots of other client code to be rewritten to handle the use of ref_ptr<> in place of C pointer I introduced a serious of templte methods in various class to adapt ref_ptr<> to the underly C pointer to be passed to old OSG API's, example of this is found in include/osg/Group: bool addChild(Node* child); // old method which can only be used with a Node* tempalte<class T> bool addChild(const osg::ref_ptr<T>& child) { return addChild(child.get()); } // adapter template method These changes together cover 149 modified files, so it's a large submission. This extent of changes are warrent to make use of the Object Cache and multi-threaded loaded more robust. git-svn-id: http://svn.openscenegraph.org/osg/OpenSceneGraph/trunk@15164 16af8721-9629-0410-8352-f15c8da7e697
137 lines
4.6 KiB
C++
137 lines
4.6 KiB
C++
/* -*-c++-*- OpenSceneGraph - Copyright (C) 1998-2009 Robert Osfield
|
|
*
|
|
* This library is open source and may be redistributed and/or modified under
|
|
* the terms of the OpenSceneGraph Public License (OSGPL) version 0.0 or
|
|
* (at your option) any later version. The full license is in LICENSE file
|
|
* included with this distribution, and on the openscenegraph.org website.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* OpenSceneGraph Public License for more details.
|
|
*/
|
|
|
|
#ifndef OSGVOLUME_LOCATOR
|
|
#define OSGVOLUME_LOCATOR 1
|
|
|
|
#include <osgVolume/Export>
|
|
|
|
#include <osg/Object>
|
|
#include <osg/observer_ptr>
|
|
#include <osg/Matrixd>
|
|
#include <osg/TexGen>
|
|
#include <osg/MatrixTransform>
|
|
|
|
#include <vector>
|
|
|
|
namespace osgVolume {
|
|
|
|
class OSGVOLUME_EXPORT Locator : public osg::Object
|
|
{
|
|
public:
|
|
|
|
Locator() {}
|
|
|
|
Locator(const osg::Matrixd& transform) { setTransform(transform); }
|
|
|
|
/** Copy constructor using CopyOp to manage deep vs shallow copy.*/
|
|
Locator(const Locator& locator,const osg::CopyOp& copyop=osg::CopyOp::SHALLOW_COPY):
|
|
osg::Object(locator, copyop),
|
|
_transform(locator._transform) {}
|
|
|
|
META_Object(osgVolume, Locator);
|
|
|
|
/** Set the transformation from local coordinates to model coordinates.*/
|
|
void setTransform(const osg::Matrixd& transform) { _transform = transform; _inverse.invert(_transform); locatorModified(); }
|
|
|
|
/** Set the transformation from local coordinates to model coordinates.*/
|
|
const osg::Matrixd& getTransform() const { return _transform; }
|
|
|
|
/** Set the extents of the local coords.*/
|
|
void setTransformAsExtents(double minX, double minY, double maxX, double maxY, double minZ, double maxZ);
|
|
|
|
|
|
virtual bool convertLocalToModel(const osg::Vec3d& /*local*/, osg::Vec3d& /*world*/) const;
|
|
|
|
virtual bool convertModelToLocal(const osg::Vec3d& /*world*/, osg::Vec3d& /*local*/) const;
|
|
|
|
static bool convertLocalCoordBetween(const Locator& source, const osg::Vec3d& sourceNDC,
|
|
const Locator& destination, osg::Vec3d& destinationNDC)
|
|
{
|
|
osg::Vec3d model;
|
|
if (!source.convertLocalToModel(sourceNDC, model)) return false;
|
|
if (!destination.convertModelToLocal(model, destinationNDC)) return false;
|
|
return true;
|
|
}
|
|
|
|
bool computeLocalBounds(osg::Vec3d& bottomLeft, osg::Vec3d& topRight) const;
|
|
bool computeLocalBounds(Locator& source, osg::Vec3d& bottomLeft, osg::Vec3d& topRight) const;
|
|
|
|
|
|
/** Callback interface for enabling the monitoring of changes to the Locator.*/
|
|
class LocatorCallback : virtual public osg::Object
|
|
{
|
|
public:
|
|
LocatorCallback() {}
|
|
LocatorCallback(const LocatorCallback& rhs, const osg::CopyOp& copyop=osg::CopyOp::SHALLOW_COPY): osg::Object(rhs,copyop) {}
|
|
META_Object(osgVolume, LocatorCallback);
|
|
|
|
virtual void locatorModified(Locator* /*locator*/) {};
|
|
|
|
protected:
|
|
virtual ~LocatorCallback() {}
|
|
};
|
|
|
|
void addCallback(LocatorCallback* callback);
|
|
template<class T> void addCallback(const osg::ref_ptr<T>& callback) { addCallback(callback.get()); }
|
|
|
|
|
|
void removeCallback(LocatorCallback* callback);
|
|
|
|
typedef std::vector< osg::ref_ptr<LocatorCallback> > LocatorCallbacks;
|
|
LocatorCallbacks& getLocatorCallbacks() { return _locatorCallbacks; }
|
|
const LocatorCallbacks& getLocatorCallbacks() const { return _locatorCallbacks; }
|
|
|
|
protected:
|
|
|
|
void locatorModified();
|
|
osg::Matrixd _transform;
|
|
osg::Matrixd _inverse;
|
|
|
|
LocatorCallbacks _locatorCallbacks;
|
|
};
|
|
|
|
class OSGVOLUME_EXPORT TransformLocatorCallback : public Locator::LocatorCallback
|
|
{
|
|
public:
|
|
|
|
TransformLocatorCallback(osg::MatrixTransform* transform);
|
|
|
|
void locatorModified(Locator* locator);
|
|
|
|
protected:
|
|
|
|
osg::observer_ptr<osg::MatrixTransform> _transform;
|
|
};
|
|
|
|
|
|
class OSGVOLUME_EXPORT TexGenLocatorCallback : public Locator::LocatorCallback
|
|
{
|
|
public:
|
|
|
|
TexGenLocatorCallback(osg::TexGen* texgen, Locator* geometryLocator, Locator* imageLocator);
|
|
|
|
void locatorModified(Locator*);
|
|
|
|
protected:
|
|
|
|
osg::observer_ptr<osg::TexGen> _texgen;
|
|
osg::observer_ptr<osgVolume::Locator> _geometryLocator;
|
|
osg::observer_ptr<osgVolume::Locator> _imageLocator;
|
|
};
|
|
|
|
|
|
}
|
|
|
|
#endif
|