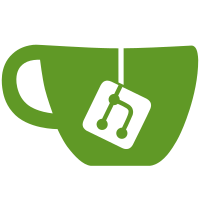
include change the CameraManipulators so they work with double for time instead of float. Also added support for DataType to osg::StateAttribute and StateSet so that they can be set to either STATIC or DYNAMIC, this allows the optimizer to know whether that an attribute can be optimized or not.
116 lines
3.6 KiB
Plaintext
116 lines
3.6 KiB
Plaintext
//C++ header - Open Scene Graph - Copyright (C) 1998-2001 Robert Osfield
|
|
//Distributed under the terms of the GNU Library General Public License (LGPL)
|
|
//as published by the Free Software Foundation.
|
|
|
|
#ifndef OSGGLUT_GLUTEVENTADAPTER
|
|
#define OSGGLUT_GLUTEVENTADAPTER 1
|
|
|
|
#include <osgUtil/GUIEventAdapter>
|
|
|
|
#include <osgGLUT/Export>
|
|
|
|
namespace osgGLUT{
|
|
|
|
/** Class for adapting GLUT events so that they can be used as input to osgUtil::CameraManipulators.*/
|
|
class OSGGLUT_EXPORT GLUTEventAdapter : public osgUtil::GUIEventAdapter
|
|
{
|
|
|
|
public:
|
|
GLUTEventAdapter();
|
|
virtual ~GLUTEventAdapter() {}
|
|
|
|
/** Get the EventType of the GUI event.*/
|
|
virtual EventType getEventType() const { return _eventType; }
|
|
|
|
/** key pressed, return -1 if inappropriate for this event. */
|
|
virtual int getKey() const { return _key; }
|
|
|
|
/** button pressed/released, return -1 if inappropriate for this event.*/
|
|
virtual int getButton() const { return _button; }
|
|
|
|
/** window minimum x. */
|
|
virtual int getXmin() const { return _Xmin; }
|
|
|
|
/** window maximum x. */
|
|
virtual int getXmax() const { return _Xmax; }
|
|
|
|
/** window minimum y. */
|
|
virtual int getYmin() const { return _Ymin; }
|
|
|
|
/** window maximum y. */
|
|
virtual int getYmax() const { return _Ymax; }
|
|
|
|
/** current mouse x position.*/
|
|
virtual int getX() const { return _mx; }
|
|
|
|
/** current mouse y position.*/
|
|
virtual int getY() const { return _my; }
|
|
|
|
/** current mouse button state */
|
|
virtual unsigned int getButtonMask() const { return _buttonMask; }
|
|
|
|
/** time in seconds of event. */
|
|
virtual double time() const { return _time; }
|
|
|
|
|
|
/** static method for setting window dimensions.*/
|
|
static void setWindowSize(int Xmin, int Ymin, int Xmax, int Ymax);
|
|
|
|
/** static method for setting button state.*/
|
|
static void setButtonMask(unsigned int buttonMask);
|
|
|
|
/** method for adapting resize events. */
|
|
void adaptResize(double t, int Xmin, int Ymin, int Xmax, int Ymax);
|
|
|
|
/** method for adapting mouse motion events whilst mouse buttons are pressed.*/
|
|
void adaptMouseMotion(double t, int x, int y);
|
|
|
|
/** method for adapting mouse motion events whilst no mouse button are pressed.*/
|
|
void adaptMousePassiveMotion(double t,int x, int y);
|
|
|
|
/** method for adapting mouse button pressed/released events.*/
|
|
void adaptMouse(double t,int button, int state, int x, int y);
|
|
|
|
/** method for adapting keyboard events.*/
|
|
void adaptKeyboard(double t,unsigned char key, int x, int y );
|
|
|
|
/** method for adapting frame events, i.e. idle/display callback.*/
|
|
void adaptFrame(double t);
|
|
|
|
void copyStaticVariables();
|
|
|
|
protected:
|
|
|
|
EventType _eventType;
|
|
int _key;
|
|
int _button;
|
|
int _Xmin,_Xmax;
|
|
int _Ymin,_Ymax;
|
|
int _mx;
|
|
int _my;
|
|
unsigned int _buttonMask;
|
|
double _time;
|
|
|
|
// used to accumulate the button mask state, it represents
|
|
// the current button mask state, which is modified by the
|
|
// adaptMouse() method which then copies it to value _buttonMask
|
|
// which required the mouse buttons state at the time of the event.
|
|
static unsigned int _s_accumulatedButtonMask;
|
|
|
|
// used to store current button value
|
|
static int _s_button;
|
|
|
|
// used to store window min and max values.
|
|
static int _s_Xmin;
|
|
static int _s_Xmax;
|
|
static int _s_Ymin;
|
|
static int _s_Ymax;
|
|
static int _s_mx;
|
|
static int _s_my;
|
|
|
|
};
|
|
|
|
}
|
|
|
|
#endif
|